Hello Steemit friends!
1. What are variables and what are they used for?
Variables are storage spaces that allow information to be stored in a program. They play a vital role in computer science because they allow data to be manipulated dynamically. When I explain this concept to my students, I often compare variables to storage boxes in which you can place different objects (the data) each box having a label (the variable name) and a specific type of object that it can contain (the data type).
When I want to explain the importance of variables to my students, I often use a simple example like the pen example. I ask them to give me a pen without reaching out or pointing to a specific place to put it.
They often look at me puzzled, and that's when I explain: "You can't give me the pen because I didn't tell you where to put it." In computer science, it's the same thing. If we want to store data (like the pen in this example), we must first designate a location where to put it, this is called a variable. The variable is like a hand or a box that allows us to keep the data safe so that we can use it later.
So, in the same way that they would need to know where to put the pen to give it to me a program needs to know where to store information to manipulate it effectively.
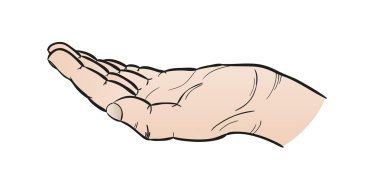
Source
2. Assign a type of data to the following variables and explain why:
email:
Character
- Why: An email address consists of a combination of letters, numbers, and symbols (e.g.,
[email protected]
), which makes it a sequence of characters. In most programming languages, strings are used to store textual data.
- Why: An email address consists of a combination of letters, numbers, and symbols (e.g.,
phone:
Character
(orInteger
depending on usage)- Why: While phone numbers may appear to be numerical, they often include special characters like "+" or dashes ("-"). In many cases, phone numbers are treated as strings to preserve formatting. If strictly numerical without special characters,
Integer
can be used.
- Why: While phone numbers may appear to be numerical, they often include special characters like "+" or dashes ("-"). In many cases, phone numbers are treated as strings to preserve formatting. If strictly numerical without special characters,
working_hours:
Integer
orReal
- Why: Working hours typically represent a numerical value, either in whole hours (
Integer
, e.g., 7 hours) or in decimal form (Real
, e.g., 6.5 hours for 6 hours and 30 minutes).
- Why: Working hours typically represent a numerical value, either in whole hours (
price_steem:
Real
- Why: The price of STEEM (or any cryptocurrency) fluctuates and often includes decimal points (e.g., 0.25 or 1.25 USD). Therefore,
Real
is the most appropriate type for precisely handling these values.
- Why: The price of STEEM (or any cryptocurrency) fluctuates and often includes decimal points (e.g., 0.25 or 1.25 USD). Therefore,
age:
Integer
- Why: Age is typically a whole number that represents the number of years. Therefore,
Integer
is the best fit for this variable.
- Why: Age is typically a whole number that represents the number of years. Therefore,
3. Explain how the following code works:
Algorithm names
Define name, last_name as Character;
Print "Enter your name:";
Read name;
Print "Enter your last name:";
Read last_name;
Print "Hello " name " " last_name ", welcome";
EndAlgorithm
Explanation:
Step | Description |
---|---|
Define name, last_name as Character | Declare the variables name and last_name to store strings (text). |
Print "Enter your name:" | Display a message asking the user to enter their first name. |
Read name | The program waits for the user to enter their first name and stores this entry in the variable name . |
Print "Enter your last name:" | Displays a message asking the user to enter their last name. |
Read last_name | The program waits for the user to enter their last name and stores this entry in the variable last_name . |
Print "Hello " name " " last_name ", welcome" | The program concatenates "Hello", the first name (name), a space, the last name (last_name), and the string ", welcome", and then displays the complete message. e.g., "Hello Bouchamia Bilel, welcome." |
4. Develop a pseudo-code to calculate the value in USD of X STEEM.
Algorithm steem_to_usd
Define price_steem, amount_steem, total as Real;
// Request the user to input the necessary data
Print "Enter the current price of STEEM in USD:";
Read price_steem;
Print "Enter the amount of STEEM you have:";
Read amount_steem;
// Calculate the total value in USD
total = price_steem * amount_steem;
// Show the result
Print "The total value of your STEEM in USD is: " total;
EndAlgorithm
Thank you very much for reading, it's time to invite my friends @josepha, @lhorgic, @mohammadfaisal to participate in this contest.
Best Regards,
@kouba01
@tipu curate
;) Holisss...
--
This is a manual curation from the @tipU Curation Project.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Upvoted 👌 (Mana: 4/8) Get profit votes with @tipU :)
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Upvoted. Thank You for sending some of your rewards to @null. It will make Steem stronger.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
The last time I used the PSeInt application I enjoyed using it despite how it is not written in English. From your post, anyone who read through would get to know how to use the application based on how well you have presented your work. Thank you for inviting me and good luck to you.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
It's good to know that you enjoyed using PSeInt and found my post helpful. It's always a pleasure to share knowledge and guide others through the process. Good luck to you as well.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Wow you have already done it. It is amazing. And thanks for inviting me I am also planning to perform it.
Variables are the containers which hold the values for their use in the programs. There are different variables with their data types and we can initialize them according to their needs.
We can make the variables private as well as public. And you have assigned all th data types to the variables greatly.
A wonderful and full of knowledge entry.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thank you for your comment! I'm happy that you found the introduction helpful and appreciated the way variables and data types are explained. I'm glad to hear you plan to try it out too. Good luck!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
@tipu curate
;) Holisss...
--
This is a manual curation from the @tipU Curation Project.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Your post has been rewarded by the Seven Team.
Support partner witnesses
We are the hope!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
TEAM 5
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
I accept your invite wholeheartedly...will be making an entry soon.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
I also explain variables as boxes, but your example with a handle - which should be transferred, not given))) I really liked it, I will use it.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
I'm happy to know that you liked the handle example! Indeed, I often think of how relevant analogies like this one can make complex concepts easier for my students to understand. I'm honored that you're thinking of using it in your own explanations, and thank you for your kind words!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hola amigo, realmente usted es un buen profesor, ha explicado de manera muy detallada los tipos de variables y la importancia de declararlas, como bien dice, hay que ponerle lugar a la información o datos que se introducirán como entrada en el programa.
Las tareas practicas están muy bien desarrolladas y entendibles.
Le deso muchísimo éxito en su entrada y en todo en cuanto emprenda.
Saludos.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit