HERE IN THIS .Net Tutorial WE WILL CREATE FEES SLIPS USING ITEXTSHARP IN ASP.NET AND C#
Comment Me IF You Want Code.
Step 1 :-
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="WebApplication15.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.auto-style1 {
width: 38%;
height: 84px;
}
.auto-style2 {
width: 145px;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div>
<table class="auto-style1">
<tr>
<td class="auto-style2">
<asp:Label ID="Label1" runat="server" Text="Fees Slips"></asp:Label>
</td>
<td> </td>
<td> </td>
</tr>
<tr>
<td class="auto-style2">
<asp:Label ID="Label2" runat="server" Text="Student Name :- "></asp:Label>
</td>
<td>
<asp:TextBox ID="TextBox1" runat="server" Width="342px"></asp:TextBox>
</td>
<td> </td>
</tr>
<tr>
<td class="auto-style2">
<asp:Label ID="Label3" runat="server" Text="Address :-"></asp:Label>
</td>
<td>
<asp:TextBox ID="TextBox2" runat="server" Height="50px" TextMode="MultiLine" Width="340px"></asp:TextBox>
</td>
<td> </td>
</tr>
<tr>
<td class="auto-style2">
<asp:Label ID="Label4" runat="server" Text="Payment Type :-"></asp:Label>
</td>
<td>
<asp:DropDownList ID="DropDownList1" runat="server">
<asp:ListItem>Select Type ---</asp:ListItem>
<asp:ListItem>Cash</asp:ListItem>
<asp:ListItem>Cheque</asp:ListItem>
</asp:DropDownList>
</td>
<td> </td>
</tr>
<tr>
<td class="auto-style2">
<asp:Label ID="Label5" runat="server" Text="Date :-"></asp:Label>
</td>
<td>
<asp:TextBox ID="TextBox3" runat="server" TextMode="Date"></asp:TextBox>
</td>
<td> </td>
</tr>
<tr>
<td class="auto-style2">
<asp:Label ID="Label6" runat="server" Text="Course :-"></asp:Label>
</td>
<td>
<asp:TextBox ID="TextBox4" runat="server"></asp:TextBox>
</td>
<td> </td>
</tr>
<tr>
<td class="auto-style2">
<asp:Label ID="Label7" runat="server" Text="Fees :-"></asp:Label>
</td>
<td>
<asp:TextBox ID="TextBox5" runat="server"></asp:TextBox>
</td>
<td> </td>
</tr>
<tr>
<td class="auto-style2"> </td>
<td> </td>
<td> </td>
</tr>
<tr>
<td class="auto-style2"> </td>
<td>
<asp:Button ID="Button1" runat="server" OnClick="Button1_Click" Text="Submit" />
</td>
<td> </td>
</tr>
</table>
</div>
</form>
</body>
</html>
Step 2:-
using iTextSharp.text;
using iTextSharp.text.html.simpleparser;
using iTextSharp.text.pdf;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace WebApplication15
{
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
public static string ConvertNumbertoWords(int number)
{
if (number == 0)
return "ZERO";
if (number < 0)
return "minus " + ConvertNumbertoWords(Math.Abs(number));
string words = "";
//if ((number / 1000000000) > 0)
//{
// words += ConvertNumbertoWords(number / 1000000000) + " Billion ";
// number %= 1000000000;
//}
if ((number / 100000000) > 0 || (number / 10000000) > 0)
{
if ((number / 10000000) > 0)
{
words += ConvertNumbertoWords(number / 10000000) + " CRORE ";
number %= 10000000;
}
if ((number / 100000000) > 0)
{
words += ConvertNumbertoWords(number / 100000000) + " CRORE ";
number %= 100000000;
}
}
if ((number / 1000000) > 0 || (number / 100000) > 0)
{
if ((number / 100000) > 0)
{
words += ConvertNumbertoWords(number / 100000) + " LAKH ";
number %= 100000;
}
if ((number / 1000000) > 0)
{
words += ConvertNumbertoWords(number / 1000000) + " LAKH ";
number %= 1000000;
}
}
if ((number / 1000) > 0)
{
words += ConvertNumbertoWords(number / 1000) + " THOUSAND ";
number %= 1000;
}
if ((number / 100) > 0)
{
words += ConvertNumbertoWords(number / 100) + " HUNDRED ";
number %= 100;
}
if (number > 0)
{
if (words != "")
words += "AND ";
var unitsMap = new[] { "ZERO", "ONE", "TWO", "THREE", "FOUR", "FIVE", "SIX", "SEVEN", "EIGHT", "NINE", "TEN", "ELEVEN", "TWELVE",
"THIRTEEN", "FOURTEEN","FIFTEEN", "SIXTEEN", "SEVENTEEN", "EIGHTEEN", "NINETEEN" };
var tensMap = new[] { "ZERO", "TEN", "TWENTY", "THIRTY", "FORTY", "FIFTY", "SIXTY", "SEVENTY", "EIGHTY", "NINETY" };
if (number < 20)
words += unitsMap[number];
else
{
words += tensMap[number / 10];
if ((number % 10) > 0)
words += " " + unitsMap[number % 10];
}
}
return words;
}
protected void Button1_Click(object sender, EventArgs e)
{
string img = Server.MapPath("~/Img/sign.jpg");
string logo = Server.MapPath("~/Img/logo.jpg");
string word = ConvertNumbertoWords(Convert.ToInt32(Math.Round(Convert.ToDecimal(TextBox5.Text))));
StringBuilder sb = new StringBuilder();
sb.Append("<header class='clearfix'>");
sb.Append("<img align='center' src='"+logo+"' width='70px' height='40px' />");
sb.Append("<p align='center' style='font-size:8px'><b>2nd Flr., B.E.S.T Commercial Complex,Andheri West,Mumbai, India - 400053.</b></p>");
sb.Append("<br>");
sb.Append("<b><p align='center'>Fees Slip</p></b>");
sb.Append("<br>");
sb.Append("</header>");
sb.Append("<main>");
sb.Append("<p align='left'><b>Student Name : Mr/Mrs./Miss :"+TextBox1.Text+".</b></p>");
sb.Append("<br>");
sb.Append("<p align='left'><b>Addresses :" + TextBox2.Text + ".</b></p>");
sb.Append("<br>");
sb.Append("<p align='left'><b>Payment Type :" + DropDownList1.SelectedItem.Text + ".</b></p>");
sb.Append("<br>");
sb.Append("<p align='left'><b>Date :" + TextBox3.Text + ".</b></p>");
sb.Append("<br>");
sb.Append("<br>");
sb.Append("<br>");
sb.Append("<table border=1 align='center'>");
sb.Append("<thead>");
sb.Append("<tr>");
sb.Append("<th style='width:3px;'>Fees No</th>");
sb.Append("<th style='width:20px;'>Course Type</th>");
sb.Append("<th style='width:10px;'>Amount Paid</th>");
sb.Append("</tr>");
sb.Append("</thead>");
sb.Append("<tbody>");
sb.Append("<tr>");
sb.Append("<td>1</td>");
sb.Append("<td>"+ TextBox4.Text + "</td>");
sb.Append("<td>Rs. " + TextBox5.Text + "</td>");
sb.Append("</tr>");
sb.Append("</tbody>");
sb.Append("</table>");
sb.Append("</main>");
sb.Append("<br>");
sb.Append("<p align='left'><b>Amount In Word : RUPEE " + word + " ONLY.</b></p>");
sb.Append("<br>");
sb.Append("<br>");
sb.Append("<img align='right' src='" + img + "' width='60px' height='40px' />");
sb.Append("<br>");
sb.Append("<p align='right'><b>Signature</b></p>");
sb.Append("<br>");
sb.Append("<footer>");
sb.Append("Note :- Fees Once Paid Will Not Be Refundable Under Any Circumstances.");
sb.Append("<br>");
sb.Append("..............................................................................................................................");
sb.Append("</footer>");
StringReader sr = new StringReader(sb.ToString());
Document pdfDoc = new Document(PageSize.A4, 10f, 10f, 10f, 0f);
HTMLWorker htmlparser = new HTMLWorker(pdfDoc);
string FileName = "File_" + DateTime.Now.ToString("ddMMyyyyhhmmss") + ".pdf";
using (MemoryStream memoryStream = new MemoryStream())
{
PdfWriter writer = PdfWriter.GetInstance(pdfDoc,memoryStream);
pdfDoc.Open();
htmlparser.Parse(sr);
pdfDoc.Close();
byte[] bytes = memoryStream.ToArray();
System.IO.File.WriteAllBytes(Server.MapPath("~/downloads/") + FileName, bytes);
memoryStream.Close();
Response.Clear();
Response.ContentType = "application/pdf";
Response.AddHeader("Content-Disposition","attachment; filename="+FileName);
Response.Buffer = true;
Response.Cache.SetCacheability(HttpCacheability.NoCache);
Response.BinaryWrite(bytes);
Response.Flush();
Response.Close();
Response.End();
}
}
}
}
If You Haven't Subscribers My Channel Than Please Subscribers It And Even Share It Link Of
My Channnel :- https://www.youtube.com/channel/UCdIDIGUh3rL9A_Yt_tbZmkg Please Go And Subscribers It
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
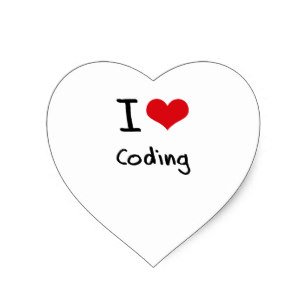
Reply !stop to disable the comment. Thanks!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
If You Haven't Subscribers My Channel Than Please Subscribers It And Even Share It Link Of
My Channnel :- https://www.youtube.com/channel/UCdIDIGUh3rL9A_Yt_tbZmkg Please Go And Subscribers It
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit