Hello Everyone
I'm AhsanSharif From Pakistan
Greetings you all, hope you all are well and enjoying a happy moment of life with steem. I'm also good Alhamdulillah. |
---|
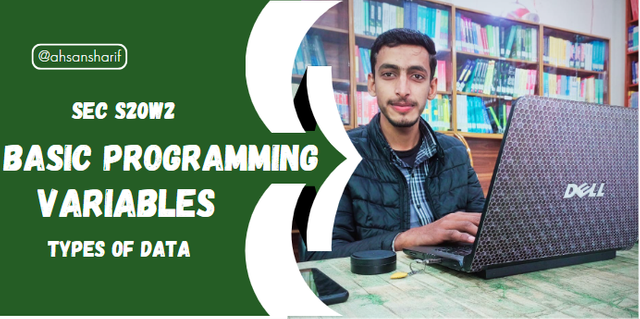
Made in canva
What are variables and what are they used for? |
---|
Variable
Variables are like containers that store data values. In programming, it acts as a placeholder that you can update and manipulate in your program throughout the program. These variables make the code more flexible and reusable. Because you can assign them different values without changing the program structure.
Uses of Variable
Storing Data:
Variables hold values such as numbers text and various complex data types.
Example: let age = 25;
Making Code Dynamic:
Using variables a program can perform actions on different inputs.
Example: let x = 5; let y = x * 2;
(value of y
depends on x
)
Data Manipulation:
Using variables we can perform different operations like addition and subtraction or even complex algorithms.
Example: total = price * quantity;
Improving Readability:
Naming variables makes the code easier to understand, so it improves our readability.
Example: let userName = "Alice";
Types of Variable
Integer: Whole numbers like 1, 10, 50.
String: Text or sequence of characters like "Hello World".
Boolean: True or false values used in logical expression.
Array or object: Used to store multiple values or complex data.
Assign a type of data to the following variables and explain why: email, phone, working_hours, price_steem, and age. |
---|
Here I am explaining how we assign variables to data types and their explanation.
Variable | Data Type | Explanation |
---|---|---|
string | A sequence of characters contains an email address, so we prefer to represent it as a string such as letters, numbers, and symbols. (e.g., [email protected]) | |
phone | String | A phone number contains digits but is often stored as a string. Because it sometimes contains some special characters like plus minus and spaces. The phone number does not need to go through the math process. |
working_hours | Number (Integer or float) | Work hours are usually shown as numeric values to represent hours worked in a day or week. |
price_steem | Number (float) | The price of a currency like Steem often includes decimals. As in 2.45, and 2.78, a floating point would be appropriate. |
age | Number (Integer) | Integer is best for this variable because it usually contains a whole number to represent the year. |
Explain how the following code works: |
---|
Define name, last_name as Character;
Print "Enter your name:";
Read name;
Print "Enter your last name:";
Read last_name;
Print "Hello " name " " last_name ", welcome";
EndAlgorithm
Define Variable:
We recognize name
and last_name
as character data types or as strings, which means they store text data.
Define name, last_name as Character;
Prompt for First Name:
In this, the program prints a message such as "Enter your name:" to prompt the user to enter their first name.
Print "Enter your name:";
Read User Input (First Name):
Here the program waits for the user to type his first name and stores his input in a variable name
.
Read name;
Prompt for Last Name:
The program prints the message "Enter your last name:" to prompt the user to enter their last name.
Print "Enter your last name:";
Read User Input (Last Name):
The program waits here for the user to type his last name and stores his input in a variable last_name
.
Read last_name;
Display Greeting Message:
Finally, the program prints a greeting message using the user's input. The output is in the format: " Hello
<name>
<last_name>
, welcome
".
It concatenates the name
, a space
" "
, and the last_name
into one string before displaying it.
Print "Hello " name " " last_name ", welcome";
Example:
If the user inputs:
- First name:
Alice
- Last name:
Smith
The final output would be:
Hello Alice Smith, welcome
Develop a pseudo-code to calculate the value in USD of X STEEM. For this you can rely on this algorithm: |
---|
I have a DEV C++ software on my laptop so I develop this code in C++. Here is the code:
#include <iostream> // For input and output operations
using namespace std;
int main() {
// Step 1: Declare variables
float price_steem, amount_steem, total;
// Step 2: Request the user for the amount of STEEM
cout << "Enter the amount of STEEM: ";
cin >> amount_steem;
// Step 3: Request the user for the current price of 1 STEEM in USD
cout << "Enter the current price of 1 STEEM in USD: ";
cin >> price_steem;
// Step 4: Calculate the total value in USD
total = amount_steem * price_steem;
// Step 5: Show the result on the screen
cout << "The total value of your STEEM in USD is: " << total << endl;
return 0;
}
Input:
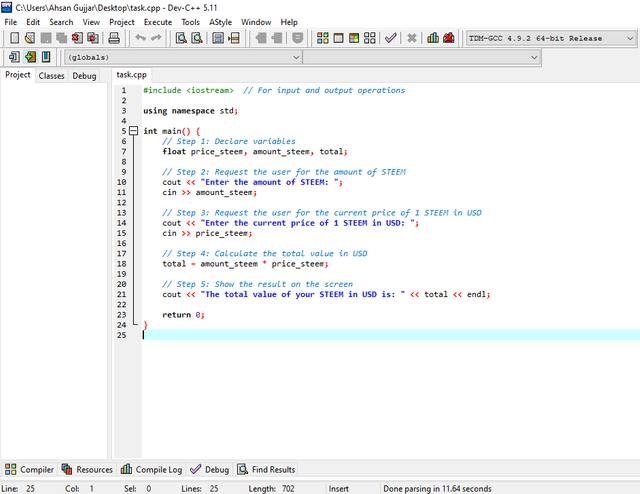
Secreenshot of Input From DEV C++
Example of Execution:
User Input:
Enter the amount of STEEM: 100
Enter the current price of 1 STEEM in USD: 0.25
Output:
The total value of your STEEM in USD is: 25
This code follows the basic steps of input, process, and output, making it easy to understand and implement.
Output:
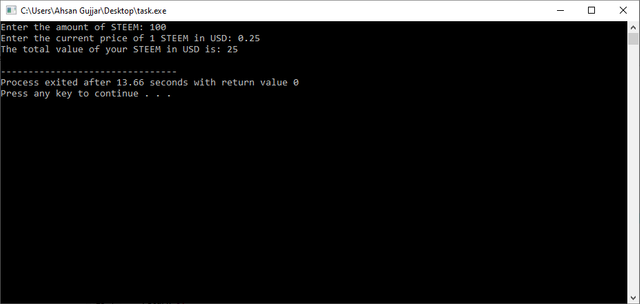
Secreenshot of Execution
Thank you so much all for staying here. Here my task is complete.
X:
https://x.com/AhsanGu58401302/status/1835928297848602676
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Upvoted. Thank You for sending some of your rewards to @null. It will make Steem stronger.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
!upvote 25
💯⚜2️⃣0️⃣2️⃣4️⃣ Participate in the "Seven Network" Community2️⃣0️⃣2️⃣4️⃣ ⚜💯.
This post was manually selected to be voted on by "Seven Network Project". (Manual Curation of Steem Seven).
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
the post has been upvoted successfully! Remaining bandwidth: 90%
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Your post has been rewarded by the Seven Team.
Support partner witnesses
We are the hope!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hola amigo, has hecho un buena explicación acerca de lo importante que son los tipos de variables en programación y has dado ejemplo de las existentes.
El programa que realizaste en C++ esta muy entendible y lo bueno de todo es que mostraste la ejecución.
Hiciste una buena entrada, por lo que te deseo mucho éxito en la misma.
Saludos.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thank you so much for your valuable feedback. I'm happy to see you here. I wish you more success too
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit