Hello there,
In this post I am going to work on the homework from Basic programming course: Lesson #5 Control structures. Part 2. Cycles. by @alejos7ven
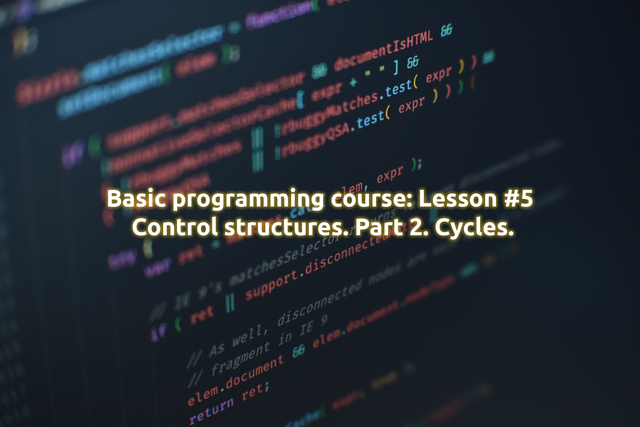
Image used from Pixabay
Explain the cycles in detail. What are they and how are they used? What is the difference between the While and Do-While cycle?
Cycles also known as loops are used in programming to control "chunks"/blocks of code that need to run repeatedly based on conditions. These loops are used to run a task multiple times, as an example going through each element of a list, array, matrix etc.
What loops exist:
While loop
WHILE condition
// Code to execute
END WHILE
The WHILE loop executes the code as long as the condition is TRUE, if the condition becomes FALSE the code inside the WHILE LOOP is skipped. In this case the condition is always checked before executing the code.
Do-While loop
DO
// Code to execute
WHILE condition
In a Do-While loop the code is being executed as long as the condition is TRUE, the difference here is that the code is ran at least one time before it reaches the condition to check it.
For loop
FOR initialization TO condition STEP update
// Code to execute
END FOR
It iterates a specific number of times, based on the initialization, condition, and update expressions. The for loop can be used in multiple ways, using a break to stop the execution at a given time, you can use it without and update expression or without a condition, you can use a for loop inside another for loop, it all depends on the programming language you use and it's rules and restrictions.
Now let's see the difference between While and Do-While cycles:
While vs Do-While
In a While loop condition is checked before the loop body, while in a Do-While the condition is checked after the loop body.
In a While loop the code inside the loop might not even be executed if the condition is false, while in a Do-While the code is executed at least one time before the condition is being checked.
Investigate how the Switch-case structure is used and give us an example.
A switch-case structure is a control flow structure used in programming that allows a variable to be tested against different case values. It's an alternative for a big if-else. It's purpose is to make the code clear while working with many conditional checks.
Let's analyze it, the Switch structure starts with an expression, if the expression is TRUE we get
SWITCH (expression)
CASE value1:
// Code to execute if expression equals value1
BREAK
CASE value2:
// Code to execute if expression equals value2
BREAK
...
CASE valueN:
// Code to execute if expression equals valueN
BREAK
DEFAULT:
// Code to execute if expression doesn't match any case
END SWITCH
SWITCH (expression) -> The expression is being evaluated
CASE value1, value2, ... valueN: -> The expression is being compared to (value1, value2, ..., valueN).
If the expression matches a case (e.g., value1), the corresponding block of code is executed.
BREAK: After executing the matched case's code, the break statement prevents further cases from being evaluated.
DEFAULT: If none of the cases match the expression, the code in the DEFAULT section is executed. This acts as a fallback.
This structure is used for cleanly handling multiple possible values of a single variable.
Example:
SWITCH (gear)
CASE gear1:
PRINT "Gearbox selector set for the first gear";
gear_selector = 1;
BREAK
CASE gear2:
PRINT "Gearbox selector set for the second gear";
gear_selector = 2;
BREAK
...
CASE gearR:
PRINT "Gearbox selector set for the Reverse gear";
gear_selector = -1;
BREAK
DEFAULT:
PRINT "Unknown Gearbox value";
BREAK
END SWITCH
Explain what the following code does:
Algorithm switchcase
Define op, n, ns, a As Integer;
Define exit As Logic;
exit = False;
a=0;
Repeat
Print "Select an option:";
Print "1. Sum numbers.";
Print "2. Show results."
Print "3. End program.";
Read op;
Switch op Do
case 1:
Print "How much nums do you want to sum?";
Read n;
For i from 1 to n Do
Print "Enter a number: ";
Read ns;
a = a + ns;
EndFor
Print "Completed! Press any button to continue";
Wait Key;
Clear Screen;
case 2:
Print "This is the current result: " a;
Print "Press any button to continue.";
Wait Key;
Clear Screen;
case 3:
Clear Screen;
Print "Bye =)";
exit = True;
Default:
Print "Invalid option.";
Print "Press any button to continue";
Wait Key;
Clear Screen;
EndSwitch
Until exit
EndAlgorithm
Now let's have an overview of the Algorithm above:
The program has a Loop with a Switch case statement inside and the only way to escape the loop is through our exit variable, only when this variable gets the value True we end the loop.
It starts with a declaration of multiple integer variables op, n, ns, a
, exit
as boolean, after that the exit and a variable are given a value, False and 0 in this case.
We have a Repeat until exit is True that contains some Prints and the Switch case. The user is being presented with a few messages, being presented to select and option and the 3 options that he can pick from, the option being read using the OP variable.
Once the user has selected an option and the OP variable has a value, this value is being used in the Switch statement where the compiler is going to compare our OP value with the available cases. If the user enters one of the 3 values we have in our cases (1,2 or 3) the code in that Case is going to be executed. If the value presented by the user is not (1,2 or 3) we get the Default case.
Let's see what code is being executed in each case:
case1:
If the value of OP is one, in the switch statement we are executing the code in case1 which does the following:
Asks the user "How much nums do you want to sum?" after that registers the value sent by the user in the N variable.
With the value of N known now the code runs a loop from 1 to N and asks the user for a number that in the end gets added to variable A.
Let's run an example here to see clearly how it works:
How much nums do you want to sum?
We enter 5, n becomes 5.
The for starts from 1 to 5, we read the value from the user and add it to a:
- n = 1 -> read 17
- a = 0 + 17 -> a = 17
- n = 2 -> read 11
- a = 17 + 11 -> a = 28
- n = 3 -> read 5
- a = 28 + 5 -> a = 33
- n = 4 -> read 3
- a = 33 + 3 -> a = 36
- n = 5 -> read 12
- a = 36 + 12 -> a = 48
We get out of the for loop, the user is presented with the message "Completed! Press any button to continue". The program waits for a Key to be pressed and after the a key is being pressed the console is being cleared.
Because this case doesn't have an Exit = True we are still in the loop and the user will be asked again to present an option and get into another case (or the same one if he wants).
case2:
The second case is much simpler, the user is presented with a message "This is the current result:" and the value of variable a, while again we have the user asked to press any key, once pressed screen is cleared and we are back in the loop because we have no Exit = True.
case3:
In case 3, we have the screen cleared, a message with "Bye =)" appears and exit becomes true and the program ends because we get out of the loop.
Default
The user gets the message "Invalid option" and the same as seen in previous cases "Press any button to continue", waits for a key after and clears screen.
Write a program in pseudo-code that asks the user for a number greater than 10 (Do-While), then add all the numbers with an accumulator (While) Example: The user enters 15. The program adds up to 1+2+3+4+5+6+7+8+9+10+11+12+13+14+15.
START
DECLARE number, sum, counter AS INTEGER
SET sum TO 0
SET counter TO 1
DO
PRINT "Enter a number greater than 10: "
INPUT number
WHILE number <= 10
WHILE counter <= number
sum = sum + counter
counter = counter + 1
END WHILE
PRINT "The sum is ", sum
END
We start by declaring our variables, number, sum, counter
all as integers, we set the sum to 0 and counter to 1. We add the DO-WHILE prompting the user to enter a number greater than 10, we save this number in the variable, all this while the number is smaller or equal to 10. If we enter anything below 10 the number will be saved over it's previous value in the number variable.
- Enter 5 -> number = 5
- Enter 7 -> number loses the 5 value and gets the new one -> number = 7
- Enter 3 -> number loses the 7 value and gets a new one -> number = 3
- Enter 15 -> number loses the value 3 and gets a new one -> number = 15
- 15 is greater than 10, we get out of the loop
And now for our WHILE, we check our counter if it's value is smaller or equal to our number (15), if it isn't we get inside the loop and to the math.
Counter is 1, 1<=15? Yes, we go inside the loop.
Our sum is 0 and add the counter 1, sum becomes 1.
Counter is 1 and we add 1, counter becomes 2.
Counter is 2, 2<=15? Yes, we are still inside the loop
Our sum is 1 and add the counter 2, sum becomes 3.
Counter is 2 and we add 1, counter becomes 3.
Counter is 3, 3<=15? Yes, we are still inside the loop
Our sum is 3 and add the counter 3, sum becomes 6.
Counter is 3 and we add 1, counter becomes 4.
And we do this until our counter reaches a value of 16, in which case the condition will not be met and we get out of the WHILE loop.
All that needs to be done after is print the sum of the numbers.
That was it for this homework, thank you for stopping by, wishing you a great day!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit