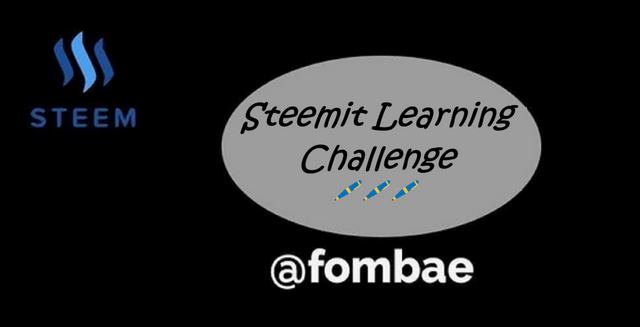
Greetings Steemit friends
Task 1
Here, the program is expected to detect a fault sensor by skipping invalid temperature readings, logging error messages, and continuing to process the rest of the readings.
I create a list of the temperature readings by creating an array (sensorReadings[]
), and create a list to hold valid readings.
Now, I will go ahead and apply my logic, which is to loop through each element in the array. An invalid reading (negative temperature reading) will log an error message. Using the try-catch block, our program will be able to handle the invalid reading, log the error, and continue running.
Task2
Here our program objective is to validate participant ages and log errors for ages out of the ranges. I start by creating a method InvalidAgeException
which will allow the user to show a clear message when the input (age) is invalid.
In the main method, I make use of a while loop to be able to repeat the process until we have a valid input. We made use of a scanner to get the user's age and passed it to the validAge
method. If the input(age )is invalid, an exception is used to throw messages with the InvalidAgeException
method.
The last section of the program is to check the age of less than 18 or greater than 60 and throw the exception or do nothing if age is a valid input.
Task 3
Here, I will try to break the program in a way that will be simple to develop. Our objective is to have a player make a mistake by moving the wrong way or doesn't have sufficient health. Some games will call it hearts.
To handle the mistakes, we used the three methods to create the logic to handle the different errors for the specific problems. So we used HealthException
when health is low, MoveException
when moving the wrong way, and InventoryException
when an item is missing. These are specialized exceptions inherited from the GameException, which is the base class for all the exceptions.
Next, it is our Game Class SimpleRPGame
. I start assigning the attribute health
to keep track of health points and hasRifle
to indicate the player's item.
Here we have two methods, one to handle player movement and the other to handle the item used. The move(String action) method will check if movement is in the wrong direction to throw the MoveException and if health points are insufficient (i.e. less than or equal to zero) to throw the InventoryException
. At this point, if all checks are valid. A success message is printed and moved to the next method useItem(String item)
for the player to use an item. If the player doesn't have the item, throw an InventoryException
. In my case I will use a Rifle(gun), and if there is no error. A success message is printed.
Here is our main Class, and we start by creating SimpleRPGame objects. We are using try-catch blocks to run our program and handle moves by calling game.move("attack")
. We have over 20 hearts so no exception is thrown. If in the case of HealthException
, a message is printed or MoveException
, a message is printed.
Next, to handle item usage by game.useItem("rifle")
. My player doesn't have a rifle, so an InventoryExceptionis thrown
.
- Health is zero(0)
Task 4
Here, I am looking for a system that keeps track of and processes only valid transactions. I start with the exceptions just like the previous task. We have the NegativeTransferException
thrown if the amount to be transferred is negative, and the InvalidAccountException
thrown if the account number is not correct. An InsufficientFundsException
is thrown if the account doesn't have enough balance to do a transfer.
So we will need a class to manage Bank accounts (BankAccount
) and a class to manage the bank system (BankingSystem
). The BankAccount class handles operations like withdraw and deposit. BankingSystem class to manage to add accounts and transfer operations in the bank system. Check if the amount transfer is negative, if the accounts are valid, and if the sender account has enough funds.
Here in the main class, I will create two accounts with funds for test purposes. We will be using the try-catch block to run the necessary operations, with each of them throwing the exception when an error occurs.
We have two accounts "456DC7" with a balance of 500 and 875DC8 with a balance of 300. I used the transfer method to test the different transactions.
case1: Sourceful transfer
case2: Throws NegativeTransferException
case3: Throws InvalidAccountException.
case4: Throws InsufficientFundsException
Task 5
Here we are looking at creating a program that will open multiple files, read their content, and close them. I will make use of the try block while including the Finally block for Resource Cleanup. We have to make use of BufferedReader, and FileReader, and handle file-related exceptions.
In the main class, I start by creating an array containing the name of the files("config.txt", "data.txt", "settings.txt".) the program will have to try reading. If you are using an Eclipse IDE, you place your files in the root directory of the project.
I used the for loop to iterate through each file in the array. We used the BufferedReader for reading the file line by line, and the try block to open and read. If the fill doesn't exist, the IOException
is thrown.
Next is the catch block for printing the messages IOException thrown.. The Finally block makes sure the file is closed, no matter if an exception was thrown during reading. First of all, check if the BufferedReader object is not null before closing and print the message for confirmation.
Task 6
Here we create a program to count and log incorrect login attempts, throwing SuspiciousActivityException to give administrators data to review and halt the services.
I started my program by creating the custom exception SuspiciousActivityException
to handle suspicious login attempts. This will only happen when the cases exceed 3, which is the program threshold.
HashMap<String, Integer>
is used to store the number of failed attempts.
As the user continues to insert an incorrect password, the if statement checks the failed count and adds by one until it gets to the threshold. The SuspiciousActivityException
exception is thrown and goes ahead to print the message "Login failed for user: ". If a password is correct, the failed attend count is reset to 0.
During every attempt, the logActivity()
method logs the username and the number of failed attempts, which will be displayed to the administrator for review.
Cheers
Thanks for dropping by
@fombae
Upvoted! Thank you for supporting witness @jswit.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Greetings,@fombae
This is a small gift from me to you. I just want a little smile from you in return. 😊
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hello! Thanks for sharing your post. This comment was automatically generated by kouba01.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hi friend, great work it seems you have a good knowledge of java all concepts are applied properly.Thanks for sharing and wishing you luck for the contest.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit