In this section, we are going to have an overview of the character arrays in C. In addition, we are going to familiarize ourselves with the concept of external variables and scope.
Character Arrays
The array of characters is the most common type of array in C. We will explore the array of characters and some functions to manipulate them by writing a program that reads a set of text lines and prints the longest.
Our outline is as follows:
This gives us a clear overview of how to divide the program into functional pieces: one piece gets a new line, another tests it, another saves it, and the rest controls the process.
while (there's another line)
if (it's longer than the previous longest)
save it
save its length
print longest line
This gives us a clear overview of how to divide the program into functional pieces: one piece gets a new line, another test it, another saves its, and the rest controls the process. The whole algorithm written in C is given on the table below,
Main Functions | Intrinsic Functions |
---|---|
![]() | ![]() |
It is shown that we have 3 functions to discuss: main, getline, and copy.
main and getline communicate through a pair of arguments and returned a value.
- getline are declared by the line:
o first argument s is an array
o second argument lim is an integer holding the max size of the array
o getline returns a value counting the length of the line
o getline is declared to return a int - copy function is declared as:
o returns a void value which states that no value is returned
Convention: when a string appears in C program, it is written in an array of characters containing the characters of the string and is terminated with a ‘\0’ to mark the end. Thus for a string like,

a string of character conversion to an array of characters ended with a '\0'
The %s specification in printf expects the corresponding argument to be a string.
External Variables and Scope
To demonstrate the concept of external variables and scope, consider the following program:
Main Functions | Intrinsic Functions |
---|---|
![]() | ![]() |
The variables in main, e.g. line, longest, etc, are private or local to main in our previous program; that is, no other function can access them because they are declared within the main function. Similarly, the i in getline is different from the i in copy.
Local variables are summoned only if the functions they are in are called and eventually disappear as the function execution is terminated. These local variables are also sometimes known as automatic variables.
(There is an object in C known as the static storage class, in which local variables do retain their values between calls.)
There is however a variable in C that can be accessed by name by any function – a variable external to all functions. So, instead of using arguments, we can use these external variables to communicate data between functions, since they are globally accessible.
Interestingly, since these external variables remain in existence even after the function call, they are capable of retaining their values.
External variable must be defined, exactly once, outside of any function; this set aside storage for it. The declarations maybe an explicit extern statement or may be implicit from context.
Declaration:
External definitions are just like definitions of local variables, but since they occur outside of functions, the variables are external.
Declaring the external variable | Using the external variable |
---|---|
![]() | ![]() ![]() ![]() |
Calling/Using:
Before a function can use an external variable, the name of the variable must be made known to the function. You also have to write an extern declaration in the function, it’s the same as before but with added keyword extern.
If you have several source files, the usual practice is to collect extern declarations of variables and functions in a separate file, historically called a header, that is included by #include at the front of each source file. (.h is conventional for header names)
Definitions vs. Declarations
- Definitions: refers to the place where the variable is created or assigned storage
- Declarations: refers to places where the nature of the variable is stated but no storage is allocated
When using external variables, one must be careful in using it if the data connections in the program are not at all obvious.
Disclaimer: this article is a summary of section 1.9-1.10 from the book The C Programming Language (ANSI C): by Brian Kernighan and Dennis Ritchie, the content apart from rephrasing is identical, and the same line of codes are treated.
Thank you for reading. copyright 2018 by @sinbad989
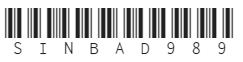
Hi! I am a robot. I just upvoted you! I found similar content that readers might be interested in:
http://www.java-samples.com/showtutorial.php?tutorialid=492
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
This post has received a 8.16% upvote from @msp-bidbot thanks to: @sinbad989. Delegate SP to this public bot and get paid daily: 50SP, 100SP, 250SP, 500SP, 1000SP, 5000SP Don't delegate so much that you have less than 50SP left on your account.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit