Hello friends, this is a blog about my programming journey, I am basically teaching myself how to code. This is a formal documentation about my progress.

There are instances where it becomes necessary to generate random outcomes in programming, the inbuilt random module can be used in such scenarios.
The syntax for using a random module is as shown below:
import random
outcome = random.randint(1,10)
The simple code is used to generate random integers between 1 and 10. The first line imports the random module into the program and the second line stores a random number between (1-10) into the outcome variable. The program therefore generates a random number each time it runs.
We can also use a for-loop to generate random outcomes in a given number of times.
import random
for count in range(5):
print(random.randint(1,10))
The code above simply generates 5 random numbers between(1-10).
The first line imports the module into the program. the second line starts a loop that runs 5 times, and the third line prints the random integers.

Create a shell-based interactive guessing game that asks the user to guess numbers between 1-10, give the user hints along the way

The first step is to import the module and assign a variable that stores our random values. this variable(x) would be also used later in our program.
The second step is to accept our guessed number from the user and store it into the guess variable(which will be used later to test against the randomly generated number in the x-variable)
Because we don't know how many times the user will make a wrong guess, we have to create and infinite loop that keeps running until our user makes the right guess.
In the next step,inside the while loop, we will create a couple of if-conditional-statements. like the name implies. if the condition is true, it runs the if-code-block below the if-statement.
This if-code-block below simply contains a print statement to tell the user about his success and a break-statement to break out of the infinite-loop when the user is correct.
In other to give our user some hints to guide the guess. we'll create some couple of if-statement to test if the guess(input by the user) is lower or higher than the randomly generated(x-variable)
These if-statements above doesn't contain a break statement to escape the infinite loop because we want them to keep running in the loop since the user is not correct yet, the if-statements also contains a input function to accept a new value and stores into variable(guess) to test again with our variable(x).
import random
x = random.randint(1,10)
guess = int(input("Guess a number between 1 - 10"))
while True:
if guess == x:
print("you are correct!!! here have a cookie\n")
break
if guess > x:
print("That is too high buddy, try again?")
guess = int(input("Guess a number between 1 - 10"))
if guess < x:
print("That is too low buddy, try again?")
guess = int(input("Guess a number between 1 - 10"))
If we compile and run the code above we get something like this.

Create a random dice throwing game

This is much more easier with just a couple of lines.
Using the random fuction we can generate numbers between 1 and 6 which can be stored in our die 1 and die 2 variable. these variables can be used later in our program.
We can now use the print statement to display the two dies to the user screen.
import random
die1=random.randint(1,6)
die2=random.randint(1,6)
print('you rolled', die1, 'and', die2)
input('press enter to exit')
If we run the code we get something like this:
That is all for today, thanks for visiting my blog
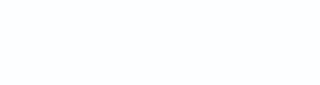

Your post was featured here:
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Looking at coding gives me a headache! Hahaha
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
lol... thanks for passing by @joelai... It does takes some getting used to
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
I don't think I can... :D
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
:-)
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit