This is my homework post for Steemit Engagement Challenge Season 20 Week 4 assignment of Professor @alejos7ven’s class, Basic Programming Course: Lesson #4 Control Structures Part 1: Conditionals.
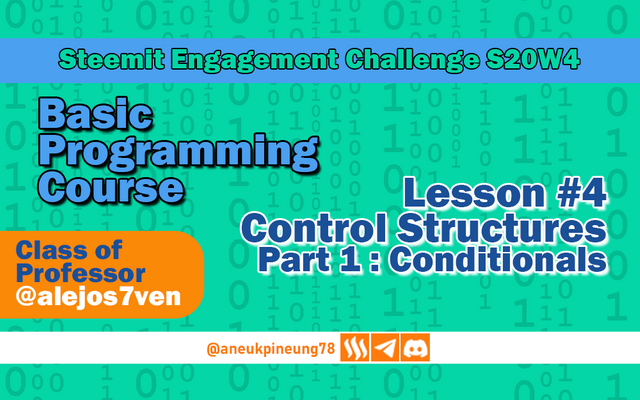
Task 1 - Describe your understanding of conditional structures and mention 2 situations of everyday life where you could apply this concept.
Conditional Structures are a structured way of making decisions based on certain conditions, meaning that there are different execution paths created based on different conditions, and the execution will be done by seeing which condition is TRUE
or FALSE
.
Conditional structures range in complexity from simple (e.g. If Statement) to complex (e.g. conditionals with nested logical operators). Some of the expressions of conditional structures in programming are:
1. If Statement. This is the simplest and basic expression, it executes a decision if a condition is met (TRUE). The code will be executed if the condition is TRUE. For example, a Steemian can enter the Steemit Engagement Challenge contests if they have already verified. In this case, “can enter contests” is the code, and “verified” is the condition.
Algorithm ContestEligibility
// 1. Declare variable
Define verification As Character
// 2. Ask the user if they are verified
Print "Are you verified on Steem? (yes/no): "
Read verification
// 3. Evaluate the answer
If (verification == "yes") Then
Print "Good! You can participate in all of the SEC contests. Whichever you like!"
EndIf
EndAlgorithm
2. If-Else Statement. This is a more complex kind of statement than the If Statement. In this type of statement there are 2 variables that will be the basis for decision making (execution). If the code is TRUE then the corresponding condition will be executed, if the code is FALSE then the program will look for and execute another condition. This means that there are different blocks of code that are used as a reference by the program to execute. Example:
- If a Steemian is verified, then he can participate in SEC contests,
- if not, he cannot.
Algorithm ContestEligibility
// 1. Declare variable
Define verification As Character
// 2. Ask the user if they are verified
Print "Are you verified on Steem? (yes/no): "
Read verification
// 3. Evaluate the answer
If (verification == "yes") Then
Print "Good! You can participate in all of the SEC contests. Whichever you like!"
Else
Print "My friend! You are tresspassing. This area is forbidden for you.”
Print “Go back and get yourself verified before trying to get involved in any of the SEC contests."
EndIf
EndAlgorithm
3. Elif (Else If) Statement. This statement evaluates several different consecutive conditions. For example, in determining Steemians classification according to MVEST ownership:
- below 9 MVEST, then they are called Minnows;
- between 9 to 99 MVEST, then they are categorized as Dolphins;
- between 99 to 999 MVEST, Orcas;
- above 1000 MVEST, Whales.
Algorithm SteemianClass
// 1. Declare variable
Define SteemName, curiosity As Character
Define MVEST As Real
// 2. Observe the user's curiosity
Print "Dear Friend, what is your Steem Name?: "
Read SteemName
Print “Hello, " SteemName "Are you here to know your classification? (yes/no)”
Read curiosity
// 3. Evaluate Curiosity
If (curiosity == "yes") Then
Print "Enter the amount of MVEST you have: "
Read MVEST
// 4. Steemian classification based on MVEST
If MVEST < 9 Then
Print "You are a Minnow. Work harder and Power Up harderer!"
Elif MVEST < 99 Then
Print "You are a Dolphin. That’s cool! But is it enough?"
Elif MVEST < 999 Then
Print "You are an Orca. Wow! You made it here!"
Elif MVEST >= 1000 Then
Print "You are a Whale. Great! Congrats! Don’t let it hold you back from doing more Power Up, alright? And please vote @aneukpineung78 now and then."
EndIf
Else
Print "So, " SteemName ", what are you doing here? (Sarkasm. No answer needed.)"
EndIf
EndAlgorithm
4. Nested If. This structure shows a condition inside another condition. Example:
---- if it is a sunny day, then
-------- if there is a vehicle then I will go to the city to buy food;
-------- if not then I will buy food at a stall near the house;
---- if not then I will cook;
Algorithm FoodBuyingDecision
// 1. Declare variables
Define weather As Character
Define vehicle As Character
// 2. Ask the user if it is a sunny day
Print "What is the weather? (Sunny/Rainy): "
Read weather
// 3. Check the weather
If (weather == "Sunny") Then
// Ask the user if there is a vehicle
Print "Is there a vehicle? (Yes/No): "
Read vehicle
// Check if there is a vehicle
If (vehicle == "Yes") Then
Print "You can go to the city to buy food and maybe meet some friends.”
Print “How long has it been since the last time you go out? Meet a girl? (Sarkasm. No answer needed)."
Else
Print "You can buy food at a stall near the house.”
Print “Remember to not buying from Ms. Bananacrusher. You know why!"
EndIf
Else
Print "You must cook! Good for you! Mama will be very proud!"
EndIf
EndAlgorithm
5. Switch Case, which is a command structure for the program to execute based on variable values. For example, there are 4 class options in SEC and Steemian is asked to choose the one class he likes the most. For example:
- type “1” if you favor Programming Basics class;
- type “2” if you favor Graphic Designing class;
- type “3” if you favor Fitness class;
- type “4” if you favor Health class;
In this structure, a default “wrong answer message” is required that will be displayed when the user’s answers in not in the options, for example if they type “5”, the default message can read, “I can’t believe it! You must type the number 1 or 2 or 3 or 4”.
Algorithm FavoriteClass
// 1. Declare variable
Define favorite As Integer
// 2. List of classes
Print "Enter your favorite class by typing a number (1-4):"
Print "Type 1 if you favor Basic Programming class"
Print "Type 2 if you favor Graphic Designing class"
Print "Type 3 if you favor Fitness class"
Print "Type 4 if you favor Health class"
Read favorite
// 3. Switc Case to Determine the favorite class
Switch Choice
Case 1:
Print "Your favorite is Basic Programming class."
Case 2:
Print "Your favorite is Graphic Designing class."
Case 3:
Print "Your favorite is Fitness class."
Case 4:
Print "Your favorite is Health class."
Default:
Print "Wrong answer, you must type the number 1 or 2 or 3 or 4."
EndSwitch
EndAlgorithm
The two situations in my everyday life that similar to Conditional Structure in programming are
Situation 1 : I have plans to write for the SEC and also have some office work that needs to be done urgently. I need to prioritize office work. So the decision that resembles the conditional structure rule in programming is: I will write for SEC if the office work is done, otherwise I will continue doing the office work.
Algorithm SEC_and_Office
// 1. Declare variables
Define status As Character
// 2. Ask the user if the Office works is finished
Print "Have you finished the office works? (Yes/No): "
Read status
// 3. Check the status
If (status == "yes") Then
Print "You can work on SEC Contests."
Else
Print "You should finish the office works before doing anything else.”
EndIf
EndAlgorithm
Situation 2 : In the middle of the night while writing for the SEC I felt hungry. I forgot whether I had noodles and egg or not, on the way to the kitchen I said to myself,
Algorithm MidnightHungerStrike
// 1. Declare variable
Define noodleAvailability As Character
Define eggAvailability As Character
// 2. Ask the availability of noodle
Print "Dear Friend, Is there noodle? (yes/no): "
Read noodleAvailability
// 3. Evaluate noodleAvailability
If (eggAvailability == "yes") Then
Print "Is there any egg?: (yes/no): "
Read eggAvailability
// 4. Evaluate eggAvailability
If (eggAvailability = "yes") Then
Print "Make boiled noodles. It's yummy. And will serve you good in the middle of the night."
Else
Print "Make fried noodles, my friend!"
EndIf
Else
Print "Fried rice is the only answer tonight, my beloved friend! Get on with it!"
EndIf
EndAlgorithm

Task 2 - Create a program that tells the user "Welcome to the room What do you want to do?", if the user writes 1 then it shows a message that says "you have turned on the light", if the user writes 2 then it shows a message that says "you have left the room". Use conditionals.
Based on my findings in the internet, this second task falls into the use case of the Switch or Switch-Case (some languages like Python call it Match) structure. Below is an algorithm that I can write based on my understanding so far in this class and by looking at examples on the internet, for the task.
Algorithm WelcomeToTheRoom
// 1. Declare variable
Define answer As Integer
// 2. Show the welcom message
Print "Welcome to the room. What do you want to do?";
Print "Write 1 if you want to turn on the light";
Print "Write 2 if you are leaving the room";
// 3. Get answer
Read answer;
// 4. Check the answer
If (answer = "1") Then
Print "You have turned on the light";
If (answer = "2") Then
Print "You have left the room";
Else
Print "What is wrong with you? You should have typed 1 or 2.";
EndIf
EndAlgorithm
Or maybe I can use Switch Case Structure?
Algorithm WelcomeToTheRoom
// 1. Declare variable
Define answer As Integer
// 2. Show the welcom message
Print "Welcome to the room. What do you want to do?";
Print "Write 1 if you want to turn on the light";
Print "Write 2 if you are leaving the room";
Read answer;
// 3. Check the answer using Switch Case
Switch Choice
Case 1:
Print "You have turned on the light";
Case 2:
Print "You have left the room";
Default:
Print "What is wrong with you? You should have typed 1 or 2.";
EndIf
EndSwitch
EndAlgorithm

Task 3 - Create a program that asks the user for 4 different ratings, calculate the average and if it is greater than 70 it shows a message saying that the section passed, if not, it shows a message saying that the section can improve.
This case combines basic arithmetic functions and comparison operation with an If-Else Statement condition structure.
Algorithm RatingAverage
// 1. Declare variables
Define rating1, rating2, rating3, rating4, average As Real
// 2 Ask the user to input their ratings
Print "Enter rating 1: ";
Read rating1
Print "Enter rating 2: ";
Read rating2
Print "Enter rating 3: ";
Read rating3
Print "Enter rating 4: ";
Read rating4
// 3. Calculate the average
average = (rating1 + rating2 + rating3 + rating4) / 4;
// 4. Checking if average is greater than 70
If average > 70 Then
Print "The Section Passed";
Else
Print "The Section Can Improve";
EndIf
EndAlgorithm
I can understand this for example the operation in a Spreadsheet application such as MS Excel, which collects numerical data and then performs the operation of finding the average value which then becomes the reference for decision making.
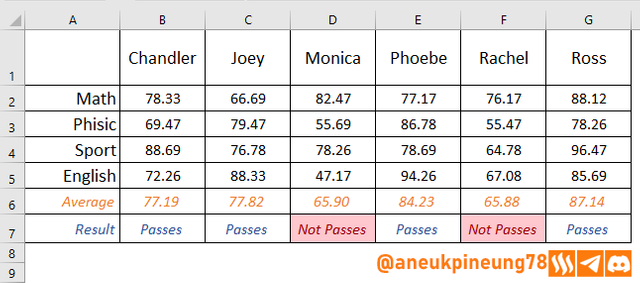

Thanks
Thanks Professor alejos7ven for the lesson.
My Previous Articles on This Class (Newest to Oldest)
SEC Week | Link | Date |
---|---|---|
3 | Lesson #3 Operations | Sept 27 |
2 | Lesson #2 - Variables and Types of Data | Sept 20 |
1 | Lesson #1 - Introduction To Programming | Sept 13 |


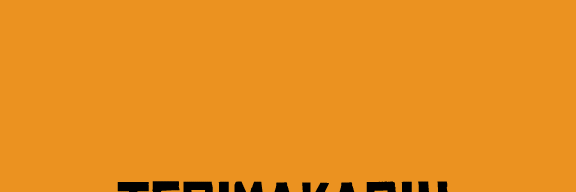

https://x.com/aneukpineung78/status/1842786783362441400
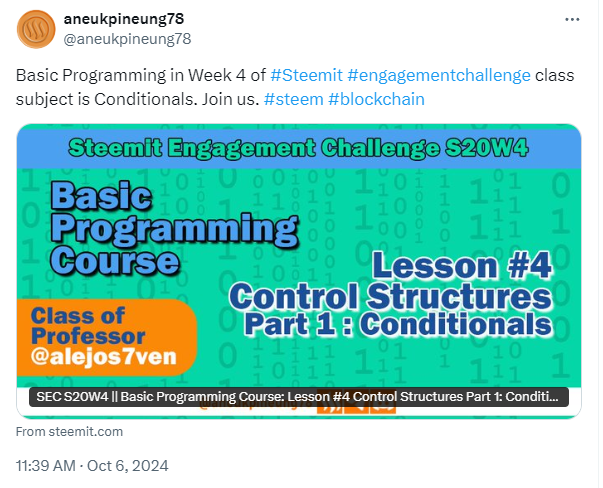
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Saya selalu salut dengan orang-orang yang mengerti tentang programmer. Karena keahlian khusus tersebut dalam menghasilkan karya yang luar biasa dan berguna dan mempermudah pekerjaan orang lain.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Kelas profesor @alejos7ven ini masih sangat dasar sekali, dan dia melakukannya dengan begitu terstruktur sehingga kita mudah mengikuti alurnya, dan juga dia membawakan kelas dengan cara yang mudah dipahami oleh orang yang bahkan tidak memiliki pengetahuan dasar apapun tentang pemrograman, seperti saya. Saya harap kelas ini akan terus ada pada musim-musim SEC selanjutnya, sehingga saya bisa terus mempelajarinya. Saya menemukan ini menyenangkan, membuat kemampuan logika kita terasah selama mengerjakan tugas. Dan bukan hanya itu, tugas-tugas di kelas ini juga membuat kita bisa mengevaluasi tingkat kejelian kita terhadap detail.
O iya, saya lihat Bang @walictd juga telah menulis untuk kelas profesor Alejos7ven di sini. Dia mempraktekkan dengan cara yang di luar apa yang saya bayangkan, yaitu dia telah membuat sejenis program yang mampu memberikan contoh antarmuka pengguna jika kode-kode yang dimintakan dalam tugas diimplementasikan dalam praktek sebenarnya. Itu sangat keren menurut saya.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Anda berdua memang keren banget bang....👍👍👍👍
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Aduh bang maap banget lama ngebalasnya, soalnya mau buat postingan dan lagi nginstall dev c++ untuk ikot challenge, namun si laptop jadul dan kentangku minta ganti. Ntah gimana ni gatau bilang kan.
Pusing-pusing...., maaf pak bos @anroja untuk review posting kayaknya agak slowly dikit ni gapap kan.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit