Is this C program valid? What does it do?
#include <stdio.h>
int main( int argc, char *argv[] ) {
(*puts)( "foo" );
(&puts)( "foo" );
(***puts)( "foo" );
(*&**&puts)( "foo" );
}
Surprisingly, this is perfectly valid C. The authors of the ANSI C specification decided there wasn't anything reasonable you could do with a function pointer other than dereference it, so there is no difference between a function and a function address. All of the above lines have exactly the same meaning, which we can see using the Compiler Explorer: https://godbolt.org/z/UmaKcM
main: # @main
push rbp
mov rbp, rsp
sub rsp, 32
movabs rax, offset .L.str
mov dword ptr [rbp - 4], edi
mov qword ptr [rbp - 16], rsi
mov rdi, rax
call puts
movabs rdi, offset .L.str
mov dword ptr [rbp - 20], eax # 4-byte Spill
call puts
movabs rdi, offset .L.str
mov dword ptr [rbp - 24], eax # 4-byte Spill
call puts
movabs rdi, offset .L.str
mov dword ptr [rbp - 28], eax # 4-byte Spill
call puts
xor ecx, ecx
mov dword ptr [rbp - 32], eax # 4-byte Spill
mov eax, ecx
add rsp, 32
pop rbp
ret
.L.str:
.asciz "foo"
The &
and *
operations have no effect at all on a function pointer. You can apply as many as you want.
Don't try this, though:
(*&&*puts)( "foo" );
C's lexing rules mean that && is parsed as the logical AND operator, not two "address of" operators.
Source: https://www.quora.com/In-C-is-it-possible-to-pass-a-function-to-another-function/answer/Petr-Skocik
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
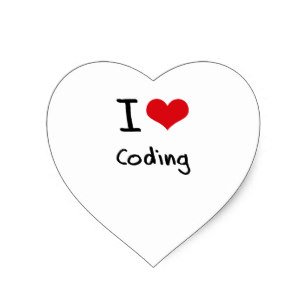
Reply !stop to disable the comment. Thanks!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit