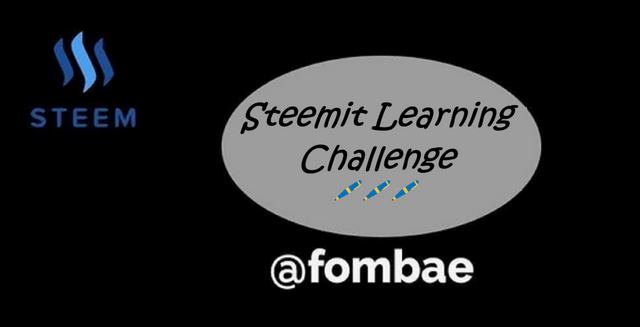
Greetings Steemit friends
Write a program that demonstrates how thread priorities affect execution order. Create three threads and assign them the priorities MIN_PRIORITY, NORM_PRIORITY, and MAX_PRIORITY. Run the threads and observe the execution order. Provide an explanation of whether the thread priority influences their execution order and why.
Here, I will use the example shared in the course on Java Thread Priorities to have the relative order for executions and the effect of Thread priorities.
We start by creating a PriorityExample
Class that extends to the Thread class, which I will have the run method. In the main class, I will create the thread objects. For each of the threads, I will use a different string for the name parameter.
I set the Thread priorities by assigning the priorities and starting the Threads.
The Thread priority influenced the scheduling order. At some point, the High Priority Thread runs first, the Normal Priority Thread second, and then the Low Priority Thread. If you run the threads again, the Thread priority scheduling order can vary depending on the Java Virtual Machine (JVM) and operating system.
So at this point, I can't guarantee a strict Thread priority scheduling execution order. That means, using Thread priority scheduling only when it is necessary.
Develop a program that intentionally causes a deadlock using synchronized methods. Design a scenario where two or more threads attempt to acquire locks on shared resources in reverse order, leading to a deadlock. Provide a detailed explanation of how the deadlock occurs and suggest strategies to prevent it.
Here we are looking at designing scenarios to intentionally cause a deadlock. A deadlock is when two or more threads are blocked, and one has to wait for the other to release a lock on a resource. We can always have a deadlock when threads want to acquire locks on shared resources in a circular or our case will be in a reverse order.
I will be making use of the template format shared in the course, starting by creating the Resource
class with the two synchronized methods, MethodA()
and MethodB()
. MethodA()
locks the current Resource instance either resourceA
or resourceB
and tries to call MethodB()
, which will attempt to acquire the lock. MethodB()
synchronized method that prints the message and needs the lock of the Resource instance
My main class has two Resource objects, that is resourceA
or resourceB
, and the two threads are defined (thread1
and thread2
).
Thread 1 Locks resourceA
and then resourceB
, that is why it will print the message and attempt to call resourceB.MethodB()
and vice versa for Thread 2. Both threads are simultaneously executing while waiting for each other to release their respective locks. For this reason, causing a deadlock as each other prevents the other from having access.
To prevent deadlock, carefully design a consistent lock ordering. That means having both Threads attempting to acquire locks in the same order. That way we have the thread attempting to acquire resourceA first before attempting to acquire resourceB.
Task 3: Create a program where multiple threads count from 1 to 100 concurrently. Ensure that the numbers are printed in the correct order without conflicts, even though the counting occurs in parallel. Describe the mechanisms (e.g., synchronization) used to maintain the correct sequence.
Okay, we are preventing a deadlock and making sure the multiple threads count from 1 to 100 and print numbers in order without conflict. I will be using the synchronization mechanisms to maintain the correct sequence while making use of the shared counter.
I started by creating a Counter
class as we created the resources class in the previous task. Now I will have a variable to manage the count starting at 1. I synchronized the block in the method printNumber()
to make sure only one Thread prints the count at any given time while the increment continues to reach the maximum count (100)
In the main class, I created a thread that will share the same counter object. They will all call the printNumber()
to count from 1 to 100. With the used synchronized, wait()
, and notifyAll()
. I can maintain the correct sequence, as wait()
stops the current thread until it is notified by another thread.
Write a program that uses a thread pool to process a list of tasks efficiently. Implement a set of simple tasks (e.g., displaying messages or performing basic calculations) and assign them to the threads in the pool. Explain how thread pools improve performance compared to creating threads for each task individually
Here we are creating a Thread Pool, which will help me to collect pre-instantiated, reusable threads to execute tasks from the list.
I start by creating a class SimpleTask
that implements Runnable
for each task to be executed by a thread. Each task will print a message and then sleep for a second to simulate.
In the main class, I used the Executors.newFixedThreadPool()
to create the threadPool, which will create 4 threads to process the task. Here is the pool to manage the threads and reuse them to perform tasks.
The pool will assign tasks to the available threads by using the threadPool.execute()
, which I used as a for loop to submit 10 tasks.
The threadPool.shutdown()
is used to shut down threads, making sure all submitted tasks are completed.
Thread pools improve performance by reducing overhead, as we will not have to create a new tread for each task. Note thread pools will help limit the number of threads, and at the same time handle a large number of tasks.
Write a program where multiple threads read different parts of a file simultaneously. Divide the file into distinct segments, assign each segment to a thread, and print the content read by each thread. Explain the logic used for dividing and assigning file segments to avoid conflicts and ensure efficiency.
This was a little challenging, as I had to start by finding a way to read a file, separate it, and assign it to a thread without any conflict. I will need multiple threads, which will handle the different parts of the file simultaneously.
I create a FileReader
Class and implement Runnable
for reading specific segments of the file while taking into consideration the start
and end
. The start
and end
is to define the section to be read from the file. The RandomAccessFile
acts as a pointer in reading specific segments of the file.
In the main class, use RandomAccessFile.length()
to determine the file length and have equal segments by dividing the fileLength / threadCount
to have the segmentSize.
The logic used for dividing is to use the start and end offsets to have the segmentSize
, which will help not to have any conflict as threads are being executed. With the use of the pointer RandomAccessFile
, threads can read specific segments without loading the whole file.
Develop a program simulating a bank system where multiple threads perform deposits and withdrawals on shared bank accounts. Use synchronization to ensure thread safety and prevent issues such as race conditions. Provide an explanation of the techniques used to maintain data integrity in the presence of concurrent threads.
Here we are expected to build a bank system to simulate transactions making use of threads to perform the operations. So my program needs to be able to perform deposits and withdrawals on shared bank accounts.
I will start by creating a BankAccount
class, with synchronized methods for depositing, withdrawing, and getting the balance. The deposit()
, withdraw()
, and getBalance()
are synchronized to make sure only one thread is executed and the others are blocked from executing the other method on the same instance.
Now in the main Class, I create a thread to represent the action taken on an account. I used the start()
method to create and start threads making use of the BankAccount
object. I passed the account number [002345607819]
and the initial balance of [5000] FCFA
. Each of the threads will perform the task of depositing, withdrawing, or checking the balance and lock the BankAccount
object. That way no other thread can use it until the lock is released.
Cheers
Thanks for dropping by
@fombae