Some of you might know that I do payouts and such for @platforms. I did it manually at first and then realized that it would take way too long, so I wrote up a script to do it for me. I have some work to do, but 5 minutes of work every time is a lot easier than 20 minutes now, and 500 minutes later when there are tons of people to pay out.
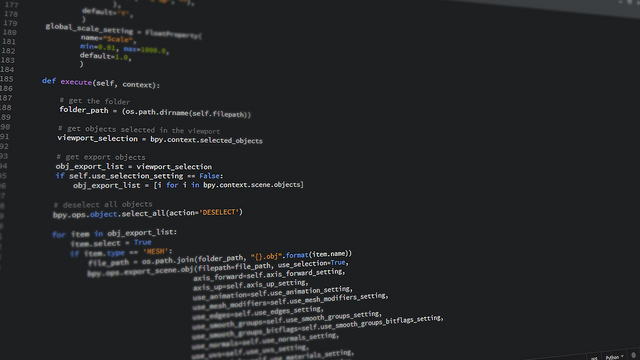
Source
Please understand that there might be mistakes in my code, and I would recommend testing the code out with an account with just enough money that you are willing to lose to make sure theres no errors in it. I'm not responsible for lost funds.
I'll explain the code pretty much chuck skipping over unnecessary stuff like comments) and post the entire code at the end so you can modify it to your liking. This tutorial is designed for people who have a basic python knowledge or knowledge of other programming languages, but it should be pretty easy to follow along even if you are code illiterate.
To start, you import the Steem library into with the following line:
from steem import Steem
Next you want to set up a variable to represent Steem:
s = Steem()
After that you will want to define a list of accounts to who you will be sending along with how much you'll be sending to them. A simple dictionary works perfectly, with the key being who you want to send to and value for the key being how much you want to send to them. While this will be tedious to write out each time, I use google sheets and a few other things to simplify my time with it. I don't write it out myself. Theres tons of ways to get this, but I won't go into that, lets just say you already have it in this format. This is what a smaller version of the dictionary will look like, but the dictionary will grow to get much bigger as you have more people to send to:
accounts = {'jglake': 0.076, 'steemitqa': 3.043, 'weberh8': 2.697, 'taskmanager': 0.39}
The following hunk is setting up information to send. The coin variable can be either STEEM or SBD. I have that set as an input so I can easily change it every time I run it instead of having to change it in the code. The fromAccount variable is the account that the funds will be sent from. Because I won't be changing that in the future, I set it up right in the code, but this too can be set up as an input from the user. yourMemo is the memo that you will be using when sending the funds. Its possible to customize the memo, but for the proposes of this tutorial, it will be the same for each user. privatePosting and privateActive are pretty self explanatory. Your private posting key and active keys go within the quotation marks. Its possible to get that as an input as well. Here's the hunk:
coin= input("What coin do you want to send? Choose STEEM or SBD.") #What coin to send
fromAccount = 'platforms' #from what account to send
yourMemo = "Your Share of The Payout For April" #the memo
privatePosting = "" #private Posting Key Goes There
privateActive = "" # Private Active Key
Now onto the stuff about sending. This is pretty important stuff. I made it a function so you can call it when you need to. To start, you basically unlock your wallet by providing the keys. Because we already entered our keys above, we can just call the variables. Now we want a list of just the people we are sending to, not the amount we are sending. To do that, you convert the keys of the dictionary into a list. From there, you iterate through the list, sending payments to each person along the way. The s.commit.transfer starts the transaction. The to field is the person you are sending it to, and since we have it in a list that is being iterated through, we can pass the variable with the name of the person we send to. The amount is how much you are sending. To get that, we just look up the value for the person in our dictionary with the name and amount. We pass along the account we are sending from and the memo too. Here's the hunk:
def sendCoustom(sendDict):
s = Steem(keys=[privatePosting, privateActive])
listAccount = list(sendDict.keys())
for i in listAccount:
s.commit.transfer(to=i, amount=sendDict[i], asset=coin, account=fromAccount, memo=yourMemo)
Finally we want to create a test function to see how much each person will get. This is useful to check to see if you messed up somewhere. We start of with the exact same thing as the actual sending function, but instead of sending to each person, we just print out their name and how much they will get paid, copying and pasting the way we get their name and their payment from above to make sure you have no typos and your actual sending amount will be what your test shows.
def test(sendDict):
listAccount = list(sendDict.keys())
for i in listAccount:
print(i,sendDict[i])
Now thats it, we can call the test function to see if it will work and if so, we can call the send function to send to people. Here's the full thing put together:
from steem import Steem
s = Steem()
accounts = {'jglake': 0.076, 'steemitqa': 3.043, 'weberh8': 2.697, 'taskmanager': 0.39}
#set up sending stuff
coin= input("What coin do you want to send? Choose STEEM or SBD.") #What coin to send
fromAccount = 'platforms' #from what account to send
yourMemo = "Your Share of The Payout For April" #the memo
privatePosting = "" #private Posting Key Goes There
privateActive = "" # Private Active Key
def sendCoustom(sendDict):
s = Steem(keys=[privatePosting, privateActive])
listAccount = list(sendDict.keys())
for i in listAccount:
s.commit.transfer(to=i, amount=sendDict[i], asset=coin, account=fromAccount, memo=yourMemo)
# To use sendCoustom, call it and pass accounts
def test(sendDict):
listAccount = list(sendDict.keys())
for i in listAccount:
print(i,sendDict[i])
If you want to use the code to test it out, use pip to install steempy and then add this line to the very bottom : test(accounts)
to see how much you will send to each person and then add sendCoustom(accounts)
when you are ready to send. To run the rest code, you don't need to add in private and active keys, only when you want to send do you need to insert them in. I recommend deleting it from that as soon as you send so that way someone else can't login and find your keys. Be safe with them.
Very thorough. I really like the idea of a test run of the code.
Have you written code for master-delegator yet?
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Yeah, the test is importnat, especially for what I plan to share today.
Ive been working on the code for master delegator, but im having a hard time with the bitshares aspect if things. Going to spend time on it this weekend.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Great and amazing post sir.
Nice content
Very well articulated
I really loved your post. 😍😍😍
please check out my post once🤗💙
Thanks @rishi556 for sharing this post.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Intellectual post.Really impressive. Obviously you done a great job.Thanks.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit