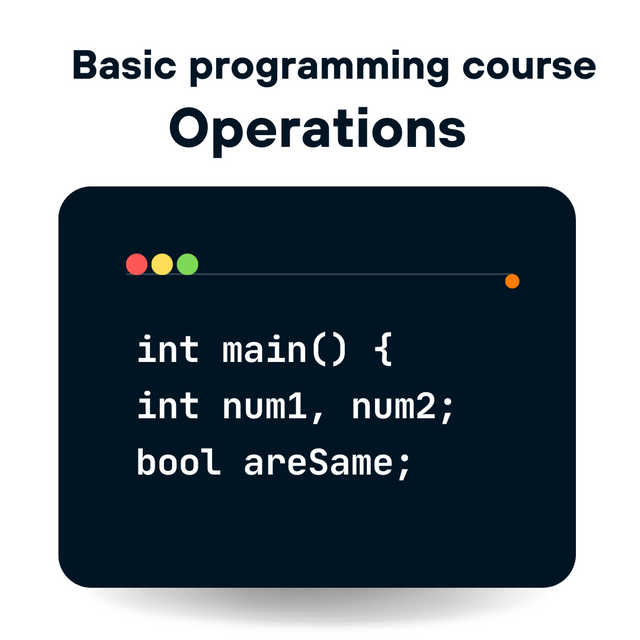
Give a brief summary of what arithmetic, comparison and logical operations are for.
Here is the explanation of arithmetic, comparison and logical operations.
Arithmetic Operations
Arithmetic operations perform the basic mathematical functions. They are used for the calculations and data processing. We can use them to manipulate the numeric values in programming. Common arithmetic operators are given below:
Addition: Addition operator
+
is used to add values. For example:5+3=8
.Subtraction: This operator is used to subtract the second value from the first value. For example:
10-6=4
. This is how this operator works.Multiplication: This is another important operator and it is used to multiply two values. For example:
4 * 7 = 28
. We can multiply any values with this operator.Division: This division
/
operator is used to dived first value by the second value. For example:20 / 5 = 4
So in this way division operator helps us to divide the first value by the second.Modulus: This operator is represented by
%
and it returns the remainder of dividing two values. For example:10 % 3
returns 1 as remainder because it divides 10 by 3 and it leaves 1 as remainder.
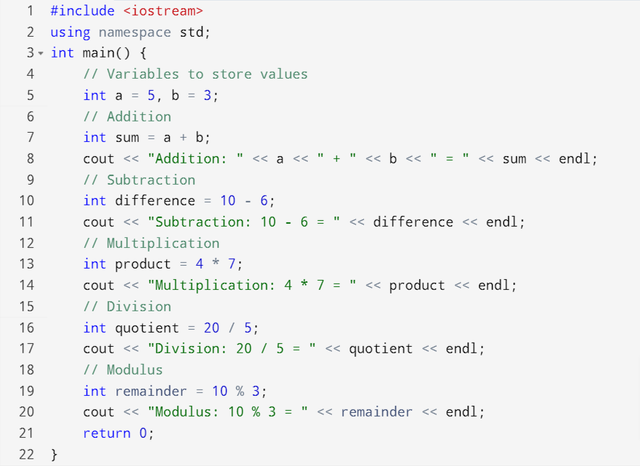
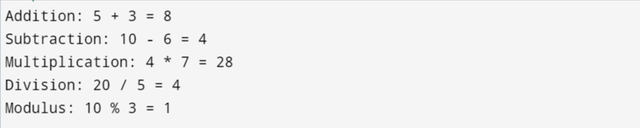
It is how arithmetic operations are carried out in programming.
Comparison Operations
Comparison operations are used to compare two values. These operators return a boolen result in the form of true or false. They help us in writing the conditions for the decision making. We can use them in if
statements and to control the program. Common comparison operators are given below:
Equal
This comparison operator checks if the 2 values are equal to each other or not. Its symbol is =
.
Example
If(a == b){
return true}
else false
Here if we take values of a = 5 and b = 5 then it will return true otherwise if we take values a = 5 and b = 3 then it will return false.
Not Equal to
This operator checks if two values are not equal. The symbol of this operator is !=
.
Example:
If(a != b){
return true}
else false
Here if we take values of a = 5 and b = 3 then it will return true otherwise if we take values a = 5 and b = 5 then it will return false.
Greater than
This operator checks if the first value is greater than the second value. its symbol is >
.
Example:
If(a>b){
return true}
else false
Here if we take values of a = 5 and b = 3 then it will return true otherwise if we take values a = 3 and b = 5 then it will return false.
Less than
This operators checks if the first value is less than the second value. Its symbol is <
.
Example:
If(a<b){
return true}
else false
Here if we take values of a = 3 and b = 5 then it will return true otherwise if we take values a = 9 and b = 5 then it will return false.
Greater than or equal to
This operator checks if the first value is greater than or equal to the second value. It will return true whether the first value is equal to second or greater than second. Its symbol is >=
.
Example:
If(a>=b){
return true}
else false
Here if we take values of a = 8 and b = 5 or a=5 and b = 5 then it will return true otherwise if we take values a = 2 and b = 5 then it will return false.
Less than or equal to
This operator checks if the first value is less than or equal to the second value. It will return true whether the first value is equal to second or less than second. Its symbol is <=
.
Example:
If(a<=b){
return true}
else false
Here if we take values of a = 3 and b = 5 or a=5 and b = 5 then it will return true otherwise if we take values a = 7 and b = 5 then it will return false.
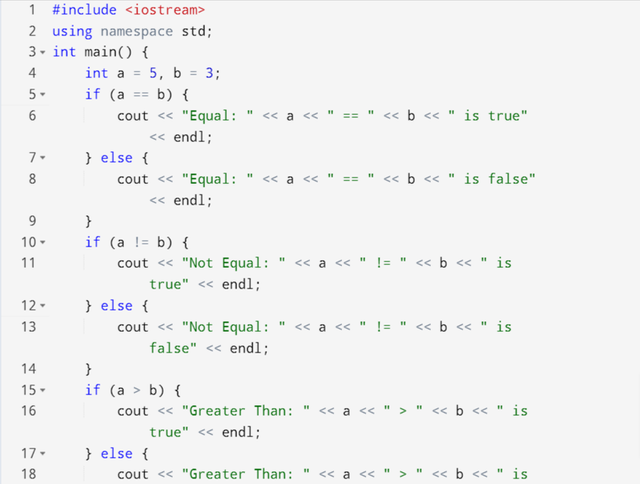
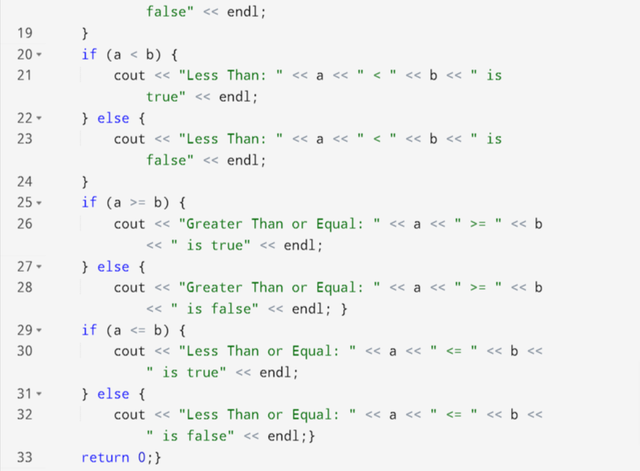
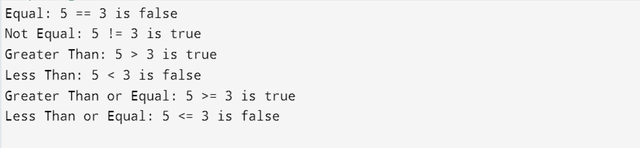
Here you can see the working of all the comparison operators I have combined all of them in a single example to show how comparison operations are carried out in programming. I have take a = 5 and b = 3.
Logical Operations
These operations are used to combine or modify the boolen expressions. They allow us to use multiple conditions at once. We can check more than one conditions in programming using these logical operators. They play a key role in the decision making. Commonly used logical operators are given below:
AND Operator
This operator allows us to check two conditions at once and it returns true
when both the conditions are true other false. I will further explain it using a truth table chart below:
A | B | A && B |
---|---|---|
0 | 0 | False |
0 | 1 | False |
1 | 0 | False |
1 | 1 | True |
From the above table we can see that if at least one value is true then it will return true otherwise false. In the above table 0 is representing false and 1 is indicating true.
Example:
if(a > b) && (c<d) {
return true;}
{else return false;}
So in this way the logical operators check different conditions at once and return true or false value accordingly. (5 > 3) && (4 < 6)
returns true because both conditions are true.
OR Operator
This operator allows us to check two conditions at once and it returns true
when both the conditions are true other false. I will further explain it using a truth table chart below:
A | B | A OR B |
---|---|---|
0 | 0 | False |
0 | 1 | True |
1 | 0 | True |
1 | 1 | True |
From the above table we can see that if both the values are true then it will return true otherwise false. In the above table 0 is representing false and 1 is indicating true.
Example:
if(a > b) || (c<d) {
return true;}
{else return false;}
So in this way the logical operators check different conditions at once and return true or false value accordingly. (5 > 7) || (3 < 9)
returns true because the second condition is true.
NOT Operator
This operator allows us to reverse the value of a boolean expression. It turns true
into false
and vie versa.
A | !A |
---|---|
True | False |
False | True |
From the above table you can get more easy understanding how it reverses the expression.
Example:
!(5 > 3) returns false because 5 > 3 is true and NOT negates it and converting it to say (5<3) which is not true so this is the reason it is returning false value.
This is how we can use logical operators work by checking multiple conditions at once. So whenever we need to check multiple conditions we can use logical operators with other operations.
Make a program that asks the user for 2 numbers and evaluates if both numbers are the same
Before writing any program it is essential or you can say helpful thing to prepare algorithm for that program. So here is an algorithm to represent the steps of program:
Here's a straightforward algorithm for the C++ program that asks for two numbers and checks if they are the same:
- Start
- Declare two integer variables:
num1
andnum2
. - Declare a Boolean variable:
areSame
. - Output Ask the user for the first number "Enter the first number:".
- Input Get first number from the user and store it in
num1
. - Output Ask the user for the second number "Enter the second number:".
- Input Get the second number from the user and store it in
num2
. - Assign the result of the comparison
(num1 == num2)
to the Boolean variableareSame
. - Output Display if the numbers are same, "Are the numbers the same?" followed by the Boolean result stored in
areSame
. Useboolalpha
to ensure the Boolean value is displayed as "true" or "false". - End
This algorithm provides a clear step-by-step method to input two numbers, compare them, and output whether they are the same using a simple Boolean expression.
I am using C++ language to write that program so that everyone can understand it easily.
#include < iostream >
using namespace std;
int main() {
int num1, num2;
bool areSame;
cout << "Enter the first number: ";
cin >> num1;
cout << "Enter the second number: ";
cin >> num2;
//Compare the numbers and store the result in a Boolean variable
areSame = (num1 == num2);
cout << "Are the numbers the same? " << boolalpha << areSame << endl;
return 0;
}
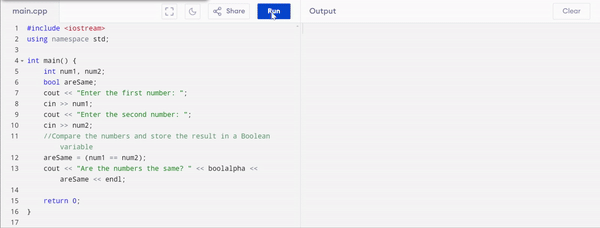
So it is the program which asks the user to input two numbers and then stores the numbers in respective variables after that it stores the result in the bool variable and returns if the 2 numbers are same or not. Again I want to mention that I have used boolalpha
to show the result in the form of true or false otherwise it will show 1
for the true value and similarly 0
for the false value.
Transform the following mathematical expressions into arithmetic expressions on your computer
Conversion of the mathematical expressions into arithmetic expressions in computer are given below:
First Expression "Y"
Y = (8 - 2)*(5+4)
Simplification: (8 - 2)*(5+4) = 6 * 9 = 54, So X = 54
Second Expression "X"
X = (8/2) + (5/5)
Simplification: (8/2) + (5/5) = 4 + 1 = 5 , so X = 5
Third Expression "Z"
Z = ((4 + 8) * 3) / (2 * 3)
Simplification: ((4 + 8) * 3) / (2 * 3) = (12 * 3) / 6 = 36 / 6 = 6 , so Z = 6
Evaluate if the results of the 3 operations are greater than or equal to 0 (>=) and show it on the screen.
#include < iostream >
using namespace std;
int main() {
int Y = (8 - 2) * (5+4); // Y = 54
double X = (8 / 2) + (5 / 5); // X = 5
double Z = ((4 + 8) * 3) / (2 * 3); // Z = 6
bool are_greater_zero = (X >= 0) && (Y >= 0) && (Z >= 0);
cout << "Y = " << Y << endl;
cout << "X = " << X << endl;
cout << "Z = " << Z << endl;
// Show if all results are greater than or equal to zero
cout << "Are all results greater than or equal to zero? " << boolalpha << are_greater_zero << endl;
return 0;
}
Here in the above multimedia you can see the working of the program which calculates all the given expressions and then it shows if all the 3 results are greater than or equal to. According to the given expression and values the program has calculated the exact results and as all the results are non negative numbers then it is showing that all the results are greater than equal to 0 as true. The use of the boolalpha
is returning true otherwise the program will return 1
which also represents the true value. I have used C++ language to write all the required programs.
Disclaimer: I have used Programiz C++ compiler whichis an online compiler and it offers compilation of many languages. And the recorded videos are also from that compiler.
I invite @fombae, @event-horizon and @suboohi to participate in this contest to learn some programming.
TEAM 5
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
This post has been upvoted/supported by Team 7 via @httr4life. Our team supports content that adds to the community.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit