Hello everyone! I hope you will be good. Today I am here to participate in the contest of @kouba01 about Getting Started with Java and Eclipse. It is really an interesting and knowledgeable contest. There is a lot to explore. If you want to join then:
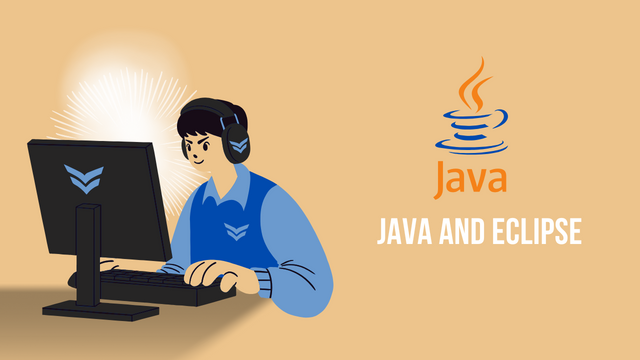
Write a detailed explanation of the differences between JDK, JRE, and JVM by including their roles, functionalities, and interdependence.
JDK, JRE, and JVM Differences
Java is a platform-independent language. Its ecosystem is based on three core components which are JDK (Java Development Kit), JRE (Java Runtime Environment) and JVM (Java Virtual Machine). These three are very different but work together for the smooth development and execution of Java programs. Below is a detailed explanation of each:
1. JDK (Java Development Kit)
Role: Offers tools for Java program development.
Key Components:
- JRE (Java Runtime Environment): Guarantees the execution of Java programs.
- Java Compiler (
javac
): Translates Java source code into bytecode. - Development Tools: It comprises debuggers, profilers, and documentation tools such as
javadoc
.
Functionality:
- The JDK is necessary for developing Java applications.
- It packages libraries and tools for writing, compiling and debugging Java programs.
- Without JDK we cannot create Java applications.
2. JRE (Java Runtime Environment)
Role: Provides the runtime environment to execute Java programs.
Key Components:
- JVM (Java Virtual Machine) : Executes the bytecode.
- Core Libraries: Prewritten classes and functions for input/output, networking, and many more.
Functionality:
- JRE does not come with development tools such as a compiler.
- It is a container that offers all the libraries and the JVM to run a program.
- It is best suited for end-users who need to run Java applications.
3. JVM (Java Virtual Machine)
Role: Runs Java bytecode, thereby allowing platform independence.
Key Components:
- Class Loader: Loads compiled bytecode into memory.
- Bytecode Verifier: It checks that the code is following the rules of Java security and structure.
- Execution Engine: It executes the loaded bytecode by converting it into machine code (Just-In-Time Compilation).
Functionality:
- JVM is platform specific but ensures Java code runs the same way on any system.
- It manages memory and garbage collection.
- JVM's structure allows portability, security and runtime optimization.
Interdependence
- JDK contains JRE which contains JVM.
- JVM is the one that runs the code and JRE provides the libraries and environment to support the JVM.
- Developers use JDK to write and compile Java programs and end users use JRE to run these programs.
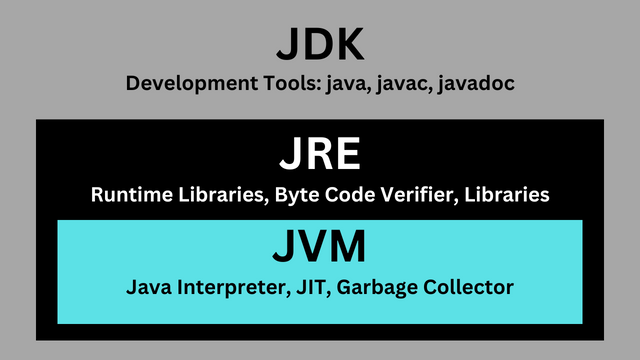
The above graphic illustration explains the differences between JDK, JRE, and JVM. It represents their layered structure and functionalities in a graphic way, and interdependence as explained in detail.
Install and Set Up Your Java Development Environment and provide step-by-step screenshots of the installation process, Eclipse configuration.
Here is a step by step guide to set up Java development environment, including the JDK and Eclipse IDE.
1. Installation of Java Development Kit (JDK)
Step 1: Download the JDK
- Visit the official Oracle JDK download page. We can also use an open-source version like OpenJDK.
- Here we need to choose the latest version of JDK for operating system (Windows, macOS, or Linux). I am using Windows so I will use windows version.
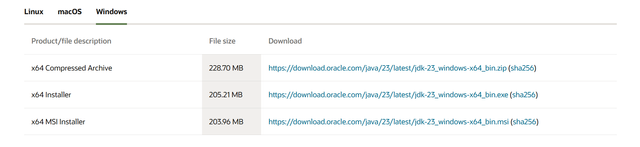
Step 2: Install the JDK
- I have opened the installer setup for the installation. And It has asked some permissions from me so I have given these permissions.
- The setup has opened as you can see in the below image.
- Then I have set the destination folder for the installation.
- Now the installation is going on as you can see here.
- Here you can see the installation has completed.
Step 3: Configuring Environment Variables
- Open the Start menu and search for "Environment Variables."
- Under System Properties, click on Environment Variables.
![]() | ![]() |
---|
3.Add a new system variable:
- Variable Name:
JAVA_HOME
- Variable Value: The path to your JDK installation (e.g.,
C:\Program Files\Java\jdk-XX.X.X
).
![]() | ![]() |
---|
Step 4: Verify Installation
- Open a command prompt or terminal.
- Type
java -version
andjavac -version
to confirm installation.
2. Installation of Eclipse IDE
Step 1: Download Eclipse
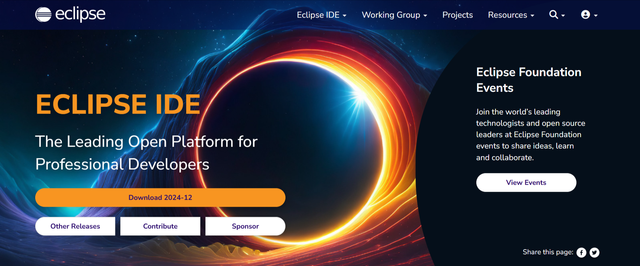
- Go to the official Eclipse website.
- Click on the Download button.
- Here I have proceeded by selecting the suitable installer for my operating system which is windows.
Step 2: Install Eclipse
- Run the downloaded installer.
- Select "Eclipse IDE for Java Developers."
- Choose an installation folder and click "Install."
- Accept the license and terms and conditions to use it.
![]() | ![]() |
---|---|
![]() | ![]() |
Step 3: Launch Eclipse
- Open Eclipse from the installed directory or desktop shortcut.
- Choose a workspace folder (where your projects will be stored).
- Click "Launch."
![]() | ![]() |
---|
Write a program that calculates and displays the sum of the first 100 integers. Define the main method in a class named Sum to handle the entire calculation.
Here are the required programs to calculate and display the sum of the first 100 integers.
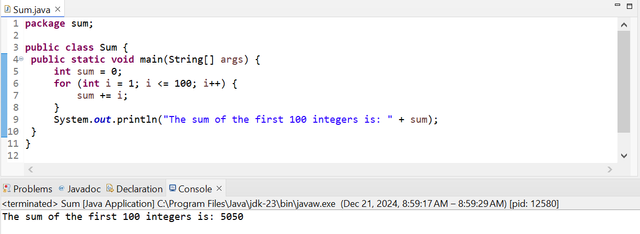
Here you can see that the entire calculation is handled in the main
method. And the main method is Sum
. In this program we have taken an integer
variable sum
to store the sum of the integers. Then there is the for loop to traverse upto 100 integers and each time we are adding the integers in the sum
variable while updating its value.
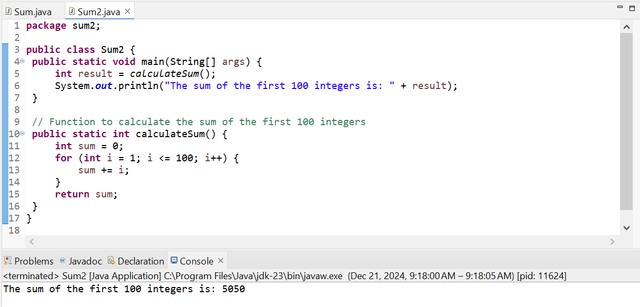
Here you can see that the calculation is performed in the separate function calculateSum()
as it was required. Then I have called this function in the main method and it is returning the sum of the first 100 integers.
Translate, Complete, and Debug the Following Program Named Tax.java
Here is the completed and debugged program for the Tax
class in Java that calculates tax based on the income provided.
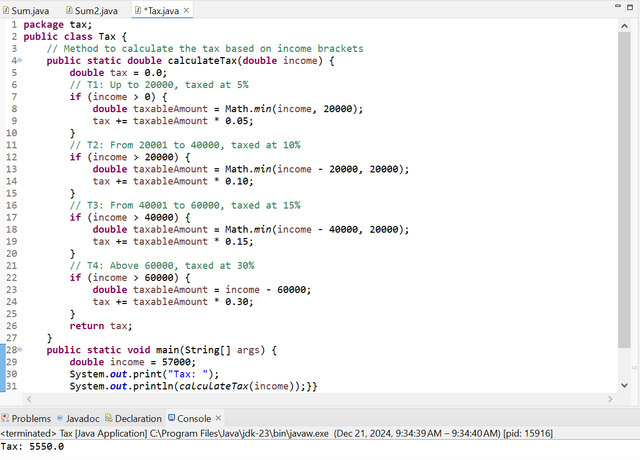
Here in this program the calculateTax
method divides the income based on the limits. It applies the appropriate tax rate for each bracket using conditional checks. Then it accumulates the tax for each. Main method calls the calculateTax
method with an example income of 57000. And in this way it prints the total tax calculated which is 5550.
Using the same program structure as in the previous exercise, write a program named Conversion.java that takes a character c as a parameter
Here is the program Conversion.java
that follows the following requirements:
- Convert uppercase letter to lowercase
- Convert lowercase letter to uppercase
- Checks if a given input is a letter or not and return the message accordingly
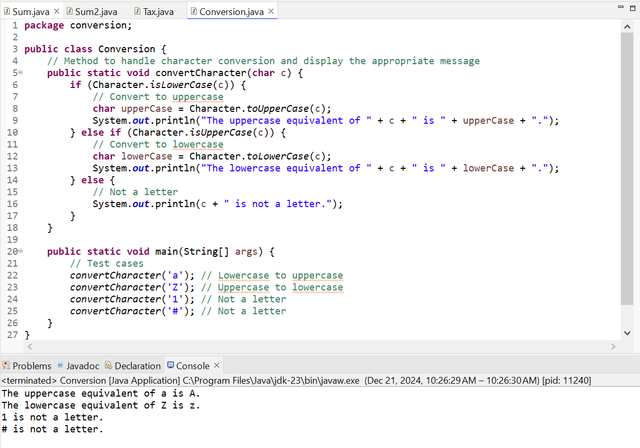
Here in the above program the method convertCharacter
uses Character.isLowerCase
to check if the character is lowercase. And similarly it uses Character.isUpperCase
to check if the character is uppercase. Then after checking it converts the character to its equivalent uppercase or lowercase by using Character.toUpperCase
or Character.toLowerCase
. Then it displays appropriate messages for each case.
In the main method to test the functionality of the program I have given different inputs such as 'a', 'Z', '1', '#'. In the main method these are all as parameter and c
gets the value in the method convertCharacter
and then execute the respective part of the program.
Using a similar structure as in the previous exercises, write a program named ArrayOperations.java that performs operations on an integer array.
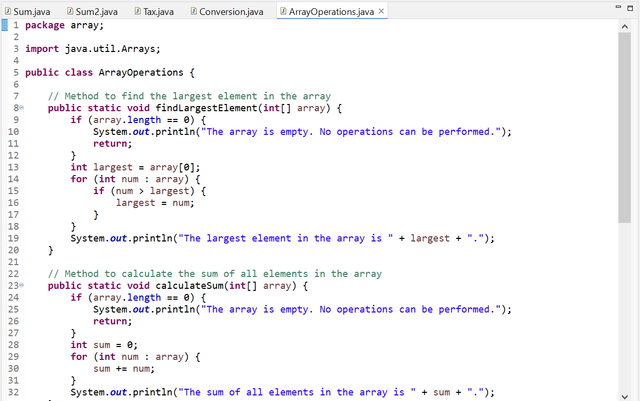
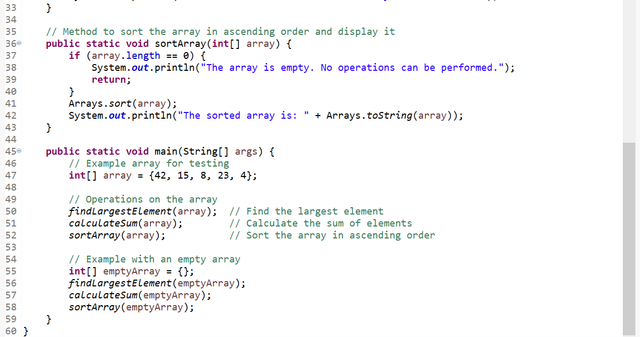

This is the program which is performing the required op[erations of the array. It is accepting an array and then:
- Finding the largest number in the array.
- Returning the sum of the array.
- Returning the sorted array in ascending order.
findLargestElement
method iterates through the array to find the largest element. Then it displays the largest element or a message if the array is empty.
calculateSum
method uses a loop to calculate the sum of all elements in the array. Then it displays the total sum or a message if the array is empty.
sortArray
method uses the Arrays.sort
method to sort the array in ascending order. After sorting it displays the sorted array or a message if the array is empty.
The main method shows the functionality with a test array which has numbers and an empty array to test the functionality of the program when the array is empty.
The first array has numbers in it so the program has returned the required values. But the second array is empty so when the program is calling the methods with an empty array then it is saying that The array is empty. No operations can be performed.
I would like to invite @heriadi, @chant and @jospeha to join this contest.
Undoubtedly, you have mastered all the materials. Especially about Java, of course you are already proficient in this one programming language. Good luck my friend.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thank you dear fellow for stopping by here and leaving your valuable feedback.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit