This is my homework post for Steemit Engagement Challenge Season 20 Week 5 assignment of Professor @alejos7ven’s class, Control Structures Part 2 : Cycles.
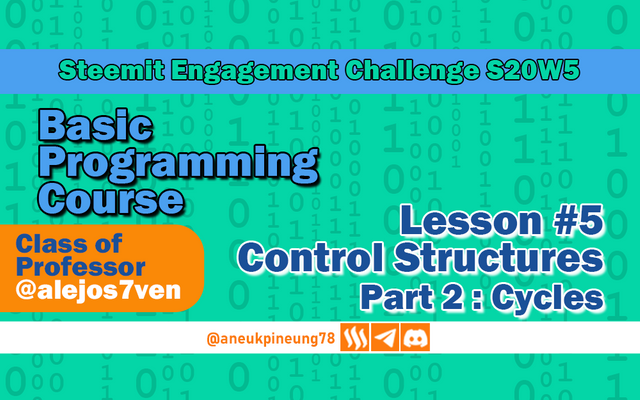
Task 1 - Explain the cycles in detail. What are they and how are they used? What is the difference between the While and Do-While cycle?
As implied in the name, cycles in programming languages are loops that occur by the program to execute certain blocks of code continuously as long as a condition is TRUE
or until a condition is met or until a specified number of iterations is reached.
There are 3 types of Cycle:
The simplest is the For Cycle, where the loop occurs in a known number of times, e.g., Steemians are expected to write 4 comments on a certain post in a certain community to be eligible for the next round of a contest in SEC. Then the condition will continue to be
TRUE
as long as the comments have not reached 4, and so the loop (request to continue writing comments) will continue until they post the 4th comment. The 4th comment makes the conditionFALSE
and therefore removes the loop, and they can now proceed to the next round. In this case we can easily tell that the loop will occur in 4 iterations.Algorithm CommentsCountFor // 1 Declare variables Define CommentsNumber, CommentsWritten As Integer; CommentsNumber = 0; // 2 For cycle for 4 Comments For i = 1 to 4 Do Print "Write comment", i, ": "; Read CommentsWritten; CommentsNumber = CommentsNumber + CommentsWritten; EndFor // 3 Check if total comments is equal to 4 If CommentsNumber = 4 Then Print "Congratulations! 4 comments have been made." Print "You are eligible for the next round."; EndIf EndAlgorithm
In action:
Image is clickable and might show larger resolution. While Cycles, where the program cannot predict the response (input) that will be received from the user, so the
TRUE
condition can continue to be reached in an indeterminate number of iterations, which can be very short or very long. For example, in an activity on Stemit, every day participants are asked to report the number of comments they started today until the number of comments reaches 100 and they will be delegated some amount of SP. It cannot be estimated that a participant will reach the target of 100 comments in how many days, it depends on how diligent he/she is in writing comments.Algorithm CommentsCountWhile // 1 Declare variables Define CommentsNumber, CommentsToday As Integer; CommentsNumber = 0; // 2 Repeat until total comments reaches 100 While CommentsNumber < 100 Do Print "How many comments did you make today? "; Read CommentsToday; CommentsNumber = CommentsNumber + CommentsToday; // 3 Display current total Print "Total comments made so far: ", CommentsNumber; Print "----------------------------------"; // 4 Check if total comments has reached 100 If CommentsNumber >= 100 Then Print "Congratulations! You have made" CommentsNumber "comments."; Print "100 SP will be delegated for your account within the next 24 hours."; EndIf EndWhile EndAlgorithm
In action:
Image is clickable and might show larger resolution. Do While Cycle. This loop is similar to While, the difference is that in Do While a block of code is executed at least once before the condition is checked. For example, in an activity to empower new Steemians, participants of the activity are required to be active in engagement, by filling in their daily comment count every day. The program asks them for the number of comments they have made today, and if their comment count reaches 100 (starting today) then they will be delegated some amount of SP. The program will run a block of code to check the number of comments that have been made today, before running a looping structure until the number of comments reaches 100. As long as the number of comments has not reached 100 (
TRUE
), each day they will continue to be asked to enter their daily number of comments, and when the number of comments reaches 100, the program will stop the loop because the condition has reached theFALSE
value, and continue to the next block of code, which could be an announcement that they have been entitled to a number of SP delegates.Algorithm CommentsCountDoWhile // 1 Declare variables Define CommentsNumber, CommentsToday As Integer; CommentsNumber = 17; // 2 Repeat asking for daily comments until total comments has reached 100 Do Print "How many comments did you make today? "; Read CommentsToday; CommentsNumber = CommentsNumber + CommentsToday; // 3 Display current total Print "Total comments made so far: ", CommentsNumber; Print "----------------------------------"; // 4 Check if total comments made has reached 100 If CommentsNumber >= 100 Then Print "Congratulations! You have made" CommentsNumber "comments."; Print "100 SP will be delegated for your account within the next 24 hours."; EndIf Print "----------------------------------"; Until CommentsNumber >= 100 EndAlgorithm
In action:
Image is clickable and might show larger resolution.
So the difference between While and Do While cycles is that in Do While, a block of code is executed (run) at least once before the condition is checked.

Task 2 - Investigate how the Switch-case structure is used and give us an example.
Switch Case is one of the control structures in programming that is similar to multiple choice, so in Switch Case there are several options that can be selected and the program will run the block of code according to the choice. Switch Case is a cleaner and easier alternative to condition structures like If-Else
that can become very long in certain cases. For example, Steemian is asked to type the number 1 if verified, and the number 2 if not verified. When the number 1 is given as input, the program can display the message “You can join SEC”, and “You cannot join SEC yet” if the number 2 is given as input.
For simple cases like in the example in the paragraph above, the If-Else
condition structure may still be reliable, but in more complex cases, let's say there are 10 conditions to choose from, then the If-Else
structure will become very long and not as clean as the Switch Case structure.
Example:
Algorithm FavoriteTutor
// 1. Declare variable
Define favorite As Integer
// 2. List of classes
Print "Enter your favorite tutor by typing a number (1-4) to see this week class of theirs:"
Print "Type 1 if you favor @alejos7ven"
Print "Type 2 if you favor @lhorgic"
Print "Type 3 if you favor @dexsyluz"
Print "Type 4 if you favor @simonnwigwe"
Read favorite
// 3. Switc Case to Determine the favorite class
Switch Choice
Case 1:
Print "Your favorite tutor is @alejos7ven."
Print "This week class is Control Structures Part 2: Cycles."
Print "Join class here: https://steemit.com/devwithseven/@alejos7ven/basic-programming-course-lesson-5-control-structures-part-2-cycles-esp-eng."
Case 2:
Print "Your favorite tutor is @lhorgic."
Print "This week class is Graphic Design Hands-on Practical 2"
Print "Join class here: https://steemit.com/hive-147599/@lhorgic/sec20-wk5-graphic-design-hands-on-practical-2"
Case 3:
Print "Your favorite tutor is @dexsyluz."
Print "This week class is Hemolytic Anemias"
Print "Join class here: https://steemit.com/hematology-s20w5/@dexsyluz/sec-s20w5-or-or-hematologia-anemias-hemoliticas-modulo-5"
Case 4:
Print "Your favorite tutor is @simonnwigwe."
Print "This week class is Creating Sheets & Form Part 2"
Print "Join class here: https://steemit.com/spreadsheet-s20w5/@simonnwigwe/3ogis6-sec-or-s20w5-or-google-sheets-creating-sheets-and-form-part-2"
Default:
Print "Wrong answer, you must type the number 1 or 2 or 3 or 4."
Print " Enter a number (1-4): "
EndSwitch
EndAlgorithm
When executed:
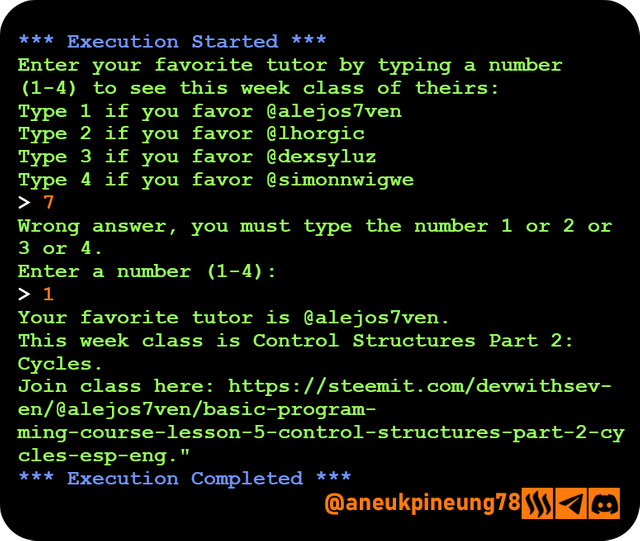

Task 3 - Explain what the following code does:
Algorithm switchcase
Define op, n, ns, a As Integer;
Define exit As Logic;
exit = False;
a=0;
Repeat
Print "Select an option:";
Print "1. Sum numbers.";
Print "2. Show results."
Print "3. End program.";
Read op;
Switch op Do
case 1:
Print "How much nums do you want to sum?";
Read n;
For i from 1 to n Do
Print "Enter a number: ";
Read ns;
a = a + ns;
EndFor
Print "Completed! Press any button to continue";
Wait Key;
Clear Screen;
case 2:
Print "This is the current result: " a;
Print "Press any button to continue.";
Wait Key;
Clear Screen;
caso 3:
Clear Screen;
Print "Bye =)";
exit = True;
Default:
Print "Invalid option.";
Print "Press any button to continue";
Wait Key;
Clear Screen;
EndSwitch
Until exit
EndAlgorithm
What I understand from the above code is that there is a Switch Case control structure combined with a For Cycle repetition structure. What happens when the program is run:
- The program prompts the user to enter a number according to the option.
- The program will read the input, and execute the code block according to this input:
- if the user writes 1, then the program will ask for the number of numbers to add, if the user writes 5 then the program will repeat until 5 numbers have been written and the program will add the five numbers and end the loop by displaying the message “Completed! Press any button to continue”;
- if the user writes 2, the program will show the current calculation result, then display the message “Press any button to continue.”, wait for the next input from the user, and display the message according to the input;
- when button 3 is pressed as input, the screen will be “cleared” and will display the message “Bye =)”. Then the program is closed.
- if the user provides input other than the numbers 1, 2, or 3, provided in the options, the program will display the message “Invalid option.” and “Press any button to continue”, the program will wait for the next input and execute the block based on it.
Put in to action:
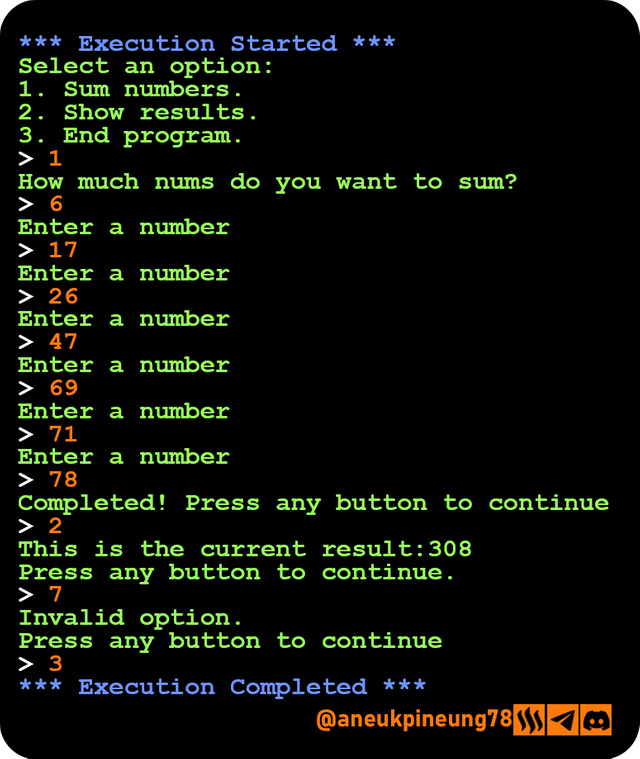

Task 4 - Write a program in pseudo-code that asks the user for a number greater than 10 (Do-While), then add all the numbers with an accumulator (While) Example: The user enters 15. The program adds up to 1+2+3+4+5+6+7+8+9+10+11+12+13+14+15.
Algorithm WhileDoWhileAndAccumulator
// 1 Declare variables
Define count, accumulation, number As Integer;
count = 1;
accumulation = 4;
// 2 Using Do-While to ask for a number greater than 10 with
Do
Print "Type a number greater than 10: "
Read number
If (number <= 10)
Print "Try again, a number greater than 10.";
EndIf
Until number > 10
// 3 Adding the number with the accumulator 8 times using While Loop
While count < 8 Do
Print "Count: " count;
Print "Accumulation: " accumulation;
Print "-------------------------------------";
accumulation = accumulation + number
count = count + 1
EndWhile
// 4 Show the final resul after 8 additions
Print "Final Result : ", accumulation
EndAlgorithm
Explanation of each block:
- Variable decalaration:
- The program will store the user-entered number;
- the number of iterations in subtraction will be stored with an initialization value of 1, using the count code;
- the total accumulation will be stored with an initial value of 3, code: accumulation;
- Do-While cycle:
- the program asks the user for a larger number;
- if the user enters a smaller number such as 9, the program will display the message “Try again, a number greater than 10.” until the user enters a number greater than 10.
- While cycle
- After the user enters the correct number, e.g. 13, the program will repeat the operation up to 8 times in the accumulation;
- The program displays the count and accumulation values at each iteration, followed by a dividing line between iterations, allowing the user to observe the process at each iteration;
- The variable count is incremented so that the number of iterations that have been passed is known;
- Show Final Result will display the final result of this accumulation operation.
Let's see it in the practice:
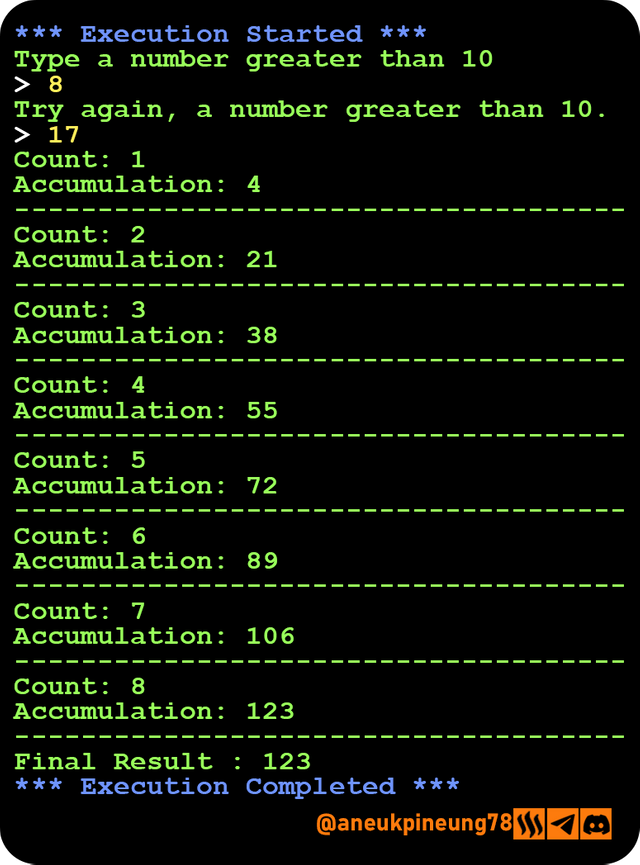

Thanks
Thanks Professor @alejos7ven for the lesson.
Pictures Sources
- The editorial picture was created by me.
- Unless otherwise stated, all another pictures were screenshoots and were edited with Adobe Photoshop 2021.


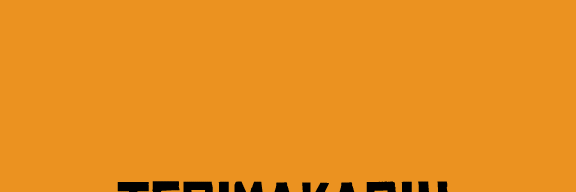

https://x.com/aneukpineung78/status/1845064727015395664
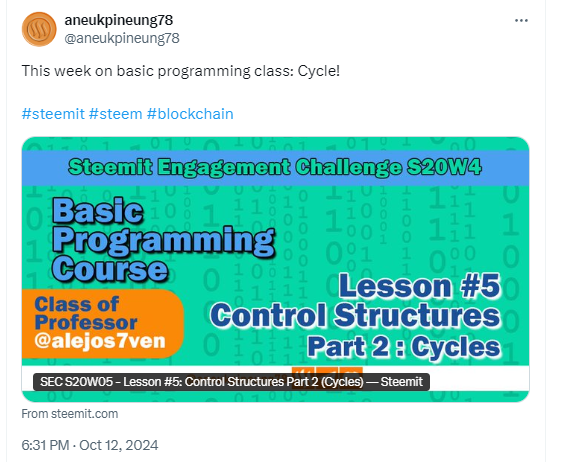
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
This post has been upvoted/supported by Team 5 via @httr4life. Our team supports content that adds to the community.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Saya selalu senang melihat steemian yang aktif mengikuti SEC, khususnya tentang programmer. Karena selain di kelola oleh orang-orang yang profesional juga karena menjadi sesuatu untuk kita belajar dan mengerti.
Sukses selalu buat Kanda @aneukpineung78
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Sebenarnya mumang juga, Ketua, tapi kelas Professor Alejos7ven ini adalah kelas programming termudah (menurut saya) dari 4 kelas yang ada. Nanti kita lihat penilaian Prof., apakah saya masih dianggap "di jalur" atau udah "melenceng". Hahaha. Terimakasih, ya.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hahaha..
Tapi saat kita menempuh jalur sulit dan penuh tantangan, adrenalin kita akan meningkat dan semakin tertantang..
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Selain itu, menyebabkan kurang tidur juga ,,, 😆😆
Bang @walictd malah dia sanggup mengikuti kelas Prof. Kouba (bersama StarChriss). Saya lempar handuk. Baru baca artikel kelas saja, kolesterolku langsung melonjak. 😆😆😆😆
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Sama juga bang, aku juga pusing dari 2 hari kemarin tes ngehosting web, tdi baru bisa. Adehhh
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hahaha. SEC memang bikin orang-orang kurang tidur tapi tambah pintar. :v
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hahahaha
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Rapor sudah keluar, ternyata saya masih di jalan yang benar, Ketua. Laporan selesai. :v
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Mantap...😀😀😀👍
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit