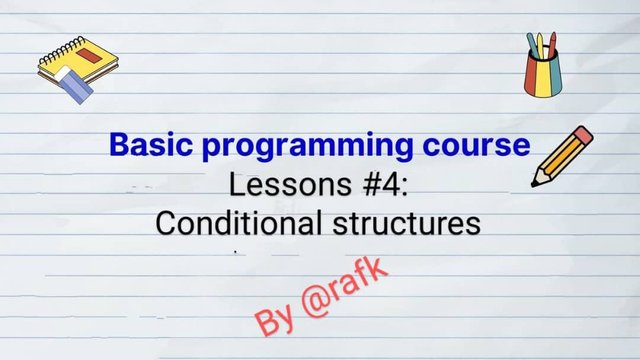
Conditional Structures
Hello everyone, welcome to this weeks engagement challenge. i'm excited to be taking on yet another course in programming, this time on Conditional structures. without wasting much time i will start with the task required.
Describe your understanding of conditional structures and mention 2 situations of everyday life where you could apply this concept.
Understanding Conditional Structures in Programming
Conditional structures refers to programming constructs which allow a program to toggle decisions with respect to certain conditions. They allow the program to execute different code blocks regardless on whether a specific condition is true or false. It flexibility is essential for creating rapid and responsive applications.
Common Conditional Structures
If statement: The program is task to executes a block of code if a specific condition is statisfied(True).
If-else statement: The program Executes one block of code if a condition is true, if not, loowed by another block of code if the codition is false.
Else-if statement: The program is tasked to Allows for multiple conditions to be checked, and the most appropriate block of code is executed based on the first true condition in course.
Switch statement: Efficiently compares a value against multiple cases and executes the corresponding code block.
Everyday Life Applications
Traffic Light Control: ON a given day, the traffic light system can make use conditional structures to determine when to change the lights based on vehicle sensors and timers. For instance, take for the case where there are no cars waiting in a given direction, the traffic light can stay red for that direction untill vechicles begin to approach.
We could also have simple conditions like weather control, where the program can be able to tell us the weather conditons and the type of cloths to put on.
program TemperatureRecommendation
var temperature: real;
begin
writeln("Enter the temperature:");
readln(temperature);
if temperature > 30 then
writeln("It's hot! Wear light clothing.")
else if temperature < 20 then
writeln("It's cold. Wear a pullover.")
else
writeln("The temperature is comfortable. Dress as you like.")
end.
Create a program that tells the user "Welcome to the room What do you want to do?", if the user chooses1 it display a message that says "you have turned on the light", if the user choose 2 it displays a message which says "you have left the room". Make use conditionals.
Explanation:
Here’s how the conditionals in the pseudocode will work when the program is executed:
1- Si option = "1" Entonces:
When the program reaches this line, it checks if the user entered "1".
If this condition is true that is the user input 1
, the program will execute the code inside this block, displaying the message "You have turned on the light."
2- SiNo:
If the first condition is false meaning the user didn't choose "1"
, the program skips the first block and moves on to this part, which acts like "else"
in many programming languages.
3- Si option = "2" Entonces
:
Now, it checks if the user entered "2"
.
If this condition is true that is the user chose "2"
, the program will execute the code in this block, displaying the message "You have left the room."
4- SiNo:
If both the previous conditions are false, the user didn't choose "1" or "2"
, the program moves to this block.
5- Escribir "Invalid input...":
This block is executed when neither "1" nor "2"
was entered, showing an error message.
Create a program that asks the user for 4 different ratings, calculate the average and if it is greater than 70 it shows a message saying that the section passed, if not, it shows a message saying that the section can improve.
Explanation:
1- Definir:
The variables math, physics, chemistry, biology, and average are declared as real numbers to handle fractional values.
2- Escribir and Leer:
These lines prompt the user to enter their scores for each subject: Mathematics, Physics, Chemistry, and Biology.
3- average
= (math + physics + chemistry + biology) / 4: The scores are added together and divided by 4 to calculate the average.
4- Escribir "The average score is: "
, average: This displays the calculated average.
5- Si average > 70 Entonces:
If the average is greater than 70, the message "Section Passed!" is displayed.
6- SiNo:
If the average is less than or equal to 70, the message "The section can improve." is displayed.
The program checks if the average score meets the criteria and gives feedback accordingly
Rounding up with this amazing lesson. i will like to invite the following persons to participate with me. @Wirngo, @chant and @sinmonnwigwe
Best regards: @rafk
Your post has been rewarded by the Seven Team.
Support partner witnesses
We are the hope!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit