Hello everyone! Today I am here to participate in the basic programming course by @alejos7ven. It is about learning the basics programming specifically conditional statements. If you want to join then:
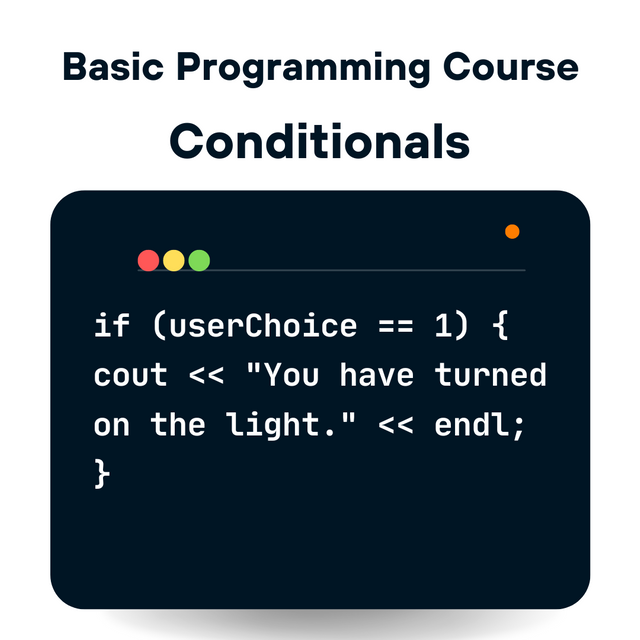.png)
Describe your understanding of conditional structures in programming and mention 2 situations of everyday life where you could apply this concept.
In our daily life we make many conditions to perform specific actions. If our specified conditions become true then we perform the respective task. But what about the computer and other machines? How they understand the conditions and perform specific actions in the computer system? So the answer is simple, they use and understand conditions through programming and coding.
In programming we use conditional structures to instruct the machines. Conditional structures in programming are used to control the flow of the program. They help us to make the decisions based on the pre-defined conditions. They help us to execute only that part or block of code whose conditions are met.
If I say that the conditional structures are just like the police checkpoint then it will not be wrong. Because their working is also same, just like the police checkpoint who checks the people or transport and if they are passed their conditions then they are allowed to move next otherwise not. Similarly conditional statements work like the checkpoint and they check the code and instructs the code to behave accordingly.
Conditional structures check the conditions and they proceed the program according to the condition. They check the condition whether it is true or false. The most common forms to implement the conditional structures are if
, else
, and else
. These are the most common forms but we have another form as well which is swicth-case
statement in some programming languages. These enable the program to perform different actions based on the inputs or circumstances.
If
statement: It executes a specific block of code when a specified condition is true.Else if
statement: This checks new condition if the previous condition is false and then proceed the program accordingly.Else
statement: If all the defined conditions become false then this block of code is executed.
We also use logical operators in the conditional statements. The logical operators help us to check more than one conditions at once. It also helps to reduce the code redundancy while writing same code again and again to check the same thing for different variables. These logical operators are ==
, >
, <
and ||
and we use them with conditions according to the nature of the condition check.
Everyday Life Examples
I have added two conditions from my everyday life here which are given below:
Deciding Whether to Exercise Based on Energy Level
- Condition:
if
I feel fresh and energetic I go out for a walk. - Else:
If
I am tired then I will decide to take rest.
This is similar to an if else
statement where energy level indicates my decision to either work out or to take rest.
C++ Code:
#include < iostream >
using namespace std;
int main() {
// Declare a variable to represent energy level (1: energetic, 0: tired)
int energyLevel;
cout << "Enter your energy level (1 for energetic, 0 for tired): ";
cin >> energyLevel;
// Conditional structure to decide based on energy level
if (energyLevel == 1) {
cout << "You feel energetic! Time to workout!" << endl;
} else {
cout << "You feel tired. Better rest or do some light activity." << endl;
}
return 0;
}
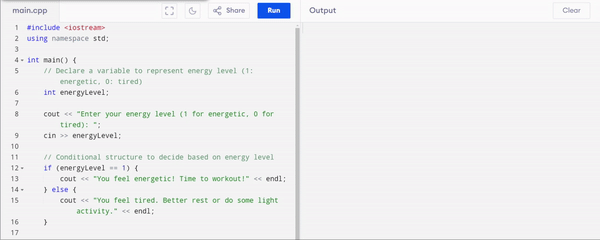
Here I have used 0 and 1 to check the conditions. I have set the condition on 1 that I am energetic and 0 for I am tired. So when I am selecting 1 it is executing the block of code defined in 1 and when I am choosing 0 it is executing the block of code defined based on 0.
Planning Transportation Based on Weather
- Condition: If the weather is good I will ride a bike to work.
- Else: If the weather is bad such as raining I will take public transportation.
This is like an if-else
structure where my choice of transportation is based on weather conditions.
C++ Code:
#include < iostream >
using namespace std;
int main() {
// Declare a variable to represent weather (1: good, 0: bad)
int weather;
cout << "Enter the weather condition (1 for good, 0 for bad): ";
cin >> weather;
// Conditional structure to decide transportation based on weather
if (weather == 1) {
cout << "The weather is good! Ride your bike to work!" << endl;
} else {
cout << "The weather is bad. Better take public transportation." << endl;
}
return 0;
}
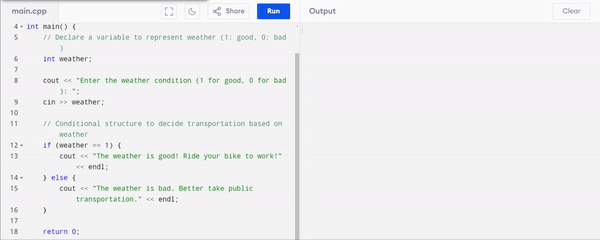.gif)
The above code has two cases to check the condition. I have set 1 for the good weather condition and similarly 0 for the bad weather condition. So when I am inputting 1 it is showing me block of code related to 1 and similarly for the 0.
Create a program that tells the user "Welcome to the room What do you want to do?", if the user writes 1 then it shows a message that says "you have turned on the light", if the user writes 2 then it shows a message that says "you have left the room". Use conditionals.
Conditional statements are very important in pour daily programming tasks. The requested program is based on the conditions.
Algorithm to write the requested program
Here is the algorithm for the C++ program that asks the user for input and shows appropriate messages based on their choice:
- Start
- Display the message "Welcome to the room. What do you want to do?"
- Display the options after entering the room:
- "Enter 1 to turn on the light"
- "Enter 2 to leave the room"
- Read the user input and store it in a variable such as
userChoice
. - Then we will check the value of
userChoice
:- If
userChoice == 1
the program will display "You have turned on the light." - Else If
userChoice == 2
the program will display "You have left the room." - Else the program will display "Invalid choice. Please enter 1 or 2."
- If
- End
Working
- Input: The user enters a choice (either
1
or2
). - Condition Check:
- If the input is
1
, the light is turned on. - If the input is
2
, the user leaves the room. - If the input is invalid, an error message is shown.
- If the input is
- Output: The program outputs the relevant message based on the user's choice.
C++ Program:
#include < iostream >
using namespace std;
int main() {
int userChoice;
// Welcome message
cout << "Welcome to the room. What do you want to do?" << endl;
cout << "Enter 1 to turn on the light, or 2 to leave the room: "<<endl;
cin >> userChoice;
// Conditional structure to respond based on user input
if (userChoice == 1) {
cout << "You have turned on the light." << endl;
} else if (userChoice == 2) {
cout << "You have left the room." << endl;
} else {
cout << "Invalid choice. Please enter 1 or 2." << endl;
}
return 0;
}
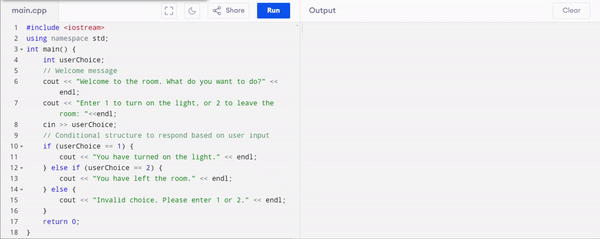.gif)
Explanation
- The program first greets the user and asks what they want to do.
- It provides two options:
- Turning on the light (1).
- Leaving the room (2).
- An
if-else if
structure checks the input of the user and based on the choice it prints the corresponding message. - If the user enters something other than 1 or 2, the program prints an error message.
Create a program that asks the user for 4 different ratings, calculate the average and if it is greater than 70 it shows a message saying that the section passed, if not, it shows a message saying that the section can improve.
Here’s the algorithm for the program that asks the user for 4 different ratings, calculates the average, and checks if the section passed or can improve:
Algorithm
- Start
- Declare variables:
rating1
,rating2
,rating3
,rating4
(for storing ratings) andaverage
(for storing the calculated average). - Ask the user to enter
rating1
and read the input. - Ask the user to enter
rating2
and read the input. - Ask the user to enter
rating3
and read the input. - Ask the user to enter
rating4
and read the input. - Calculate the average:
average = (rating1 + rating2 + rating3 + rating4) / 4.0
- Display the calculated
average
. - If the
average
is greater than 70:- Display the message: "The section passed!"
- Else:
- Display the message: "The section can improve."
End
Working
- Input: The user inputs 4 ratings as integers.
- Calculation: The program calculates the average of these ratings.
- Condition Check:
- If the average is greater than 70 it outputs that the section passed.
- Otherwise it indicates the section can improve.
- Output: Displays the average and whether the section passed or can improve based on the average score.
This algorithm ensures that the program processes the input correctly and provides meaningful feedback.
C++ Code:
#include < iostream >
using namespace std;
int main() {
// Variables to store the ratings
int rating1, rating2, rating3, rating4;
double average;
// Ask the user for 4 different ratings
cout << "Enter rating 1: ";
cin >> rating1;
cout << "Enter rating 2: ";
cin >> rating2;
cout << "Enter rating 3: ";
cin >> rating3;
cout << "Enter rating 4: ";
cin >> rating4;
// Calculate the average of the ratings
average = (rating1 + rating2 + rating3 + rating4) / 4.0;
// Display the average
cout << "Average rating: " << average << endl;
// Conditional to check if the average is greater than 70
if (average > 70) {
cout << "The section passed!" << endl;
} else {
cout << "The section can improve." << endl;
}
return 0;
}
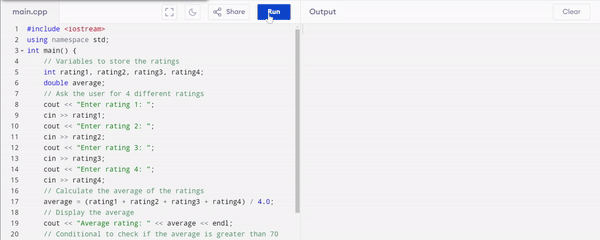.gif)
Average rating more than 70: In the above program when I am entering 4 ratings the program calculates the average of the rating. If the average rating is more than 70 it displays The section passed! and program ends here.
Average rating less than 70: According to the above program when I am entering 4 ratings the program then calculates the average ratings. If the average rating is less than 70 then displays The section can improve. In this way the execution of the conditional program ends.
In this way we can use conditional structures in programming and we can perform different works based on the pre-defined conditions.
Disclaimer: I used an online compiler Programiz and all the video recordings have been captured from that compiler.
X Promotion: https://x.com/stylishtiger3/status/1841455771344654725
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Your post has been rewarded by the Seven Team.
Support partner witnesses
We are the hope!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit