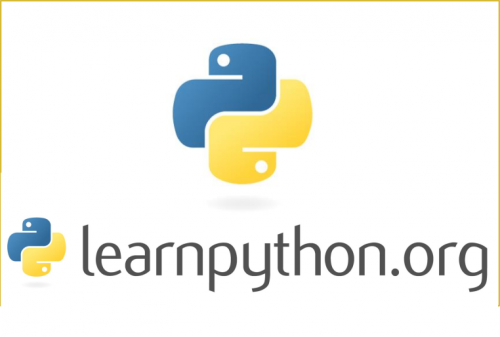
Ray is a new Python framework built out of the inspiration of Flask and Django, the framework is quite young and modern. Their main features are related with the capabilities of the modern web. It supports REST API natively as well as includes a uWSGI, ORM and a database hooks, as well as API protection.
The installation process takes 3 main libraries:
- Peewee ORM
- Ray Framework
- Ray Peewee.
You can install all three through pip by doing the following on a linux terminal:
pip install peewee
pip install ray_framework
pip install ray_peewee
The installations should be able to give you the following
After that we can now proceed to building the app.
Building your first webapp with Ray
The first thing we can do is use the Model post which are the main part of any blog. Creating a model will ensure that we can interact with the database and publish data to a public interface. First we need to create the app.py file with the model post.
First we need to make sure we import our libraries:
app.py
# app.py
import peewee
from ray_peewee.all import PeeweeModel
from ray.wsgi.wsgi import application
from ray.endpoint import endpoint
The next step would be to have our database declared, we will do this by calling peewee and our SQLite database, to a file called example.db:
database = peewee.SqliteDatabase('example.db')
Finally we will start to do some real coding by having our Post model builted in.
class DBModel(PeeweeModel):
class Meta:
database = database
@endpoint('/post')
class Post(DBModel):
title = peewee.CharField()
description = peewee.TextField()
database.create_tables([Post])
First you can see we have 2 classes, one for the database, and the other for the post data, the next thing you will notice is the endpoint which would be the one on the URL.
We can see how we create our post by having 2 fields, title and description. The title field will be a ChrField() and the description a TextField(), finally the database will create the table based on the Post class.
Once we are done with that code we can run it by starting up our framework service, we do this by doing the following:
ray up --wsgifile=app.py
# if you're using virtualenv
ray up --wsgifile=app.py --env <env_dir>
Once launched we can go to our browser and URL localhost:8080. However since we dont have any data yet, we will get a 404 Error.
We can post data using curl through our endpoint.
curl -X POST -H "Content-Type: application/json" -d '{
"title": "New blog!",
"description": "let’s do this"
}'"http://localhost:8080/api/post"
Now we can refresh and found our information, New Blog! and let's do this right there.
Other commands including Delete, List, Update and even Search will be automagically there. Here is an example:
# Listing all blog posts
curl -X GET "http://localhost:8080/api/post/"
# Retrieving one post
curl -X GET "http://localhost:8080/api/post/1"
# Record searching
curl -X GET "http://localhost:8080/api/post?name=john"
# Updating post using CURL
curl -X PUT -H "Content-Type: application/json" -d '{"title": "let’s change \
the title."}'"http://localhost:8080/api/post/1"
# Deleting a post
curl -X DELETE "http://localhost:8080/api/post/1"
And there you go, you have successfully setup your blog using Ray framework.
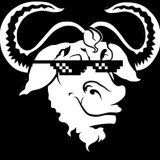
About me:
Steemer, crypto fan, like to listen to 90s hip hop, and loves to chat about Linux Python and Free software. Runs a local Tech club in sunny Cancun, and enjoys hoping on planes and landing somewhere else.