Hello Steemians,
I hope your code is running without any warning😉, Today we will talk about how to get the vote amount in steem dollars ($). We will use the API provided by @steemchiller. The code will be in typescript and you can use the same code for the Javascript project with a little change.
What is rshares
rshare basically is the short form of Reward Shares. When a user votes for a post with a weight the amount of share is added to the net_rshare of the post.
What is net_rshares
It is the total of all the voter's rshare.
Total Reward
total reward is calculated by adding the pending and the curator payout;
total_reward = pending_payout_value + curator_payout_value
To get the post data we need the author and the permlink, We will use the sds1 API to send the request.
export const fetchPostSDS = async (
author: string,
permlink: string,
withVoters: boolean = true,
)=> {
try {
const post = fetch(`https://sds1.steemworld.org/posts_api/getPost/${author}/${permlink}/${withVoters}/author,pending_payout_value,curator_payout_value,net_rshares`)
.then((response) => response.json()) // Parse the response in JSON
.then((response) => {
return response.result; // Cast the response type to our interface
});
return post;
} catch (error) {
console.error('failed to get fetchPostVoters', error.message);
return null;
}
};
Now we need to call this function to get the values from the API response.
const author = props.post_data.author;
const permlink = props.post_data.permlink;
const [initialFetch, setInitialFetch] = useStateIfMounted(false);
const [rows, setRows] = useStateIfMounted([]);
const [cols, setCols] = useStateIfMounted<VotesColsSDS>(null);
const [ratio, setRatio] = useStateIfMounted(0);
useEffect(() => {
if (!initialFetch) {
_fetchPost();
}
}, []);
const _fetchPost = async () => {
if (!initialFetch) {
console.log('fetching');
await fetchPostSDS(author, permlink, true).then((res) => {
let voters = res.votes.rows;
// sort
voters = voters.sort((a, b) => b[res.votes.cols.rshares] - a[res.votes.cols.rshares]);
// set table columns in state
setCols(res.votes.cols)
// set voter rows in state
setRows(voters);
// This state will stop the duplicate requests
setInitialFetch(true);
// set the ratio in state
setRatio((res.curator_payout_value + res.pending_payout_value) / res.net_rshares)
}).catch((e) => {
console.error(e);
})
}
}
Now in the renderItem we will use a calculation to get the vote amount in dollars.
const _renderItem = ({ item }) => (
<View style={{ flexDirection: 'column'}}>
{/* {Your View} */}
<Text>{`$${(item[cols.rshares] * ratio).toFixed(2)}`}</Text>
</View>
);
I hope you all find this method information useful. If you have any query then ask me in the comment section. Thank You.
Happy Coding !!!
Special Thank to:
@rme
@blacks
@hungry-griffin
@steemchiller
VOTE @bangla.witness as witness
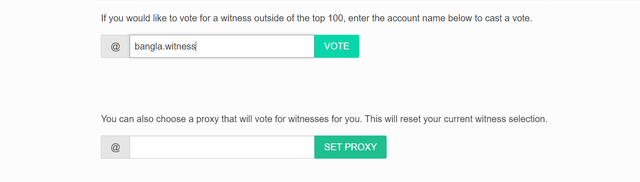
OR
▀▄▀▄▀▄ T̳̿͟͞h̳̿͟͞a̳̿͟͞n̳̿͟͞k̳̿͟͞s̳̿͟͞ ̳̿͟͞f̳̿͟͞o̳̿͟͞r̳̿͟͞ ̳̿͟͞R̳̿͟͞e̳̿͟͞a̳̿͟͞d̳̿͟͞i̳̿͟͞n̳̿͟͞g̳̿͟͞ ▄▀▄▀▄▀