This tutorial will just be an example of a C++ program using multiple files. I will show you one accepted way to organize a program. If you want to check out my other tutorials in this series, here they are:
Part 11: Colored Text in your Terminal
Part 16: Binary, Bitwise Operators, and 2^n
Part 19: Object Oriented Programming 1 -- Data Structures
Part 20: Object Oriented Programming 2 -- Classes
Part 21: Object Oriented Programming 3 -- Polymorphism
Part 22: Command Line Arguments
An Accepted Method
There are many ways to code, but I will go over one accepted method. Say you want to build a program with classes and functions for that class. Start with a header file. In the header file, create your classes with the class members and declare any global variables. Do not set any values or build any functions. We will use a separate file for that.
Here is my header file for a Rectangle program (header.h):
#ifndef HEADER_H
#define HEADER_H
class Rectangle
{
private:
int height;
int width;
public:
void set_height(int);
void set_width(int);
int area();
};
#endif
Now that a header file is made, I will create a separate file (functions.cpp) to build all of the functions:
#ifndef FUNCTIONS_CPP
#define FUNCTIONS_CPP
void Rectangle::set_height(int h)
{
height = h;
}
void Rectangle::set_width(int w)
{
width = w;
}
int Rectangle::area(void)
{
return height * width;
}
#endif
Now that the structure of the program is built, let's implement it by creating the file with main() (main.cpp):
#include <iostream>
#include "header.h"
#include "functions.cpp"
using namespace std;
int main()
{
Rectangle r;
r.set_height(5);
r.set_width(4);
cout << "Area of rectangle: " << r.area() << endl;
return 0;
}
This:
- creates a Rectangle object called r
- sets the dimensions with the functions that were declared earlier
- prints:
- "Area of rectangle: "
- what r.area() returns (which is the area)
Here is the output:
[cmw4026@omega test]$ g++ main.cpp header.h
[cmw4026@omega test]$ ./a.out
Area of rectangle: 20
[cmw4026@omega test]$
- main.cpp included functions.cpp, so it doesn't have to be listed when compiling
I hope this helped you learn how to build a neatly written program! It is always good to code as neatly as possible and leave many comments when working with others. Leave any suggestions in the comments!
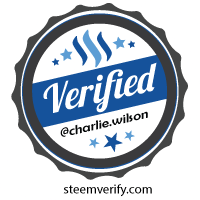