Well, hello there, curious minds!
Today I thought myself about joining the Basic programming course: Lesson #3 Operations [ESP-ENG] by @alejos7ven.
Let's not waste time and start working, this is going to a be a long post.
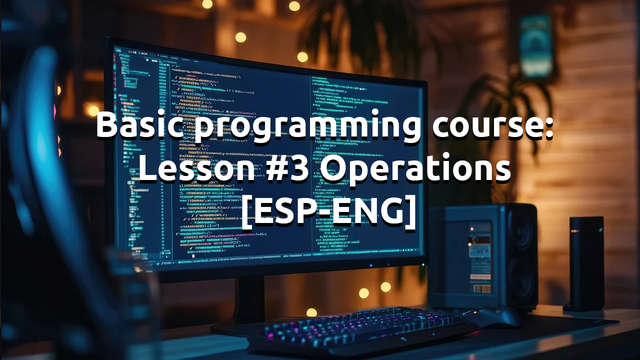
Thumbnail taken from Pixabay
Homework
First point
Give a brief summary of what arithmetic, comparison and logical operations are for.
Arithmetic Operations
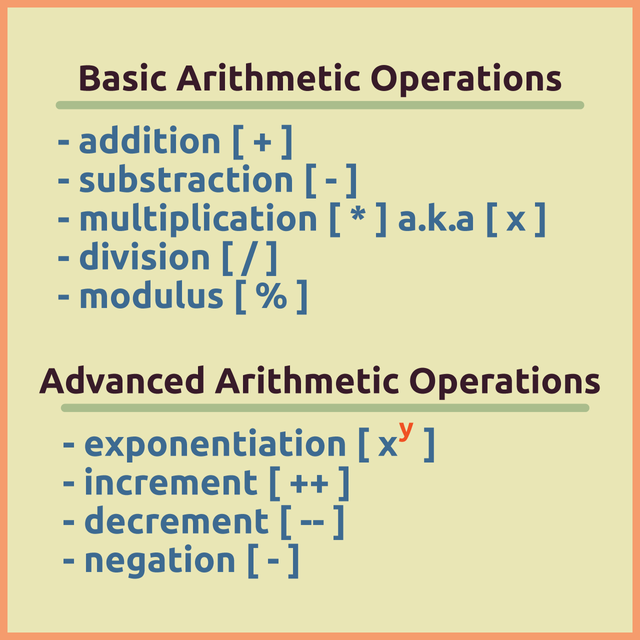
The basic and advanced arithmetic operations are used to perform calculations and are the base of any algorithm in programming.
Besides the basic ones that everyone heard about, we also have some advanced operations like:
- pow: exponentiation - you raise a number to the power of another
- increment: where you increment the current value by one
- decrement: where you decrement the current value by one
- negation: change the sign number positive -> negative / negative -> positive
Basic: + - * / %
Advanced: pow ++ -- -
Example:
int x;
x = 4+5-3*6;
printf("%d",x);
In this case we use the basic ones to calculate the value of X;
int x;
x=5;
x++;
printf("%d",x);
In this case we use the advanced one to increment the value of x by one, here X becomes 6 after being incremented by one.
Comparison Operations
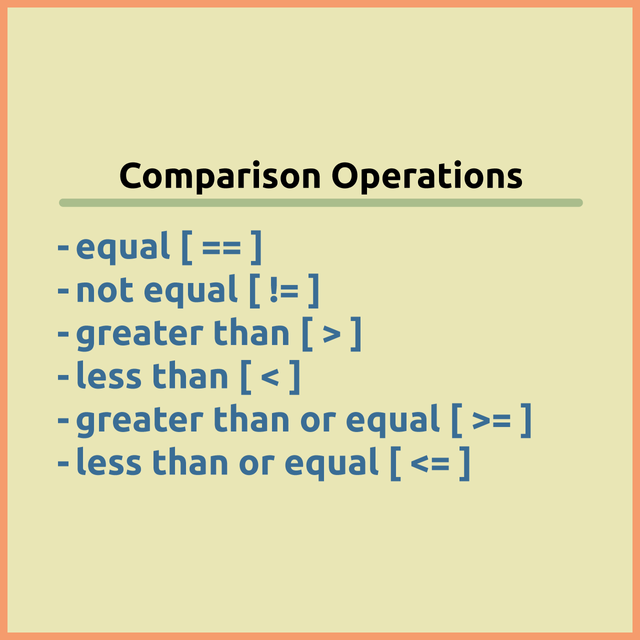
We use the comparison operations to check the relationship between two or multiple values. While using them the values returned can either be TRUE or FALSE. These are mostly used in conditions, expressions and loops to control the flow of the code.
Operations == != > < >= <=
Example:
if(5>2)
{
printf("5 is bigger than 2");
}
else
{
printf("5 is not bigger than 2");
}
if(12 != 2)
{
printf("True");
}
else
{
printf("False");
}
Logical Operations
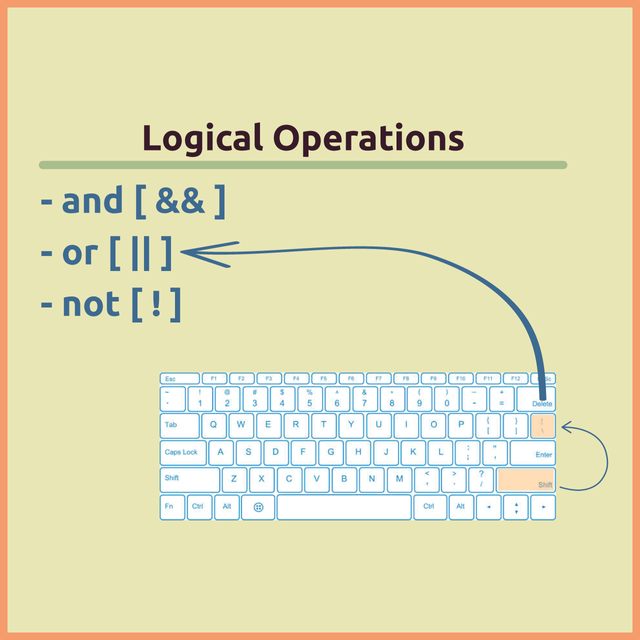
Logical Operations are used in structures that require decisions, with these we can create more complex conditions (multiple conditions in one).For these we also have some truth tables that can help you understand the operations.
AND (&&) Table
A | B | A && B |
---|---|---|
true | true | true |
true | false | false |
false | true | false |
false | false | false |
Example:
if(5>2 && 3<11)
We check it like this, 5>2? -> True 3<11?-> True, now check the table row for True and True -> True, means our condition will return True.
if(12>6 && 23<11)
We check it like this, 12>6? -> True 23<11?-> False, now check the table row for True and False -> False, means our condition will return False;
OR (||) Table
A | B | A or B |
---|---|---|
true | true | true |
true | false | true |
false | true | true |
false | false | false |
Had to use OR in the table header because markdown uses | for tables already
Example:
if(25>12 || 11<3)
Let's see, is 25 > 12? -> True what about 11<3? -> False, let's check the table True or False returns us True in this case, we need only one of them to be True while using OR.
NOT (!) Table
A | !A |
---|---|
true | false |
false | true |
Operations && || !
Second point
Make a program that asks the user for 2 numbers and evaluates if both numbers are the same.
For this point of the homework I am going to use an online C compiler, this one. I tried getting the teacher's software but it looks like it is not in english as far I see.
Now let's get started with some C.
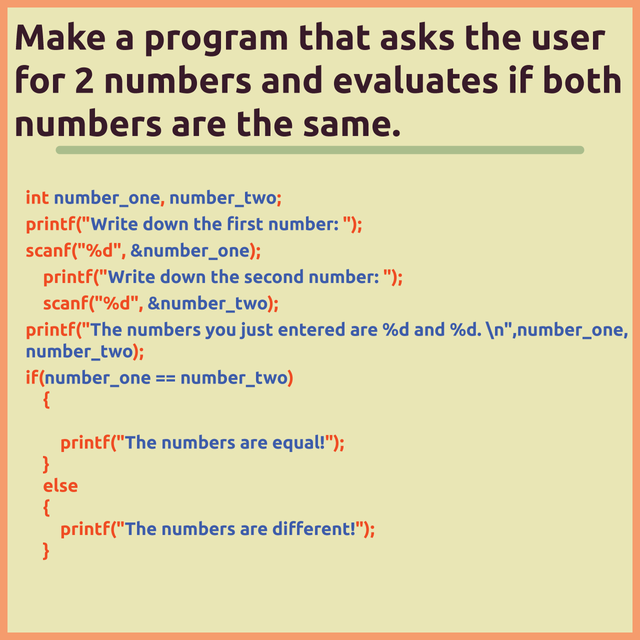
We'll assume our numbers are integers and declare them as number_one and number_two.
int number_one, number_two;
We have the variables declared, let's print a message to the user and let him know he needs to give us a number, we do this using printf function.
printf("Write down the first number:");
This will let the user know he needs to give us a number, after this we need to get the value the user entered and store it in our first variable, to do that we use scanf function.
scanf("%d",&number_one);
The %d
is used to let the program know we are going to give it an integer value (integer means the value could be positive, negative or zero), and the & is used to avoid a warning related to pointers, in some compilers it works even without adding it but in this case it was added to avoid the warnings in the compiler.
We have the first value, now we are going to to the same for the second one.
printf("Write down the second number:");
scanf("%d",&number_two);
Let's show the user the numbers he just entered:
printf("The numbers you just entered are %d and %d. \n",number_one,number_two);
, we use the %d in the printf text to let the compiler know where we want the two values to be printed in our message, as many %d we add in the text, we need that many variables after the text, we have two %d in our case so we add our two variables after, number_one,number_two. The \n
is used to create a new line after this text.
Let's compare our numbers now, we are going to use a condition:
if(number_one == number_two)
{
printf("The numbers are equal!");
}
else
{
printf("The numbers are different!");
}
We use the comparison operator ==
to check if number_one is the same as number_two and based on that condition we print a message to the user.
The whole code in the compiler looks like this:
And the results in both cases look like this:
When numbers are different!
When numbers are not different!
Third point
Transform the following mathematical expressions into arithmetic expressions on your computer:
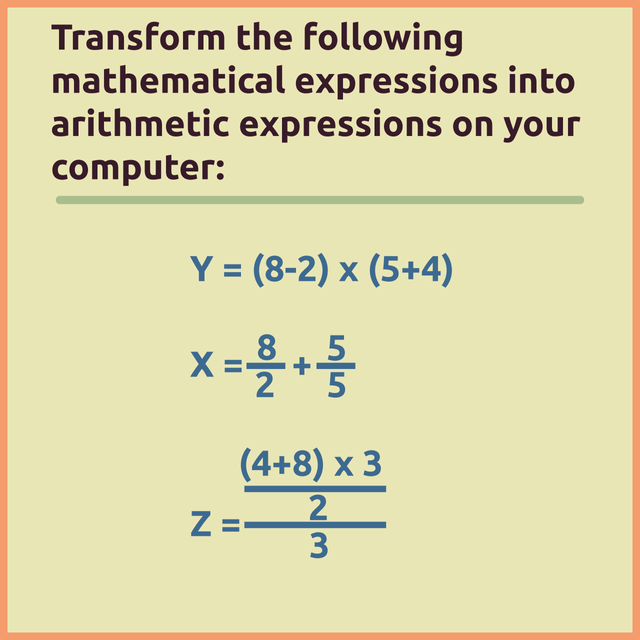
Evaluate if the results of the 3 operations are greater than or equal to 0 (>=) and show it on the screen.
Let's get started, we have the first two expressions that are easier:
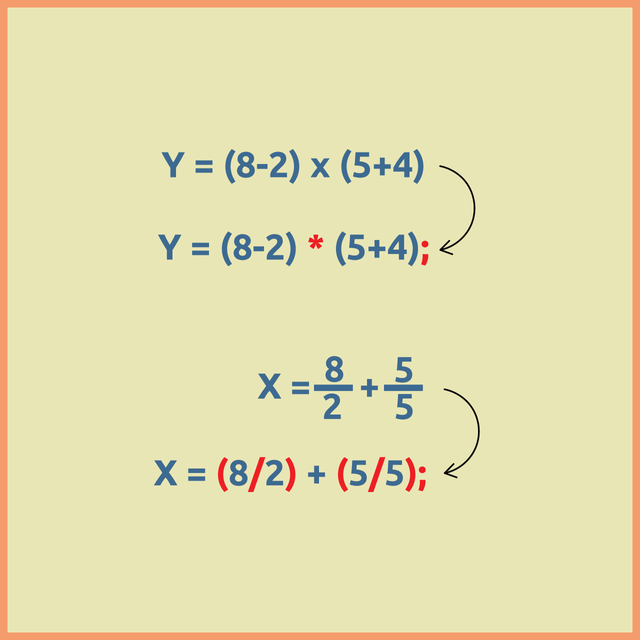
For the first one we have to replace x with * for our compiler to understand the calculations.
Y=(8-2) * (5+4)
(8-2) * (5+4) -> 6 * 9 -> Y = 54
The second one, we need to add the division where we have the fraction, and it becomes like this and add the corresponding parenthesis:
X=(8/2)+(5/5)
(8/2)+(5/5) -> 4+1-> X=5
As for the last one, here we are going to split the expression so we can understand it easier:
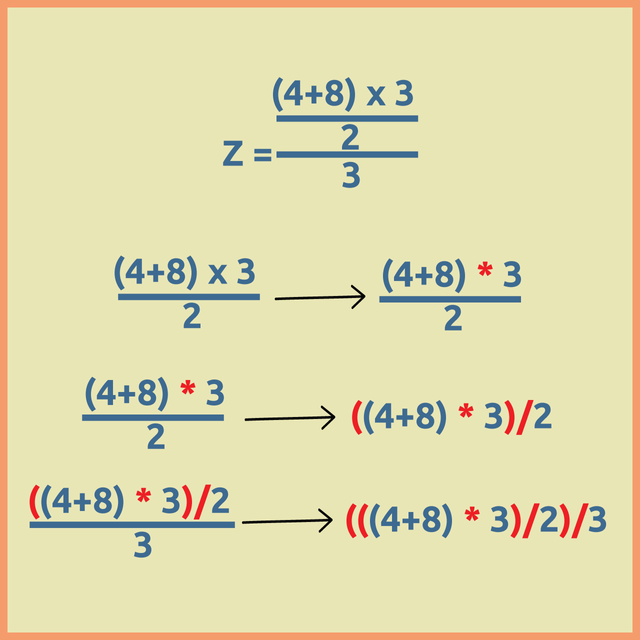
From the process described above we get:
(((4+8)*3)/2)/3
(((4+8)*3)/2)/3 -> ((12 * 3)/2)/3 -> (36/2)/3 -> 18/3 -> Z=6
Now let's code it, before we define our expressions let's assume that Y will be an integer since there's no division while X and Z will be float since we have division and the number might have decimals.
The expressions in our code will look like this:
int y;
float x,z;
y = (8-2) * (5+4);
x = (8/2) + (5/5);
z = (((4+8) * 3 )/2)/3;
To print the results of our expressions we'll use printf again:
printf("Y:%d\nX:%.2f\nZ:%.2f\n",y,x,z);
%d
is for integer, %f
for floats (numbers with decimals) and the .2
is used to tell the compiler that we want to show only 2 decimals.
The code in the compiler would look like this:
And one we run it, the result in the console is as it follows:
That was it for this homework, in the end I'd like to invite @r0ssi, @titans and @mojociocio to join this.
Thank you for stopping by, until next time I am wishing you a great day!
Your post has been rewarded by the Seven Team.
Support partner witnesses
We are the hope!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit