Python is a dynamic language that does not require you to specify data types as you create variables or supply return types. This makes development a lot easier but can introduce unexpected bugs when things don't go as expected.
Typed languages help prevent bugs by making sure strings are strings, and ints are ints when they need to be. This can save hours of debugging when suddenly something doesn't work when it used to in the past.
While Python doesn't require types, 3.5 added the option to specify a type. This allows Python IDE's like PyCharm and linters to provide better insight into your code and warn you of type misusage.
While the syntax in Python 3.5 works, it depended on comments which don't always display properly in different editors for this sort of thing. 3.6 took it further and added a specific syntax that displays better in editors that support it.
I will not cover the 3.5 Syntax as you should be using 3.6 if you are using Python 3.
Type Annotation in Python 3.6
In 3.5 you could type hint lists and dicts and this hasn't changed in 3.6.
No type hinting
employees = []
Type hinting
employees = List[str]
The type hinting specifies a list of strings. Now nothing will stop you from putting an int or a float in there, everything will work perfectly fine but if your business logic expects strings and gets a float your code may break without any previous warning.
Using an IDE like PyCharms would have warned you that there was a float in your list of strings. You can also use mypy type checker or any number of code linters.
All the above code is compatible with 3.5, when we start dealing with variables that are not strings or dicts is when the syntax changes between 3.5 and 3.6. The below code only works with 3.6.
No type checking
employee = 'Bob'
Type checking
employee : str = 'Bob'
While this seems like a simple change, it can prove extremely useful when your code suddenly breaks for no apparent reason.
The syntax is simple
variable : [TYPE] = [VALUE]
The only change is adding a : and a [TYPE] to your variable declarations. You can verify the type with type(). If you are not using a code linter, I highly suggest you do. I will cover linting in a future post.
Wow! This looks amazing but I'm confused. As much as i like programming, i feel the process is really strenous.
I like that all the codes put together just form an awesome outcome.
LInes like this
Just made me more confused😭😭 this is not for me. Kudos to programmers. Y'all deserve some accolade
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
mate, apologies for hijacking another of your incredible posts about snakes, but as the no.1 abuse blocker here, could I just draw your attention to this screenshot please. Perhaps you might like to investigate further via his blog shown on the pic...this is the 4th or 5th time hes bought a lot of accounts this way.
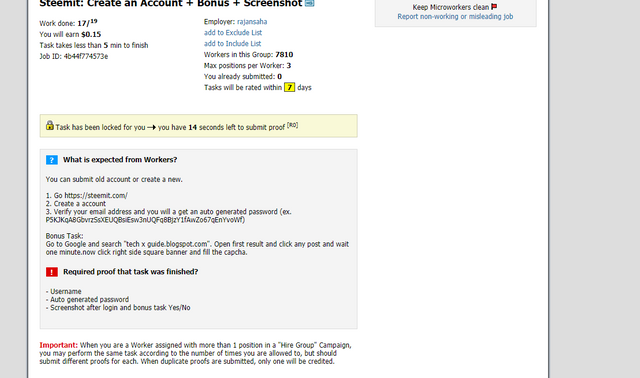
The site is microworkers and there is more and more of this shit going on there including circle jerking circles and paid for upvotes to win competitions etc.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
A most perfect idea @themarkymark
A meaningful learning in doing pemogramman Something that can ease to accelerate the activities we do in terms of typing using Python is easy for us to do our activities.
Thank's @themarkymark it important step
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Ooh, I like it. Coming from strongly typed languages, it has been a debugging worry of mine :)
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Good to know about those annotation. Thanks for sharing. If you have some time then please take a look to my open source app I made for Steemit’s user. It’s about steemit recent posts feed widget.
Steemit recent post widget
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
To be candid, the more i read more my head goes into the jungle. I thought you were talking about the snake python that we all know until i started reading programming. 😯
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Yes, we welcome such programs that serve many colleagues and friends
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Woke up, looked at steemit feed....did not expect to see a post on python. Not a developer and the time I last used that language was in Uni. :) Anyway, your post made me wonder why I wanted to relearn a while ago, because I had a friend who kept insisting I should learn ruby instead. I’ve only ever needed to use JavaScript for work. Anyway, no time for learning a new coding language atm, but nice little reminder.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
If you already know one language, other will not be as hard to learn.
That said, classical JavaScript is used in the browser and therefore a little different in its usage. Python, Ruby and JavaScript on Node are pretty similar though.
Not having time is a bad excuse. "Translate" one concept you know from one language to another each week and you will be a pro in no time ;)
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
‘Time’ is a valid excuse. My days are filled with configuring software and current system does not need me to customise existing JavaScript anymore. I’d need an excuse to learn Python. It’s been on the back of my mind it’s a useful language for years, but current interests that take my free time is writing. Maybe when I give myself a programming challenge, I’ll look into the Steemit development side of things as a different focus. That interests me, but I just need time.
Thank you for the encouragement though. :)
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
First, I would like you to notice that you say 'Time' is the problem but then go on and elaborate on the fact that you lack the right kind of motivation. In fact you imply it yourself: If you were motivated enough, you would MAKE the time.
Second, challenging your mind to focus on a specific thing for about 3-5 minutes a day has a major impact on your over all ability to concentrate on your task. I do that every day and it does not feel like work. I feel relaxed after I do it.
That said, I have stacked up to 10 minutes a day and what I think avout varies a lot. Might as well be some programming concept or software architechture principle.
It is not about building a wall, it is about laying each stone perfectly. Someday you will step back an wonder where the wall came from.
What I am trying to say is that my motivation comes from within myself, not from my boss or the challenges my work poses me - well, for the most part anyway.
On the other hand I am not an expert. Just some random happy dude on steemit :D
Have a good one. Bye!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hi @themarkymark. I've choice you as one of my witness vote
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thanks :)
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
I do "C" and Assembler but really fight with this high-level languages.
There also too much changes with the different versions which really sucks.
Maybe I finally get used to it now using "beem" library. This stuff force me using python.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Ohhh okay cool this is good, I'm a Python n00b so I had no idea about the difference in syntax between 3.5 and 3.6.
I was using Intellij for awhile but I think I broke it and somehow Pycharm was giving me issues as well so now I just use IDLE and so far it's been pretty great.
I tried rocking your example in that and it worked perfectly
(well, except for the missing = after str heh 😅 ):
Thanks for posting this, @themarkymark!
Will work my way through the rest 👍
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Cool series you have here. Are you going to do any tutorials on DTube?
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit