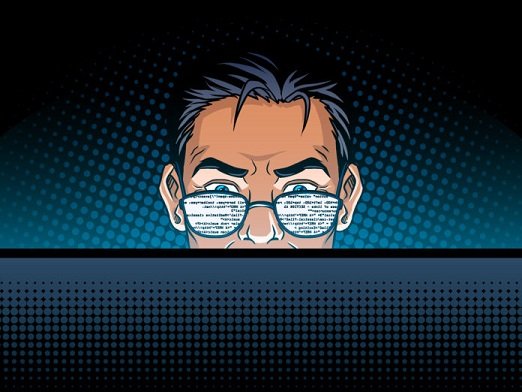
I've completed the backend logic for both Checkers and Reversi (Othello) in Java. I've only played a few test games so there could be bugs, but everything seems to work as intended so far. As one would expect, these games aren't very complicated so maybe there are no bugs, which would be nice for once.
I started with Checkers because I thought it would be the easiest project, but Reversi turned out to be much easier. The reason for this is the movement of pieces. Checkers pieces can move in two directions and they can jump in two directions. Implementing double/triple jump logic was also kind of a pain. King pieces can move in any direction while regular pieces can only move towards your opponent. In Reversi, pieces don't move at all. You simply play a piece and it says in that spot forever, making the programming for it much easier.
Another thing that made Reversi easier to program was that I did it second. I was extremely rusty from not programming for so long. The Checkers project got me back into the swing of things. Also, I made several organizational errors in the first project that I won't repeat in subsequent projects. I learned what the most important aspects of building a board game are.
The number one thing to keep track of when programming a board game, I learned, was cataloging every possible move for every turn. I learned this the hard way. Half way through developing Checkers I wondered, "Hm, how am I going to program the win condition." Turns out, when you dumb it down, the win condition for Checkers is simply the first player who can't move loses the game. In Reversi, the game ends when both players have no more moves. In Chess, the game is also over when a player can't move. Therefore, keeping track of what moves are possible is the #1 priority.
Reversi
Because I haven't programmed a front end for the games yet, this is what it looks like on the command line.
Recursion
Recursion is a technique of programming a function that calls itself, without going into an infinite loop. Normally, you'll program a bunch of stop conditions so the stack rewinds to accomplish a given task. It can be pretty hard to wrap your brain around. If you've ever played Magic The Gathering there is an order of operations called the stack. Actions go on the stack and they resolve in the opposite order that they were put down. Whatever is on the top of the stack gets resolved first and so on until the stack is empty. Recursion works exactly the same way.
I forced myself to write this sandwich() method recursively because it's been so long since I've used recursion. I thought I could use a refresher. I almost gave up on it because it was taking me so long to remember how to do it. However, I think it was worth the effort. I was surprised when I realized that these two methods are pretty much the bulk of the entire game. This is the logic used to figure out which pieces need to be flipped if the given piece (disc1) is actually played. If no pieces get flipped this move is illegal and not added to my list of legal moves.
Rules
I suppose assuming that everyone knows the rules of this game is foolish. In short, if you sandwich your opponents pieces in between your pieces they get flipped to your color. Whoever has the most of their color showing at the end of the game wins. Corners are very important because they are impossible to flip. As you connect sides to the corners they also become impossible to flip. The game is often about forcing your opponent to give you a corner.
I'm actually an expert at this game. I used to play it all the time on Windows XP. Whenever I would go against a Japanese player I would get CRUSHED. I finally got crushed so many times that I figured out the unintuitive strategy behind winning the game. It basically involves allowing yourself to be surrounded at the start of the game. You flip as few pieces as you can and you want the pieces that you do flip to not be exposed. It is in this way that you limit where your opponent can move while also giving yourself as many options as possible.
Here's how it works.
The sandwichAllSides() method simply calls the sandwich method eight times: one for each of the eight directions that pieces could get flipped. There's nothing very special about it and it has nothing to do with the recursion.
sandwich()
This method returns "false" for three different reasons:
- If it goes off the edge of the board
- If the piece it's checking is empty (null)
- If the piece it's checking is the same color and also adjacent (right next to it)
This method only returns true on one condition:
- If the piece found is the same color and also NOT adjacent.
Because the same (not adjacent) color was found we can safely assume that all the pieces in between this piece and the main piece are the opposite color and need to be flipped. The recursion happens when we find a piece of the opposite color.
Example
Say it's black's turn and he wants to move at the marked square. On my game this location would be board[6][4] (board[x][y] where the range of x and y are 0-7 in a two dimensional array using Cartesian coordinates).
So you call the method sandwichAllSides() with this location in mind. It checks all eight directions. Seven of those directions return "false" because they are blank and have no pieces on them. The last direction returns true after several mental gymnastics. That direction is sandwich() where deltaX = -1 (because it's going backwards) and deltaY = 0 (because it's not going up or down)
First call of sandwich() (deltaX = -1)
The recursive function finds a white piece, which is exactly what it was looking for. The problem is we don't know if this white piece is actually sandwiched by a black piece. It could still run off the board or hit a blank space, in which case it should still return false;
This is where recursion comes in. You leave this current function open on credit (so to speak) and make a recursive call that will eventually return the correct answer. This is the first item on the stack. Now instead of calling the sandwich function with deltaX = -1; we call it with deltaX = -2;
if( sandwich(disc1, deltaX, deltaY, flip) ){
Recursion has begun. This method gets put on hold to be resolved later when we find the answer. Is sandwich() deltaX = -2 true or false?
Second call of sandwich() (deltaX = -2)
We found another white disc. Which means yet again we don't know the answer. Let's check the next one.
Third call of sandwich() (deltaX = -3)
Another white disc. Try again.
Forth call to sandwich() (deltaX = -4)
OMG a black disk. We can finally return true, but what happens then?
REWIND!
We've called the sandwich() method four times. There are four copies of this method on the stack waiting to be resolved. This fourth call resolves first and returns true immediately.
if( sandwich(disc1, deltaX, deltaY, flip) ){
// sandwiched piece found
if(flip)
disc2.flip();
return true;
}
The forth returns true to the 3rd method call. It flips the disk and returns true to the second method call, which flips the disk and returns true to the first method call, which flips the disk and returns true, signalling to the original call that at least one disk was flipped and this is a legal move.
Conclusion
Recursion is a complicated process that you really have to wrap your brain around to get a good understanding of it. However, once you add this tool to your programming toolbox it can make very difficult problems to solve seem trivial. I don't expect I've helped anyone by writing this. You're either not a programmer, or you are and you already know what recursion is. This is mostly just for me to cement my understanding of this useful technique.
So, why am I even bothering with programming these old school classic games in the first place? Well, first of all, if you want to program games (I do) there is no excuse to not take some times to create the most basic ones. Also, if I end up putting these games on the blockchain you'll be able to bet on the outcome, which could be fun. Obviously, you'd have to fully trust your partner and bet very small amounts because cheating is extremely easy. All of these games have been solved by AI. AI can destroy the best grand-masters so you have to be sure your opponent won't use AI to cheat.
I'm actually really surprised the basic games have not been put on the blockchain. No one in the world seems to have done it yet. I find this very odd. Whenever I look for reversi apps on Android or the web I have a very hard time finding ones that are two player; they are all against the computer (boring!). Blockchain tech provides me a way to bring these two-player games to the entire world without even having to pay for a server; while also being connected to ultra secure accounts and ultra secure cryptocurrencies.
Another big reason why I'd like to put these games on Steem is to show the Steem developers how flawed the current system is. Right now, you can only comment on the blockchain every 20 seconds, meaning that if I put these games directly on the chain you'd only be able to move at the fastest once every 20 seconds. This is unacceptable, which is why I wrote this post about upgrading the account @null to allow for text information to be posted to the blockchain on every block. This would allow bot's to post to the chain every three seconds while not allowing them to clutter the rest of the site.
I have to decide whether to do Chess or Go next. It probably doesn't matter much considering the speed at which I was able to complete the other two. However, a lot of people don't even know about Go because it's more of an Eastern game. It's pretty amazing though and is much more complicated than chess, even though the rules are simpler and there is only one type of piece.
Once I've completed the four games I'll create a frontend module to start connecting them to different blockchains. I'll either start with Tron or Steem. Tron claims to use Java as a main language, but Steem already has a built in community. With Steem, I'll likely use this project as a reason to learn JavaScript and Node.JS and transfer the logic over to those platforms. Eventually, I'll probably even make a simple Android app to play all four games. If you know of any other board games you'd like to see on the blockchain let me know. Settlers of Catan is definitely on the table.
Holy mother of god programming sounds so hard and so fun at the same time!
Check out the board game carcassonne if you thinking of programming games, i played it some years ago with my brother and it's a lot of fun! And, even though i don't know much about programming i think it won't be that hard to programm. The game has a huge following and there are even some expansions to get more pieces into the game!
BTW, how do you manage to program, write and still work at the same time? that must be so hard! And your posts are always top notch!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
I work a shitty near minimum wage job 15-25 hours a week. Like a boss.
I totally forgot about Carcassonne. That is an amazing suggestion... I already own that game and know it very well. It's been a long time since I've played my board games with anyone.
Programming is fun. The kind of fun that makes you want to tear your own hair out. It is puzzle solving in the language of computers. If you like puzzles and math, you'll probably like programming.
The problem with programming is corporate imperialism. It takes a big team of programmers to get profitable projects completed. Who's going to front the bill for all those high salaries and big risks? THE MAN! So, they play their little game of, "I bet I can pay you less than you're worth!"
Meanwhile, the job is very high paying, so people that should not be considering the job want to jump in on it, creating a bunch of people who hate their job and are bad at it. Now the people that like their job and are good at it get forced to work with the rest of the tools. It's a team effort, and can be extremely frustrating when your team is incompetent.
I'm not speaking from experience. I've never had a real job :D I hope that one day soon™ the blockchain figures out a superior decentralized method of creating projects that people actually want to create while getting paid what they deserve. I think this would be the ultimate killer app that spawns all the other killer apps of the blockchain. Kind of like AI singularity.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
That would be amazing! don't know how my vet degree would come in on that but i'm sure someone would come up with an idea!
BTW you must have a raging boner with the TRX spike right? 8% in a more or less stable market is pretty good!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
The market has calmed down so much we get all excited when it jumps 10%-20% haha. NO!!! WHERE'S MY 100% damnit! :D Hopefully the announcement delivers.
One thing I think is very important is abstracting the puzzle of programming away from programming. I want to create games where we can use Steem's proof-of-brain concept to pay people to help develop, without having to know a lick of programming.
For example, my Cards Against Humanity clone where anyone can make their own deck. Also, I have an idea for a turn based RPG where anyone would be able to design their own classes and monsters to fight in a sandbox. All the good scenarios would get incorporated into the game and those developers would get paid for their good work. I think a bounty system is key.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Well, that turn based rpg sound awesome! but also it might be incredibly hard to balance!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
I agree, but I also think that community consensus is much more valuable than a development team when trying to balance a game. I assume such a thing has never happened before so I base these assumptions on absolutely nothing.
If you look at games like League of Legends and World of Warcraft and other RPGs, new classes that get introduced to the game are always extremely overpowered. This is a tactic to get people excited and buy the game. Money and profits are always out to ruin game-play. I think a decentralized environment could sidestep problems like this and identify major flaws much quicker than a small development team could.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Yes maybe, imagine if League of Legends wasn't in the hands of riot... we all know the new champs are mostly made to be overpowered at the beginning so everyone buys it with RP... Maybe decentralizing the process of balancing a game would make it much much better and faster, but in the end, i think giving the balancing of a game to an AI would make it more balanced...
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
I think ideally an AI could never play the game as well as a skilled player. That's another thing I've thought a lot about: how to stop bots from undermining the entire system. In a transparent environment where everyone can see what everyone is doing I don't think this would be very hard, and it would increase the value of being a human player by 100 fold. We have to break away from the menial pointless tasks (like farming herbs in WOW) and reward players for actual proof-of-brain. This way bots will have a very hard time keeping up with gameplay. If the game is constantly evolving the chance that a bot will be able to keep up is very low. I've even thought about utilizing fingerprint scanning on smartphones and/or facial recognition to stop botters.
I also want it to be high stakes. I always loved playing Diablo 2 on hardcore mode. Even though I played on a 56k modem and died all the time to lag like a crazy person. I think my ideal game would also involve perma death with PvP on top of that with a crypto auction house. With stakes that high bots would get shredded.
My philosophy is use AI to automate out the shit jobs that nobody wants. I think game balancing is rewarding enough that people would want to do it. No sense in trying to automate it out.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
@edicted always good content. Your post was selected and voted by the curator @pataty69 project looking for excelent rewards on great content posts that can be followed on my trail at Steemauto.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit