When a class has just a single parameter constructor, the compiler is allowed to make an implicit conversion to resolve the parameters to a function. It is used to convert from one type to another in order to get the right type for a parameter.
For example, if we have the class Foo that has a single parameter constructor that receives and saves an int foo:
class Foo
{
public:
Foo (int foo) {m_foo = foo;}
GetFoo () {return m_foo;}
private:
int m_foo;
};
And here is a function that takes and prints a Foo object:
void DoBar (Foo foo)
{
int f = foo.GetFoo();
cout << "DoBar: " << f << endl;
}
So, if DooBar is called like:
DoBar (12);
DoBar (22.6f);
DoBar ('A');
In each call of “DoBar”, the parameter is not a Foo object. In the first call is an int, the second is a float and the third call is a char. Though, there exists a constructor for Foo that takes an int so that constructor can be used to convert the parameter to the correct type. This example compiles and prints the following result:
DoBar: 12
DoBar: 22
DoBar: 65
Prefixing the explicit keyword to the constructor prevents the compiler from using that constructor for implicit conversions. Adding it to the above class will create a compiler error at the function call DoBar.
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
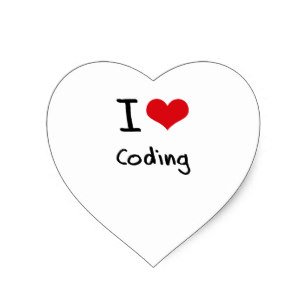
Reply !stop to disable the comment. Thanks!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hi! I am a robot. I just upvoted you! I found similar content that readers might be interested in:
https://stackoverflow.com/questions/121162/what-does-the-explicit-keyword-mean
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit