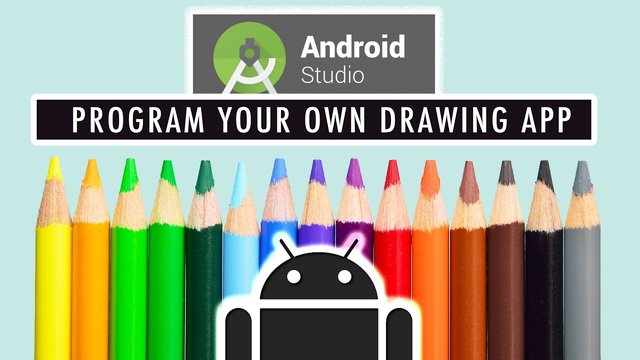
Hello, and welcome to this new Android development course. This is part one on how to make a drawing app for Android.
If you will enjoy reading and contributing to the discussion for this post, will you please join us on the YouTube video above and leave a comment there because I read and respond to most comments on YouTube?
If you find anything helpful in this video or funny, will you please leave a like because you will feel great helping other people find it?
In this video we will set up the basics and continue with our applications. We open or Android studio IDE and click on the start of a new Android studio project. Here we click on an empty activity and then we click on next.
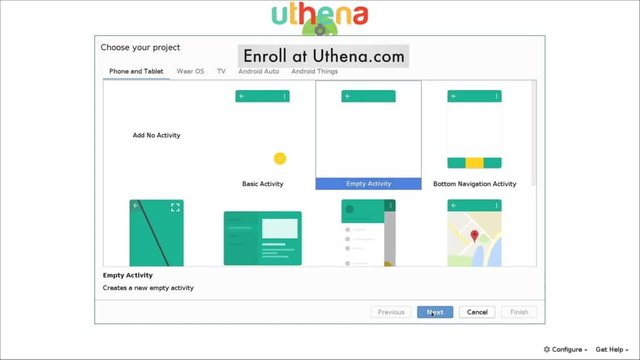
Here in my application we’ll change the name to Drawing App, for example. We’ll set the language to Java and we will set the minimum API level to 17 this time. We will now click on finish and we will wait for the IDE to load our application.
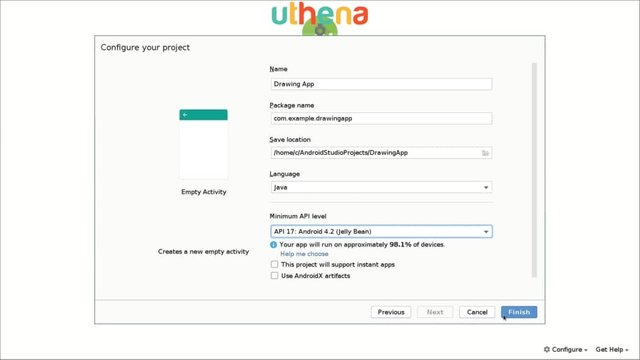
We have set this time to 17 because 17 almost targets the whole Android market share. Right now, we have our Android studio project set up right here and we have to continue to create or draw an application. To do this we will open our manifest file and we will set the activity orientation to portrait. We have set a portrait because our drawing app will be in portrait mode.
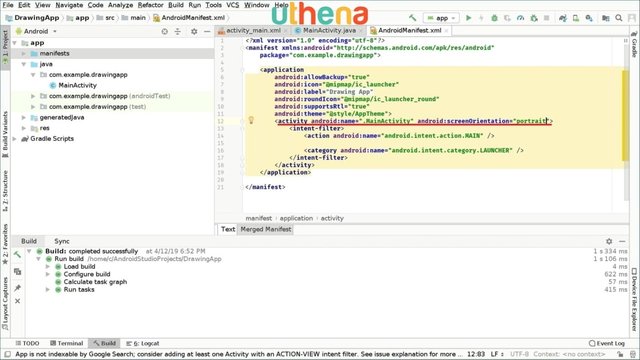
Before running we will change the default layout file with the linear layout. So we go under the res folder, and then under the layout folder, and we right-click on the activity_main.xml file and we click on delete.
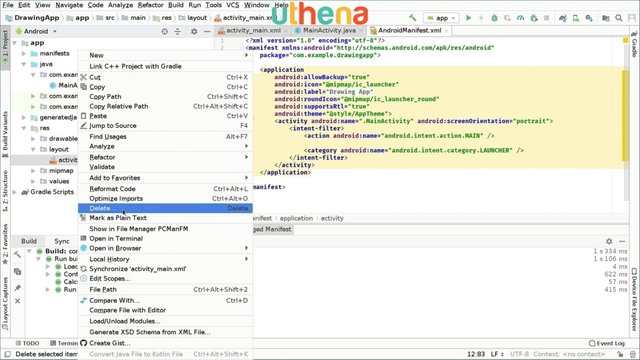
Make sure you have these two options unchecked and then click on OK.
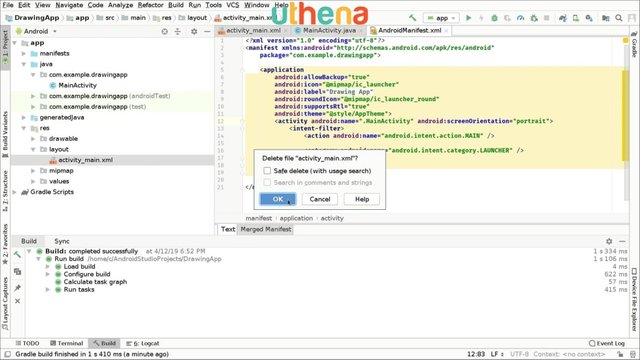
Now that we have deleted we will create a new one. So right click on the new layout resource file.
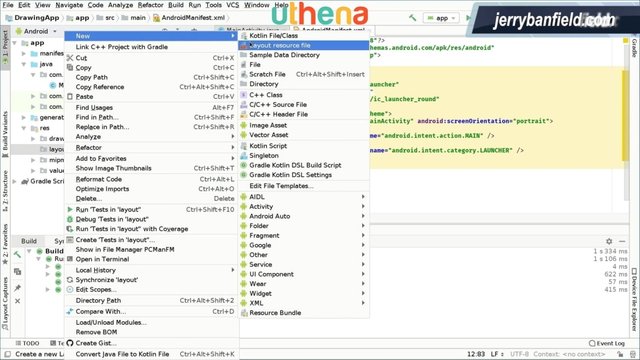
Here we will also say set the name to activity_main as you can see, but the root element will be changed to linear layout as you can see. When we click on OK, the IDE will generate this new code for us.
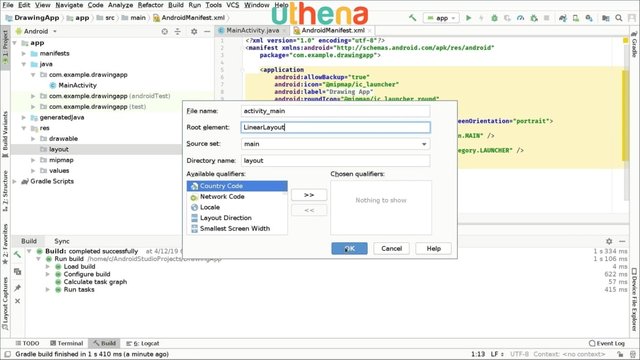
If we switch to text mode we can see that we have the linear layout we requested.
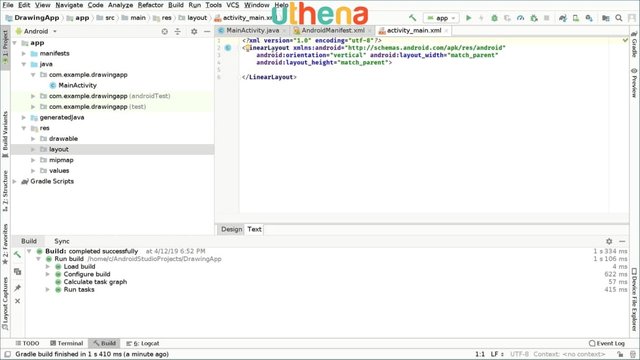
If we run this, I will run this on the emulator and we will see how it’s looking like on the device. Now, I’m compiling and I will run the app on the emulator on the left side of the screen, so you can see. We wait for the app to be compiled and I will switch to the emulator to wait for the app to run. Right here will be the emulator which was set up using Android studio and it’s taking quite some time.
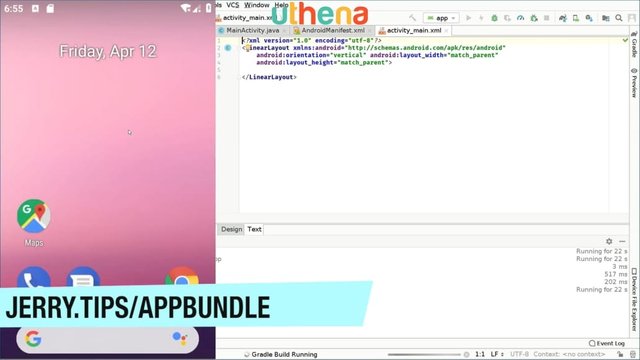
Maybe the first time takes a little bit more time than usual but it’s already installing and launching the app, and here is our drawing application. We have this top bar which shows the time and all the notification icons, and we also have this title bar which is showing the title of our app in this case Drawing App.
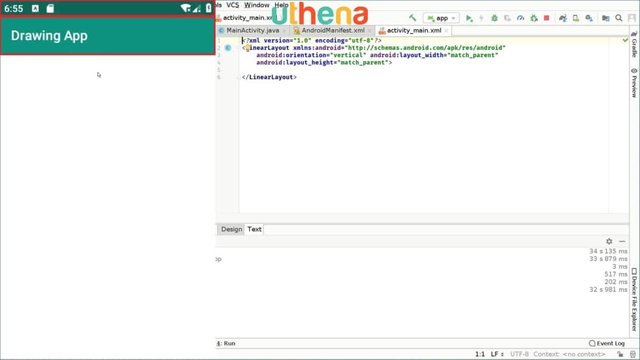
We want to hide these two bars. To hide those bars we go back to the IDE and on the left, under the res, and then values folder will open the styles.xml file and as you can see here we can change the items of our current app theme. To hide those bars we will create a new item. The first being WindowNoTitle which is the title bar, and we’ll set this to true, so we don’t want a title. We will also create a new item that will say windowFullScreen as you can see, and we will also set this to true. Once we’ve done this we will run the app again to check if the changes took effect on the emulator device.
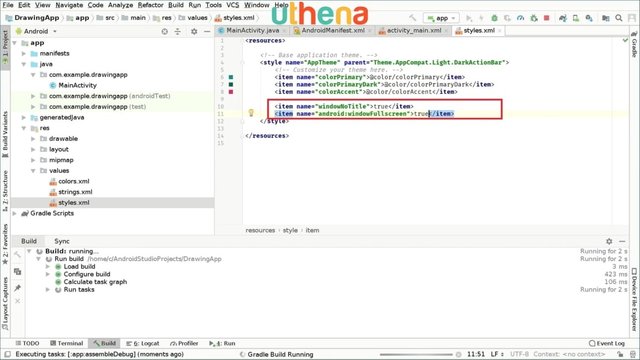
Right now it’s installing and as you can see there’s nothing shown, so both bars are hidden. All we have is a white rectangle which is exactly what we want for now.
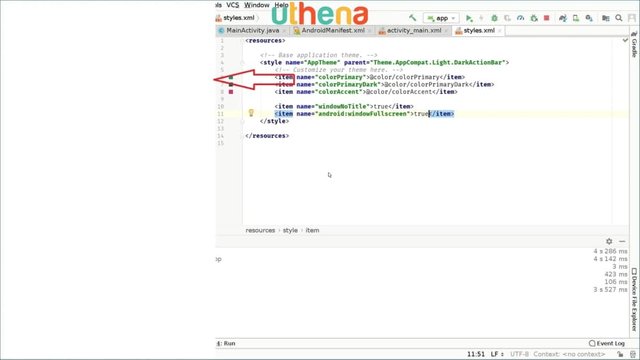
To draw on the application what we have to do is to create a kind of paint view which will use the canvas object to draw into the display. To do that we will create an external class. Our external class will be called paintView actually. So to create an external class we go and right-click under the Java folder, and we will click on the package name. In my case com.example.drawing app. I will right click on that and click on a new Java class.
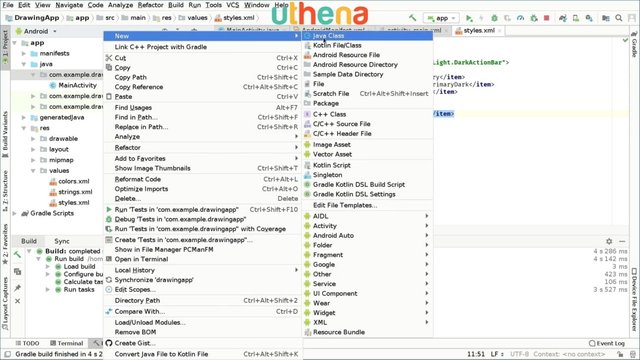
I will set that name to PaintView and then click on OK. Now, the IDE has created this new class for us.
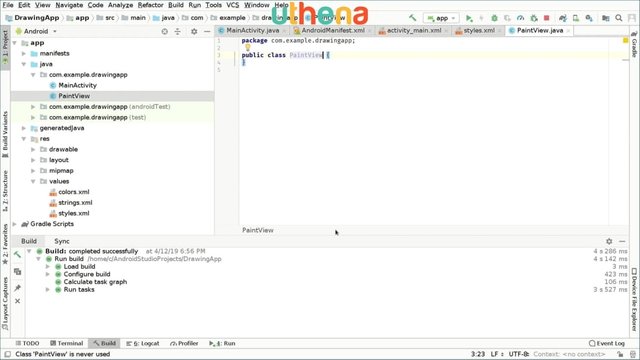
It says public class PaintView and we will continue to improve and modify this class to our drawing app needs.
The first thing we want to do is to extend the view class. To extend the viewing class is pretty simple, we will just type right here and extend the View. As you can see, of course, we have to import the view object as you can see right here, and now we have extended the view.
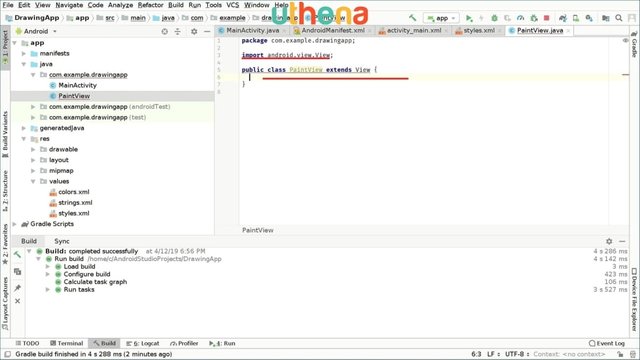
As you can see we have a warning right here which tells us there’s no default constructor available.
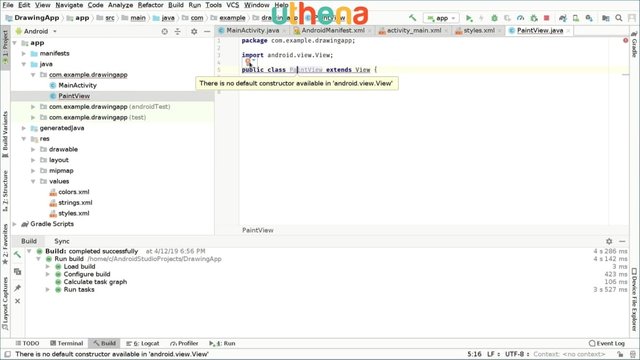
If we click here which will create the constructor matching super. We can do that or we can also do it later manually. But if we go and do this and choose the first, for example, we have this constructor just silence this warning.
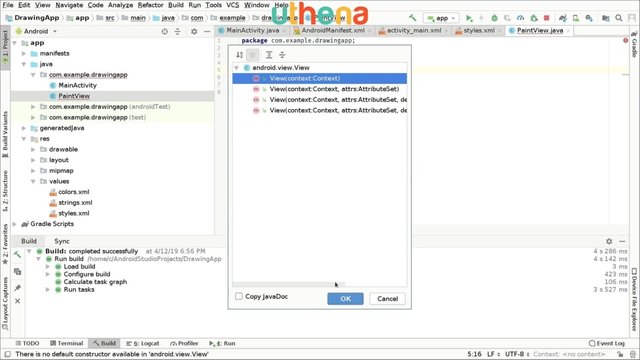
Doesn’t really matter because we will write it anyway later. This is the main method but we will also kind of pass the attributes set if I’m not wrong. We will create a global variable of paint in which will be called mPaint. For that we go and say private Paint. We have to import this. This class will enable us to draw. So private Paint mPaint. We created the paint object.
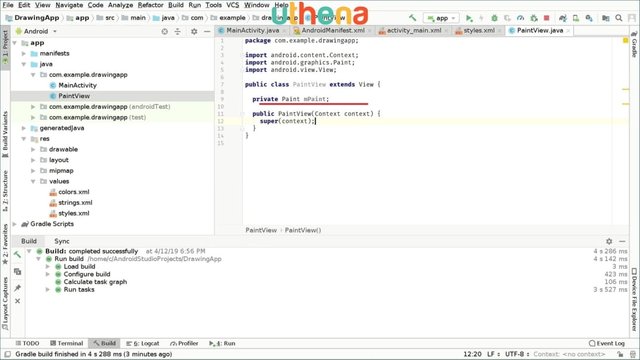
Right here, we will start setting up that object. So we’re going to say mPaint = new Paint and then we will start passing the parameters we want. For example, we can set different styles, strokes, lots of really useful stuff.
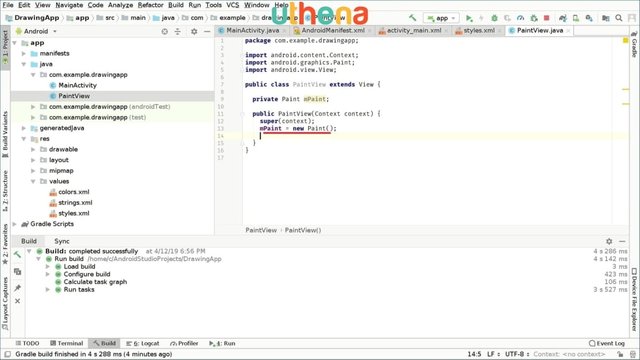
Since we want to draw something, we will do the following so we’ll say mPaint.setAntiAlias, we will set this to true to enable anti-aliasing. Great. We will set the dither to True. To do that we’re going to say mPaint.setDither to True. This is basically a small setting that we have to set in order for the app to draw. It isn't really important to go deep for every one of this so we’ll continue right now. mPaint.setColor and we might set this to black so this is the kind of color we are drawing with. We’re going to say Color.BLACK. You want to set that as default. As simple as that.
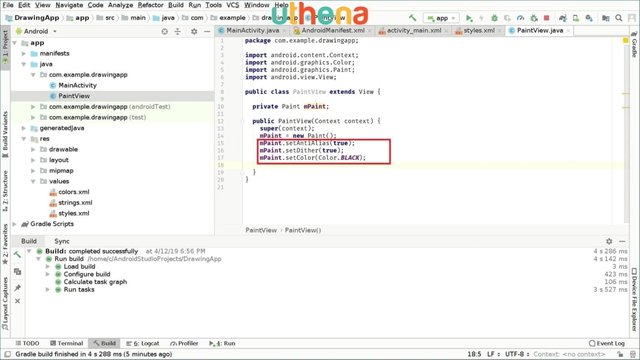
We’re going to set the style of mPaint, so I’m going to say mPaint.setStyle, and as a style we will pass Paint.Style.Stroke. Because we will be drawing. Now, maybe we will set a strong stroke style. So mPaint.setStrokeJoin, and here's what we will do, it will be Paint.Join.ROUND. We want kind of rounded endings as we draw. We also set the stroke cap which is again just aesthetic changes that are not that important. So, setStrokeCap(Paint.cap.ROUND). We’ve done this and now we’re going to talk about the mode. There is an extra mode in which we won’t use that match so we can set it to null. mPaint .setXfermode mode will be set to null right now. The last one will be set to alpha. mPaint.setAlpha we will set that to 0XFF to make it possible to see what we’re doing. That’s it for the constructor method.
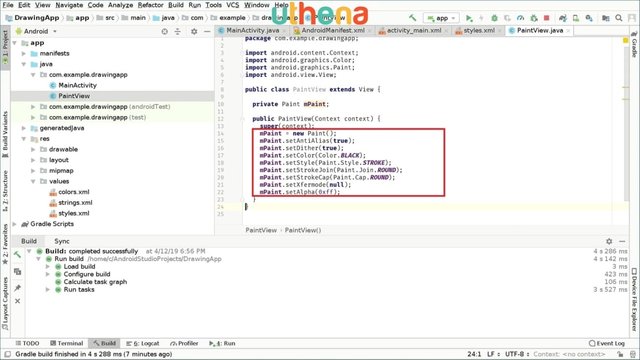
That’s the kind of main method but we also want to create an initialization method in which we will pass the size of the user of the device screen. We have to know which size we will be drawing into. So you’re going to talk about the public void. Of course, we will have to call this from our main activity class. We will call init and we will pass the display metrics we get which are the screen sizes. Display metrics metrics and we will pass this stuff right here.
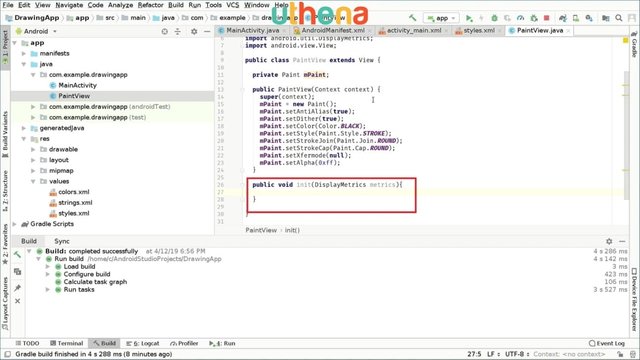
Right now we have our kind of Paint view. We’ll still have to implement some more stuff but it’s extending the view. In order for us to complete this design we will go to our activity_main.xml file right here, and we will get the view from the code. The view that we created in this place right here and specify it as xml. How can we do this? Well, we will basically set this inside the frame layout in order for us to later be flexible for us to change stuff. We created a frame layout. We will set the width to match the parent. Height to 0 DP. We want to create two views with two different weights in order for us to simplify our life. In this case, we want to make the paint view show 90% of the screen. At the bottom we will have a bar that will use the 10% maybe. We can change this later. It’s not that important.
We’re going to set this weight to nine which will represent 90% for us, and inside this frame layout we will specify our paint view which we access as com.example.drawing app.PaintView. The width of this paint view will match the parent and the height will also match parent.
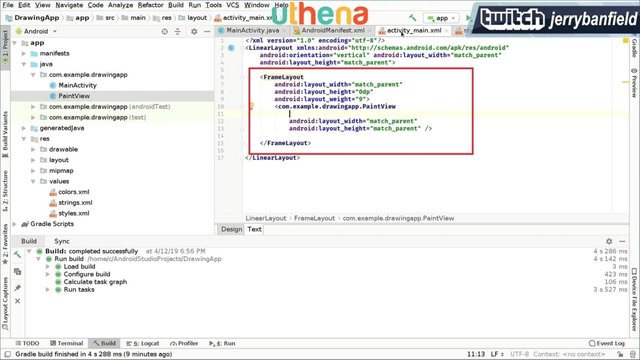
In order for us to access this from the code we have to assign it an ID, so you can call it ID. We may set this to be a paintView. Now we have to create our paint view right here and we will also create the bottom bar I was just talking about. The bottom bar will be a linear layout the width will match parent and the height will be 0 DP and the weight will be 1. This is weight one and the other was weight 9, so this occupies 10% and the other 90%. Just a simple trick to avoid doing lots of code and all of that. We solve everything here.
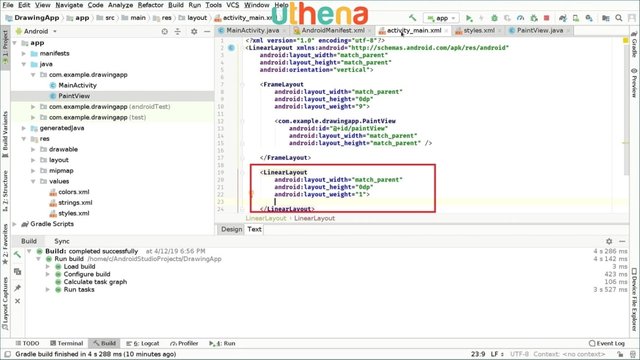
Now we can create some buttons right here. Maybe something that will enable us to change the colors of what we’re drawing with or something like that. Maybe we can clear the current drawing but we will do that later. We’ll just leave it as it is and I will set this background to black. To create a black color for that we go under colors.xml file, and down here we’ll create a new black color. For that, I open and say color name will be black and here I will specify number sign followed by six zeros which is black in hexadecimal.
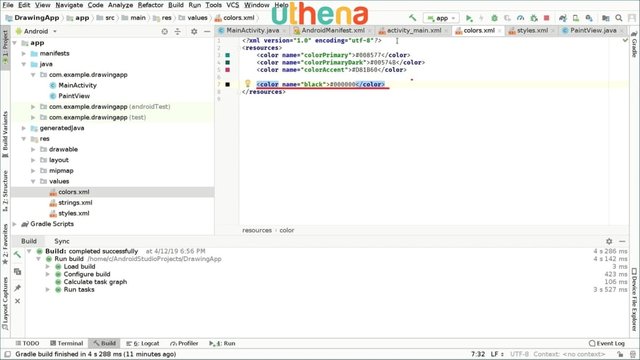
I have a black color. I can now close this file and go to activity_main.xml and this background of this linear layout, I will set this to black.
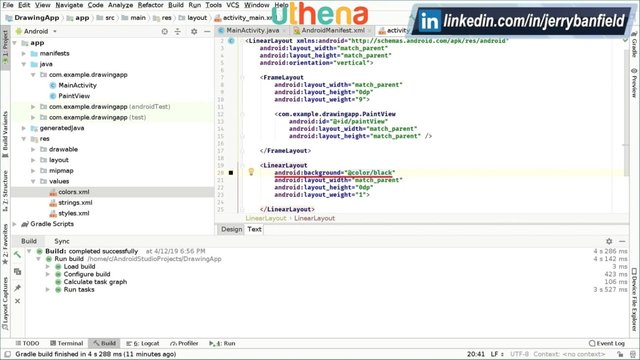
That’s our linear layout and if I run the app now I can have an idea of what I just did right here. I mean the 90-10%. The app is running. Let me run it again. It didn’t run for some reason. The app is running and we have some sort of error maybe because we have not finished coding.
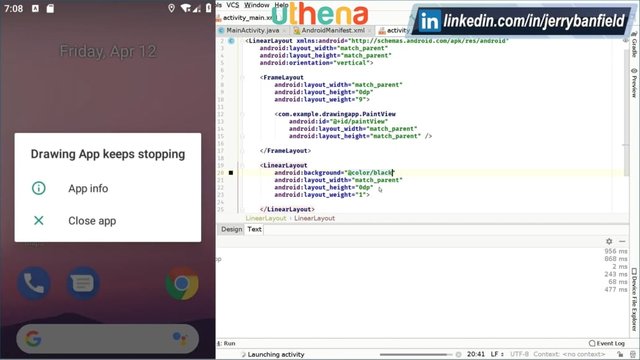
Maybe you have some sort of error right here so let’s check in the logcat to see what’s going wrong with our application. Whenever something goes wrong we can go to logcat as I’m doing and find the actual line of code that’s causing problems. Right here we have some errors in our binary XML file. That’s great because we haven’t finished writing this class and we are already using it right here. If we select this and comment on this or delete it for a moment, and run the app again, we will see if it is solved.
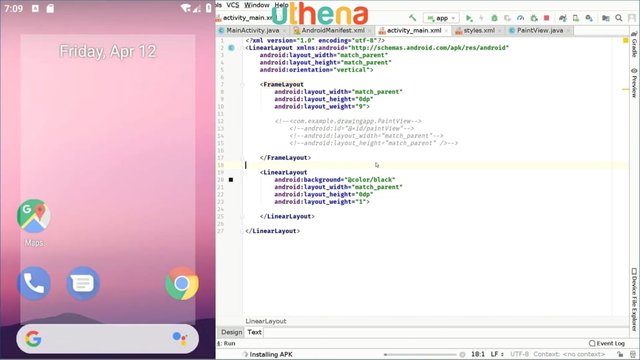
I am installing the app and as you can see it’s solved and as I was telling you this white thing which will be our paint view will occupy 90% percent of the whole screen height, and the remaining 10% remaining will be occupied by the bottom bar. That’s what I wanted to show you as a quick thing right here, and now we can uncomment this again.
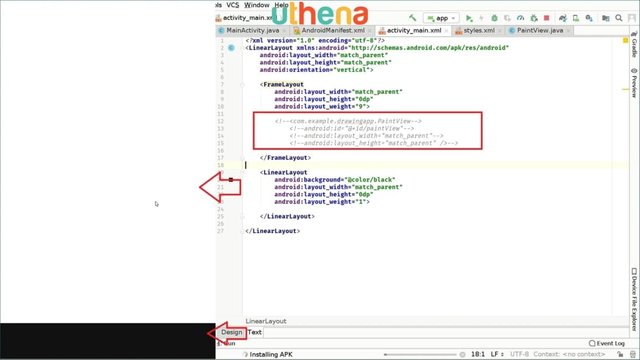
We have to keep in mind that before running the app we have to end right in this class. To write this class, we will continue to be using the init method. We will get the height and the width of the metrics we are passing right here. To do that, we’ve got to say int height will be set to metrics.heightPixels, but this will return the whole height of the device which we don’t really want. We want 90% of the entire height of the device, so we can multiply this with 0.9 that way we can get the 90%. We will cast this to double. As you can see it says method cast it to double. Cast to int actually. From double to int. If we cast it to int then everything works. We also want to get that width. The width doesn’t change so we’re going to say int width. We’ll say, the metrics.widthPixels as you can see right here. Now we have our height and our width which is great and we want to create our bitmap.
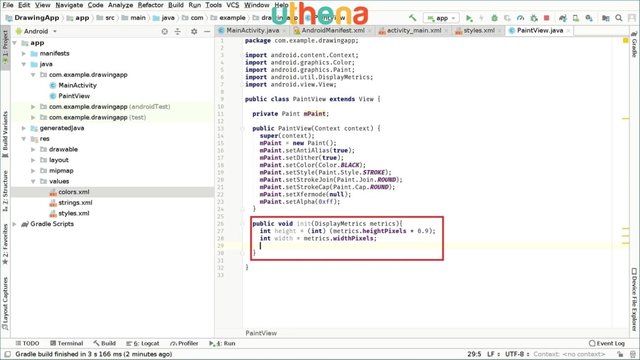
So a bitmap will enable us to store the information of our canvas where we draw our stuff. We will create our bitmap and we will access it and initialize it.
We’re going to say bitmap, I will call it mBitmap, for example, and I will set this object to Bitmap.createBitmap, and we will pass the parameters. First we will pass width, then we’ll pass height, then we will pass the configuration of our bitmap. We want it to be able to display colors. For that, the most useful configuration is Bitmap.config.ARGB_8888. Right now we have set up our bitmap and we also want to set up our canvas. I will call this mCanvas and I will set this up to a new canvas and I will create a new canvas with the bitmap I set up previously. I will pass mBitmap which is great. We have created our canvas with our bitmap.
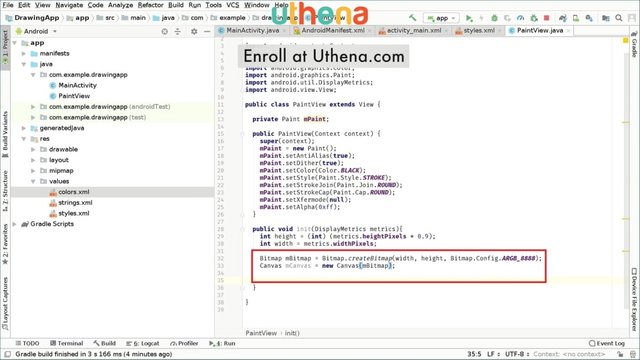
This is basically what we have to initialize in this method. We’ll have to deal with colors and all those sort of things but for now, we will leave it there and we will create another method which will be the onDraw method. To do that, we will override the onDraw method, so override protected void onDraw, and we will get the canvas right here, so canvas.
Right here we will save the canvas in order for us to draw a new color or something like that. You’re going to go and say canvas.save. This enables us to save the state of the canvas.
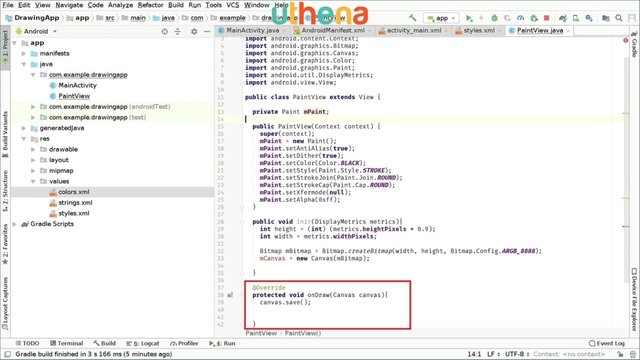
We have to access our previously created canvas which is this canvas right here, so you have to create this as a global variable, okay? Go before the paint view method and type private Canvas mCanvas.
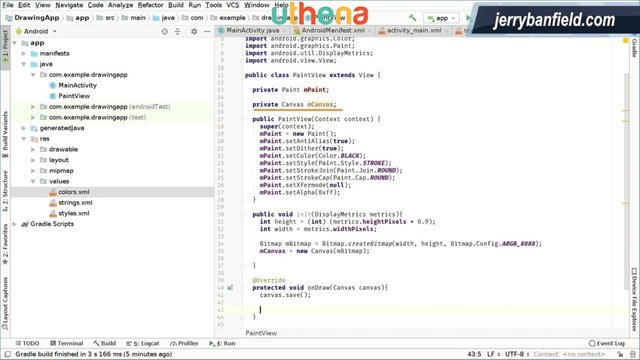
Now, that object can be accessed from the onDraw method. We want to say mCanvas and we will set some kind of the background color. So, drawColor and this background color we will set it to Color.WHITE. The background of our drawing application will be white.
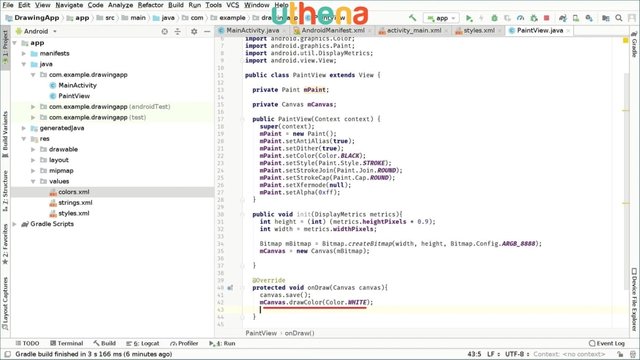
We will define how our finger moves through the screen in order for us to draw on the canvas.
We will have to define that.
To define that we will go to our main_activity.java file and we will define something that we will call our finger path. We’re going to go after the onCreate method, we are going to create a static class or rather say static class FingerPath. We open the element right here. This class will have its own color, the stroke width and the path itself. First we’ll go and say int color. We’ll have int StrokeWidth. Here as Path we will import the graphics one as you can see right here. I’m importing the graphics path.
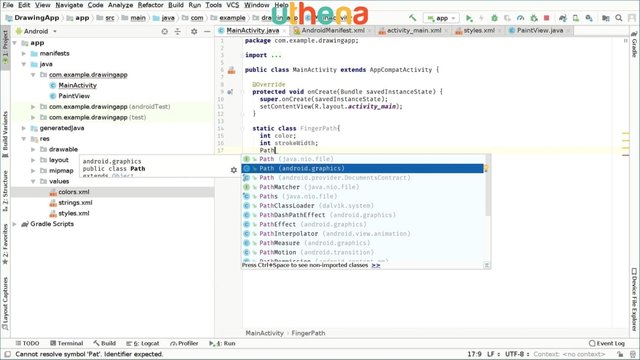
Everything’s great. Now the path we’ll call it just path. We will create a constructor method which will be FingerPath and we will pass color, of course, in order for it to set the color. We’ll pass, of course, the strokeWidth and will also pass the path. Inside this we will just set them, so you can say this color equals color. The same as the other one. So this.strokeWidth equals strokeWidth. So we’re setting new parameters for our finger path. What we do when we touch our fingers.
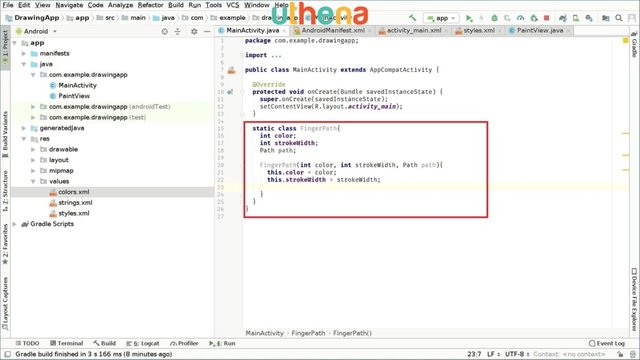
This.path will be set to path and now we have created our finger path which is awesome. So right now we can go back to our paintView.java. Now, we can finish with our onDraw method. Right here what we will do is we will get the finger path we have on our activity main or main activity, and we will set the color and the stroke and all of that. We’re going to speak for MainActivity.FingerPath,we’ll this fp, for example, and we will switch it with paths.
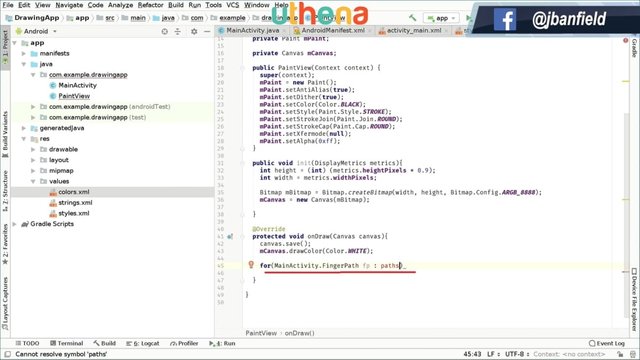
What are those paths? Right here. Those paths will store the actual path of our application, so how can we define those paths? Well, these paths will be created with an array list of finger paths. We go and create it as a global variable. Go ahead and say private array list as you can see and the data type of this arrayList will be MainActivity.Fingerpath and we will call these paths. We will set this up for the new ArrayList.
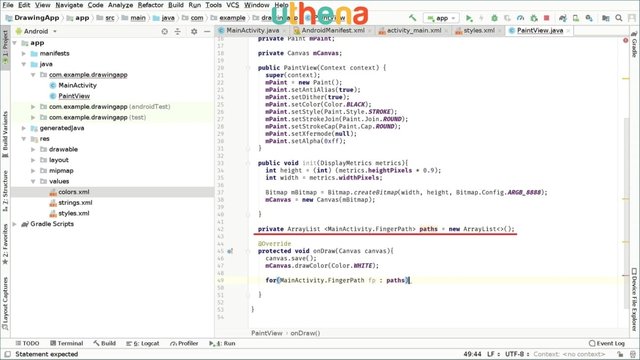
We have our paths global variable right here. So what we have to do now is continue writing the for loop. We open this loop and we’re going to say mPaint.setColor and it will be set to the path color in this case, fp.color. Now we’ll set the stroke width. I’m going to go to mPaint.setStrokeWidth and we are set to fp.strokeWidth. We will also set the mass filter to normal for us to make the drawing mask look how we want. We will set mPaint.setMaskFilter to null. We will draw the path right now. To draw the path you can say mCanvas.drawPath and we will pass, of course, fp.path, and we will also pass mPaint. T his is how we do this for every loop right here but now we have to display these changes to the screen.
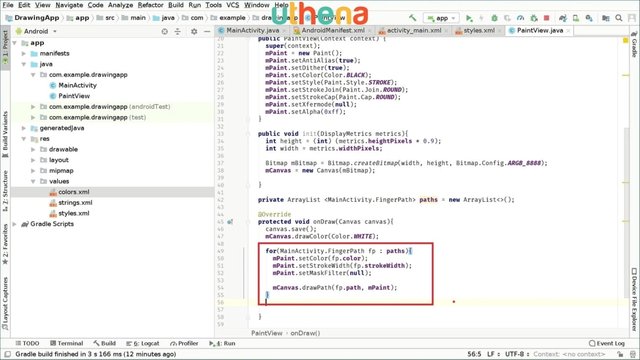
To do that we’re going to say outside the for loop, of course. Canvas.drawBitmap and inside it we will say m Bitmap which is not accessible again, so we have to mBitmap accessible. For that we go to or int method and make m Bitmap a global variable the same we did with mCanvas. You can go here and private Bitmap mBitmap.
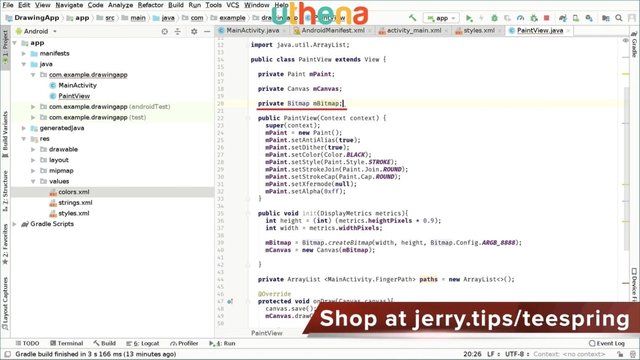
Now that can be accessed from the onDraw method and to draw path we will pass mBitmap right here and also pass where we want to start from. In this case, it will be 0, 0 so we want to start from scratch.
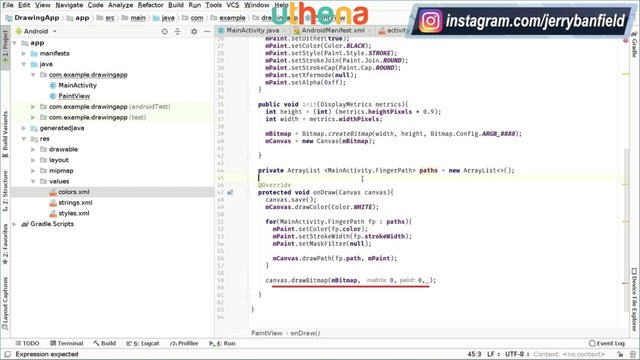
We have to pass the paint on this. To pass the paint of this we will create a new paint for this that will be called mBitmap paint, and we all create that as a global variable. You can create it right here. We went ahead and said private Paint mBitmapPaint. We will create this new paint and we’ll pass the parameter paint.DITHER_FLAG, so we can have it ready. We will use it as our last parameter right here so we’ll pass mBitmapPaint.
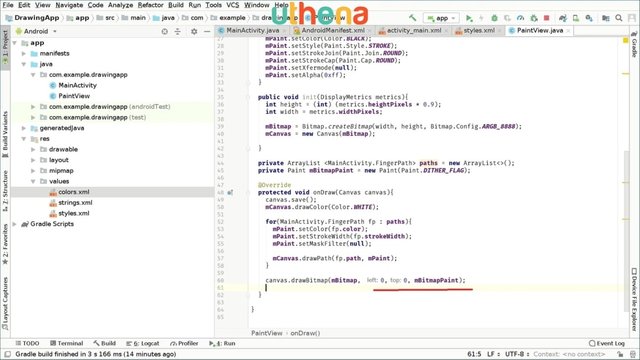
With this line of code we’re drawing the bitmap, and after we draw we will restore the canvas. You can call it canvas.restore.
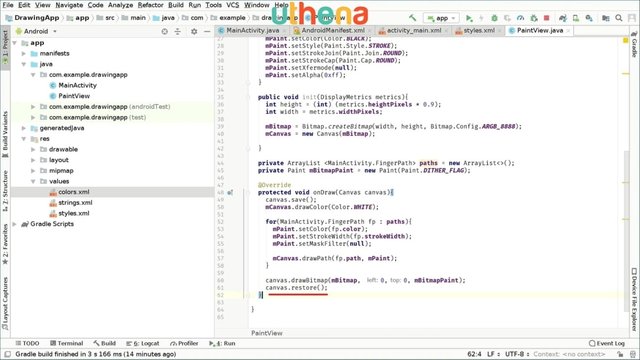
We have restored the canvas and now we have finished creating our onDraw method.
Part Two Of How To Make A Drawing App For Android.
In this video, we will continue working on our paintView.java class, so let’s start. In the previous tutorial we finished our onDraw method. Right now we have to set up a way in which we can get the event that the user generates when he or she touches the screen. For that, we will override the onTouchEvent. Go ahead and type @OverridePublicBoolean onTouchEvent. As a parameter we will have here the MotionEvent and we will call it an event. We are open to this and we will get the coordinates for this event. Since we have to return boolean we will return True. So this will be the last line of this method and we will continue working on the onTouchEvent.
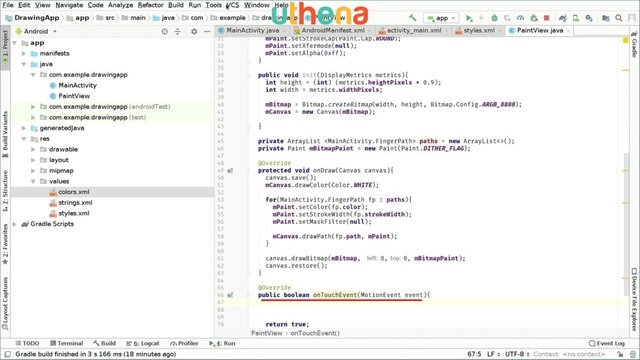
This event is called when the user touches the screen. We’ll see how it works right now so we can define two float variables. One of them will represent the coordinates at X and the other at Y.
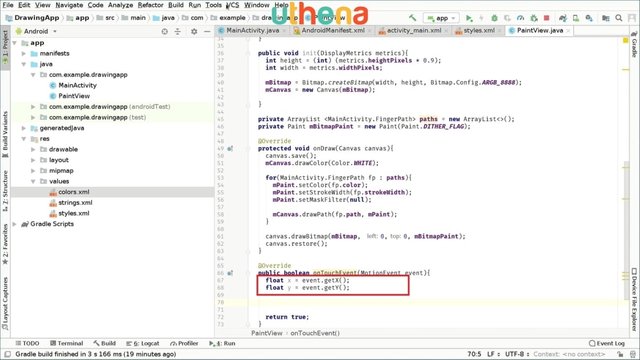
You can say float X will be set to event.get X and float Y will be set to event.get Y. We will take action. What do I mean by action? Well, when the user touches the screen, when the user does one action, when the user moves the finger around the screen that’s another action, and when the user stops touching the screen. That’s another action. You can ask for each action switch event.getAction to know which action we’re getting from the user. The first case will be the case MotionEvent in the case it is action down. So this is the case MotionEvent.ACTION_DOWN and here we’re going to basically say break. We will fill in this later but for now, we will leave them empty. That’s the first case. Now the next case will be an action move, so you’re going to call the case MotionEvent.ACTION_MOVE. We will break again. Break and end the line with semicolon.
Next, the last one will be the case MotionEvent.ACTION_UP, so when the user stops touching the screen. We would also say break.
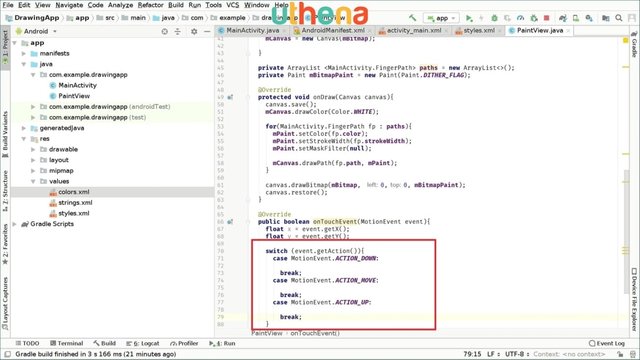
We have three kinds of actions the user can take when he touches the screen and we will use this float to pass them to another method which will enable the drawing to be created. What are those methods? Well, the first method will be called touchStart which will be set right here. From here we are going to say create a method which is private void touchStart. As parameters we will pass the coordinates right here. You’re going to get them here. For that we can say float X and float Y.
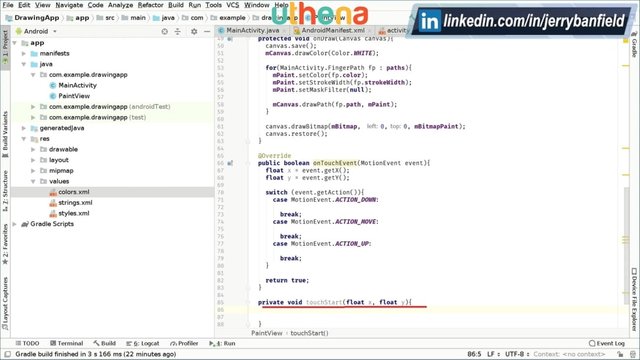
We will open this method and we will create a new path so when the user touches the screen we will start a new path. We’re going ahead and accessing the outer path to call that way. mPath will be set to a new path so this means we have to create a global variable which is a path and it’s called mPath. It’s red because we don’t have that yet so we have to create it. Here we’re going to type outside the method, of course, private Path mPath. As a path we have to import the correct path. In this case, it is the Android graphics path. Now we have our mPath that we can use to draw.
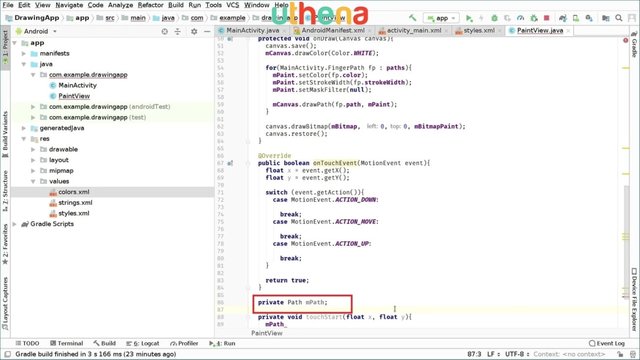
So this is the TouchStart method and the first thing is we’ll set to mPath will be creating a new path right. New path. The next thing we’ll do we will create a finger path which was created on our main activity. We are going to call it MainActivity.fingerPath and we call this fp. This is set to the new MainActivity.fingerPath, and we have to pass the color of what we want the path to be. In this case, we want it to be black then we can change it. So color.BLACK. So we’ll pass the black color, and we’ll pass the strokeWidth.
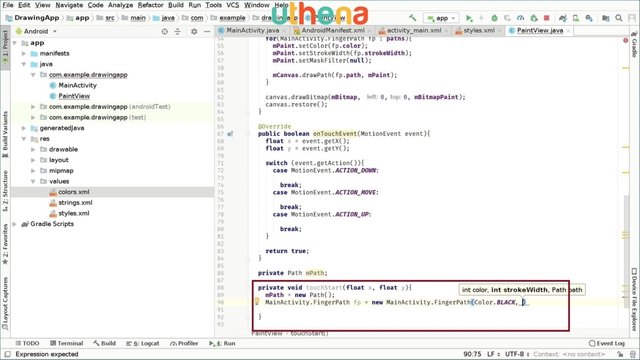
How can we know how big should the strokeWidth be? Well, basically we will start with a basic stroke with size which in this case will be 10 which is kind of a slim stroke width. We can then increase the stroke width if we want or decrease. I don’t think it will decrease but if you want you can. In touchStart we passed the color, the strokewidt, the color is black and the strokeWidth will be 10 and we have to pass the new path which in this case is mPath.
In order for us to add this path to the place in which we stored the other paths we’ll go and say paths.add and we will say add fp. We have to reset the path and move to the coordinate the user is touching.
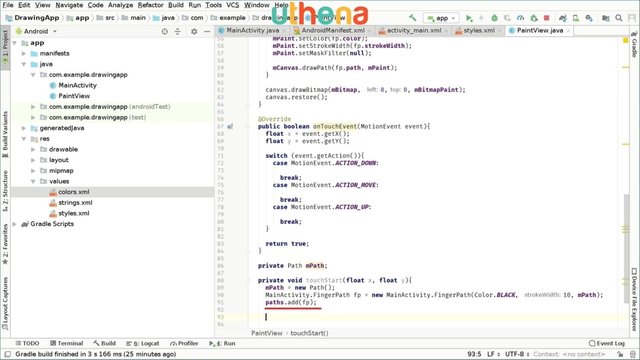
To do that we’re going to say mPath.reset and then we will say mPath. moveToX, Y). We have our new path.
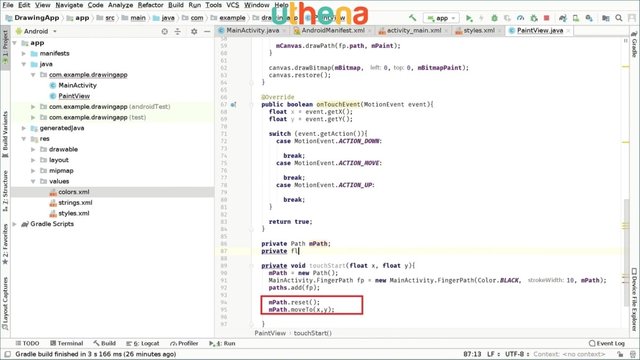
If we want to keep track of the current movement of the user we will have to create global variables that will help us do that. For example, we will create two variables to store the current X and Y position. We can say private float mX, and we’re going to say private float mY. After we move to this path we will set and save those coordinates for later use, so we’re going to say mX is set to X and mY will be set to Y. This is basically what we will do with the touchStart method.
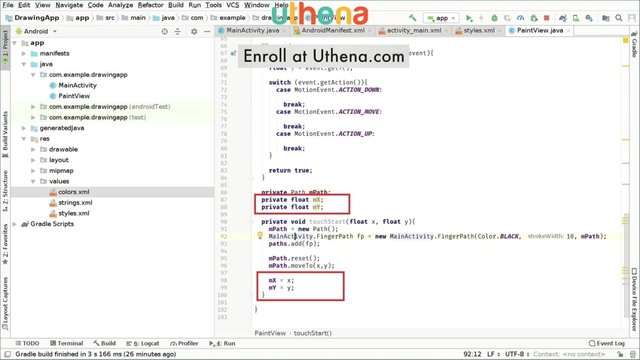
Where do we have to call this method from? Well, we will call it from our onTouchEvent.
This is the first method transaction. I will go to our onTouchEvent which is right here and in action down we have to call touchStart. You can say touchStart and we’ll pass X and Y. After all this the view class requires us to invalidate the previous view in order for us to make it look smooth. To do that they’re going to say just invalidate.
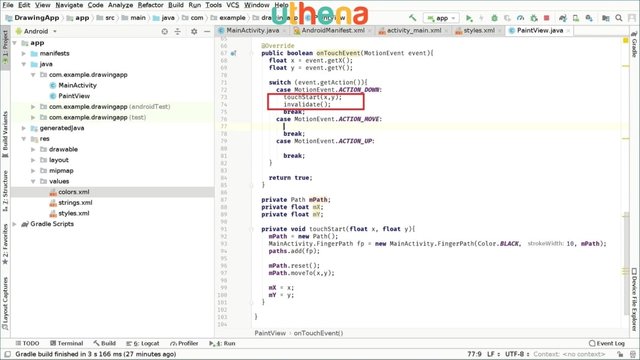
Next we have the action move so for this action we will create another method.
What method will that be? Well it will be the onTouchMove method. For that we will go and create them right here.
We can say private void onTouchMove. Again, this parameter, we’ll have X and Y. So float X, float Y.
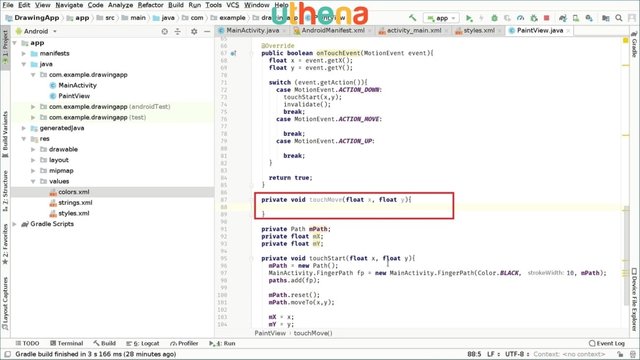
Inside this we will have to understand how much the finger has moved from the previous position which was saved with these variables I’m selecting right now.
To do that we will say the following float the X which means changing X will be set and with the math library we’ll kind of calculate the distance between them. You’re going to say the difference between them. You can say math.abs(X -mX). The difference between the current X and the saved X. We will do the same with Y. So you can say float dY will be set for math.abs( Y-mY).
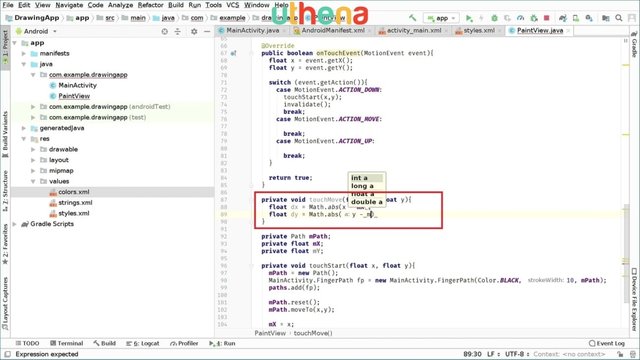
GET MORE OF THIS COURSE
Would you like to continue learning about the Program Your Own Drawing Application in Android Studio Course? If you are interested will you please buy the complete course, Program Your Own Drawing Application in Android Studio Course, on the Uthena Education Platform..
You can also get the first 45 minutes of the course completely for free on YouTube.
Thank you for reading the blog post or watching the course on YouTube.
You may like to read these posts: Android File Manager App Creation and Code Your Own Video App Player in Android Studio!
I love you.
You’re awesome.
Thank you very much for checking out the Program Your Own Drawing Application in Android Studio Course and I hope to see you again in the next blog post or video.
Love,
Jerry Banfield.
Posted from my blog with SteemPress : https://jerrybanfield.com/program-your-own-drawing-application-in-android-studio/
best app for android, please vote and download driver printer https://steemit.com/hpenvy4510driver/@softdriver/4dg3zf-epson-xp-200-driver-and-software-download
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit