Assalamualaikum my fellows I hope you will be fine by the grace of Allah. Today I am going to participate in the steemit learning challenge season 21 week 3 by @sergeyk under the umbrella of steemit team. It is about strings in C. Let us start exploring this week's teaching course.
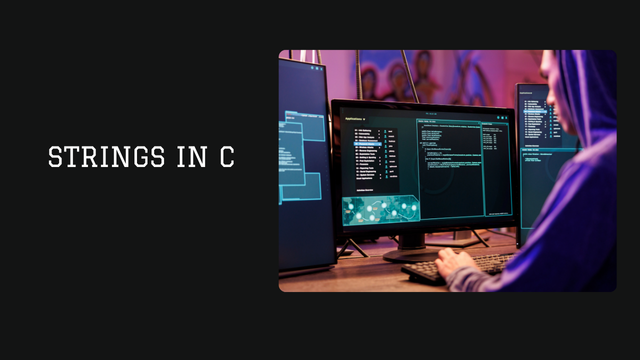.png)
Demonstrate how to make it look like the array size can be increased/decreased. All loops should work with the array size stored in the size variable. Keep the physical, actual size in the constant N.
To show the increasing or decreasing size of an array in C++ we can use two concepts.
- Logical Size: This is the "visible" size of the array. We control it with a
size
variable. - Physical Size: This is the actual maximum size of the array which is defined as a constant
N
.
So by maintaining logical and physical size we can simulate the dynamic behaviour. If logical size is less than the physical size then it means we can add elements in the array by increasing size
. Similarly when we shrink the array the size
is reduced but the physical array size remains constant.
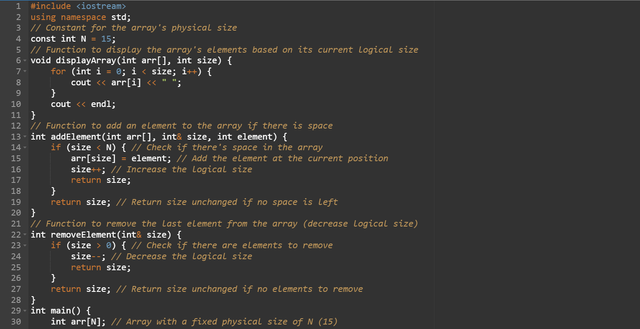
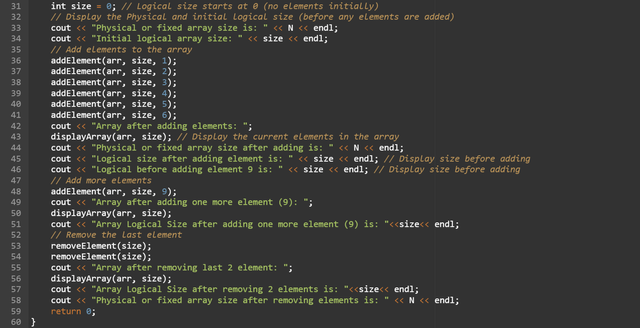
To perform and show how the array size increases or decreases while adding any element in the array and similarly while removing any element from the array I have created these functions in the program.
addElement
Function: This function adds an element to the array ifsize < N
. This increases the logical size bysize++
and it does not modify the physical size.removeElement
Function: This function decreases the logical size bysize--
to simulate the removal of an element but it does not delete the actual data from the array.displayArray
Function: Obviously we need to display the data on the screen as output so I have added This function to display the array. This displays elements up to the logicalsize
instead ofN
by showing that the array dynamically grows and shrinks.

The program shows how the array grows as elements are added and shrinks as elements are removed while the actual physical array size remains fixed. This is achieved by adjusting only the logical size (size
). If we see carefully at the start the physical size of the array is 15
becausde it is predefined and constant but the logical size of the array is 0. This is because there is no any element in the array.
Then I have added some elements in the array and the logical size of the array has become 6 but the physical size is fixed which cannot be changed. It means the total size of the array is 15 and we can increase the logical size to this extent. I have added one more element and the logical size has increased to 7. Then I have removed 2 elements from the array and due to which the logical size had decreased to 5 but still the physical size if 15. It is how the size of the array increases and decreases.
Declare a string variable (store any sentence in the array). Task: reverse the string, i.e., write it backward. For example: char s[]="ABCDEF";.....your code.....cout<<s; => FEDCBA
This looks like the real world problem as in the real life if we want to reverse some characters or things we change their position by swapping them with each other. Similarly in programming we swap the characters of the string to reverse the order. But this swap happens from the beginning and end of the array while moving towards the center. Because while reaching towards center all the characters will be reversed. So in this way we efficiently reverses the string in place without using additional memory.
Here’s the C++ code to reverse a string:
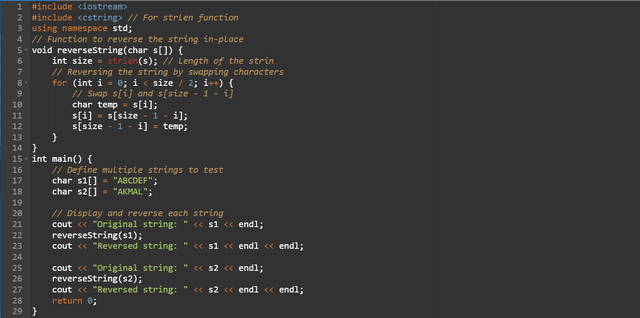
To reverse the string characters in the array I have used the following functions.
- Get the Length: First of all I have used
cstring
library to usestrlen(s)
to get the length of the string. - Swap Loop: There is the swap loop from the start of the string to the middle. For each character
s[i]
at the beginning we swap it withs[size - 1 - i]
at the end. And in this way all the characters in the string are swapped. The loop scans the array only up to the middle because each character is swapped with its corresponding character from the opposite end. Once the first half of the string is swapped with the second half then the string is fully reversed. Scanning beyond the middle would lead to redundant swaps effectively undoing the reversal. In other words after the middle point all characters have already been swapped so no further action is needed. This makes the algorithm efficient by reducing unnecessary work. The time complexity of this algorithm isO(n)
wheren
is the length of the string. So we only loop through half of the string - Output: After completing the loop the string is reversed in place. We then print the reversed string.

This code reverses any given string stored in a character array as required. I have passed an empty array of characters so that we can use any string and pass it to the function.
Swap neighboring letters char s[]="ABCDEF";.....your code.....cout<<s; => BADCFE
We can swap neighbouring letters in the string by iterating through the string with a step of 2. And in each step two adjacent characters are swapped. This approach helps to effectively swap neighbouring pairs.
Here's how to implement this in C++:
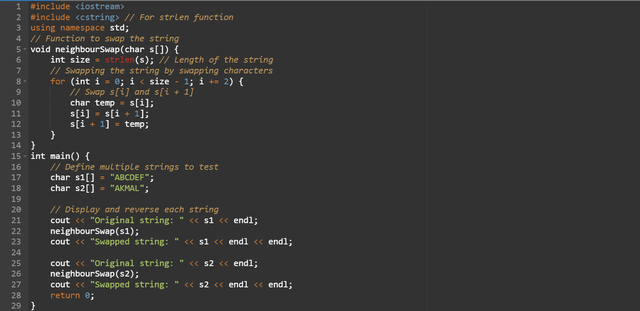
Explanation
- Get the Length: To get the length of the string by using
strlen(s)
I have importedcstring
library. I have accessed the length of the string. - Swap Loop: There is a swap loop which iterates through the string with
i += 2
. Andi
takes values 0, 2, 4, etc. For eachi
it swapss[i]
withs[i + 1]
. This ensures that only neighboring letters are swapped. The loop is stopped before the last character (size - 1
) of the string if it has an odd length to avoid an out of bounds error. - Output: In the
main
I have called the swap function and by this the neighboring letters have been swapped and we print the modified string.

This code achieves the desired output by swapping neighboring letters as specified. The function neighbourSwap()
swaps each pair of neighbouring characters in the string. In the string "ABCDEF"
the function is swapping A
with B
, C
with D
, and E
with F
to produce the string "BADCEF"
. Similarly by using this logic the function has converted AKMAL
to KAAML
by neighbouring swap.
Shift the string cyclically to the left (it’s easier to start with this), then cyclically to the right. char s[]="ABCDEF", x[]="abrakadabra";.....your code.....cout<<s<<"\n"<<x; => BCDEFA aabrakadabr
We can shift the string cyclically. These are two different cases to shift the string cyclically to the left or right.
CASE-I: For the left cyclic shift each character is moved one position to the left side and the first character is placed at the end.
CASE-II: For the right cyclic shift each character is moved one position to the right side and the last character is placed at the start.
But here you can see that we are moving in the different directions depeding upon the nature of the shift. The key difference between left cyclic shift and right cyclic shift is because of the direction in which the elements are shifted. This is the reason one loop runs forward (left shift) and the other runs backward (right shift). Here is the further explanation to understand it:
Left Cyclic Shift: We start from the leftmost character and move towards the right because each character needs to shift one position left. After shifting the first character should end up at the last position.
Right Cyclic Shift: We start from the rightmost character and move towards the left because each character needs to shift one position right. After shifting the last character should end up at the first position.
Here’s how to implement both in C++:
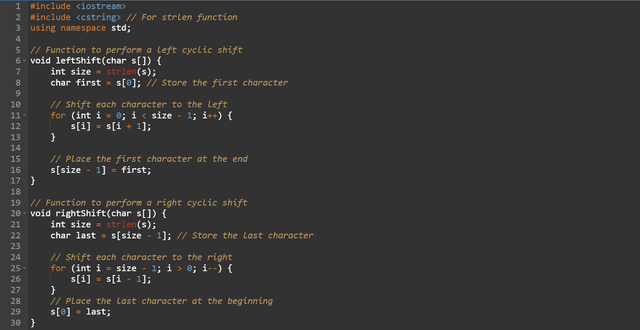
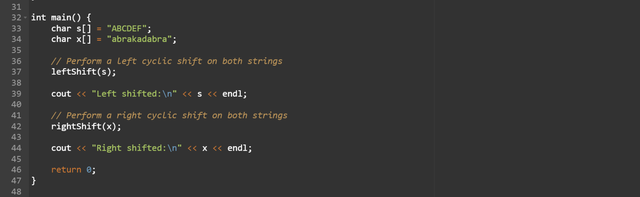
Explanation
leftShift
Function:- The program stores the first character in a temporary variable which is
first
. Then each character is shifted one position to the left side sos[i] = s[i + 1]
. After the loop thefirst
is placed at the last position such thats[size - 1] = first
.
- The program stores the first character in a temporary variable which is
rightShift
Function:- In this function of right cyclic shift it stores the last character in a temporary variable which is
last
. Then we shift each character by one position to the right side sos[i] = s[i - 1]
. After the loop thelast
is placed at the first position such thats[0] = last;
.
- In this function of right cyclic shift it stores the last character in a temporary variable which is
Example Output
The output for char s[] = "ABCDEF"
and char x[] = "abrakadabra"
will be:

I have implemnted left cyclic shifting for the first string s
where ABCDEF
has changed to BCDEFA
. And similarly I have implemented right cyclic shifting on the string x
where the string abrakadabra
has changed to aabrakadabr
.
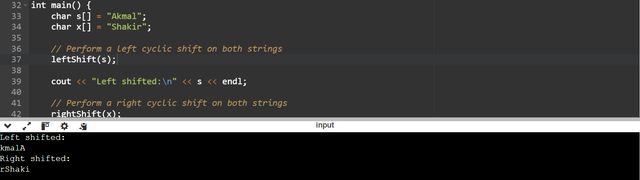
Now I have changed the value of the string s
to s[] = "Akmal";
and similarly string x
to x[] = "Shakir";
. Now the first string Akmal
has been shifted cyclically to the left and it has changed to kmalA
. And the second string Shakir
has been shifted cyclically to the right and it has changed to rShaki
. So this is how we can shift the strings cylically to the right and left.
Remove all vowel letters char s[]="this is some text";...your code...cout<<s; => ths s sm txt
To remove all vowels from a string in C++ we can iterate through each character and skip any vowels we encounter. By shifting only the non vowel characters we can modify the string in place to remove vowels. In this way we will have no need to need of any extra array because this sifting will be done in place.
Here's how to implement this:
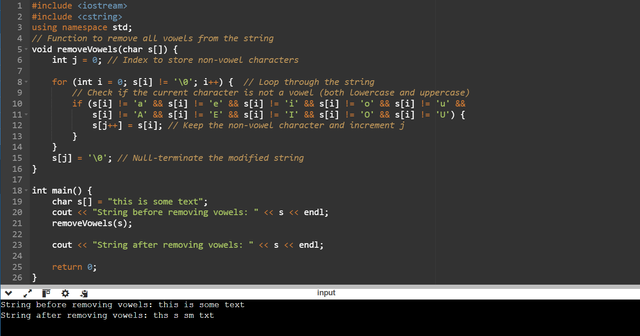
Explanation
- Loop Through Characters: We loop through each character in the string to find the vowels.
- Check for Vowels: For each character
s[i]
we check if it is a vowel or not a vowel. It checks both the lowercase and upper case letters to detect the vowels. - Store Non Vowel Characters: If the character is not a vowel we store it in the
s[j]
position and incrementj
. - Null-Terminate the String: After the loop we place a null character (
'\0'
) ats[j]
to end the modified string.
Here in this program we have used two pointers i
and j
where i
loops through the original string and j
tracks that where the next non vowel should go in the string. It means we never overwrite any part of the string. We are simply moving non vovwel characters to the new positions and when we have done it the remaining loop is null terminated safely.
Example Output

If we see here I have given input string as this is some text
. In this string i
in this
, i
in is
, o
and e
in some
and e
in text
are the vowel letters. If we remove all the vowel letters from this we should get the output as ths s sm txt
. And similarly the program has removed all the vowels correctly from the string and it has become ths s sm txt.
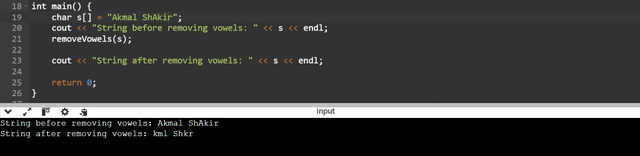
Here I have taken another example of Akmal ShAkir
. You can notice I have taken an upper case vowel A
in the string to test if this program detects the uppercase vowel and rmoves it or not. But you can see the program has detcted the lower as well as upper case vowels from the string and it has removed them. After removing the vowels from the string the program shifts the non vowels to the left side.
In this way we can remove the vowels from the strings either they are in the lower case and upper case.
Double each vowel letter char s[]="this is some text";...your code...cout<<s; => thiis iis soomee teext
It is similar with the previous one with a slight difference. In the previous we found the vowels from the string and removed them. In this question we again need to find the vowels but here except to remove we need to double the found vowel in the string.
And if we talk about the solution of this problem then we can double each vowel letter in the string by checking each character in the string if it is a vowel or non vowel. If we find vowel in the string we can add one more in that place.
Here’s the C++ code to achieve this:
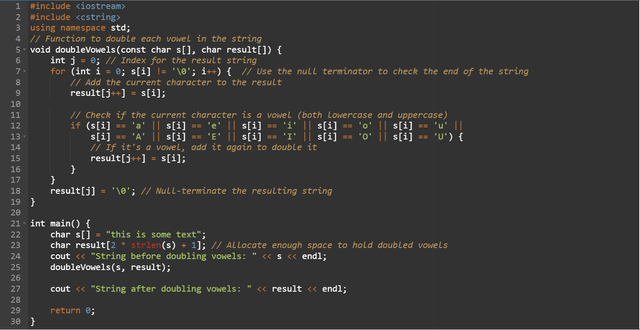
Explanation
- Loop Through Characters: We iterate through each character in the array
s
. - Copy Non Vowel Characters: While traversing the loop for each character
s[i]
, we first add it toresult[j]
. - Check for Vowels: If
s[i]
is a vowel we add it toresult
again immediately after the first copy. - Null Terminate the String: After the loop we add
'\0'
to mark the end of theresult
string. - Enough Space: As after doubling the vowels the size of the string increases and to adjust the size I have used
result[2*strlen(s)+1]
. It will allocate enough space to hold doubled vowels.
Example Output

I have taken s
string as this is some text
. In this string i
in this
, i
in is
, o
and e
in some
and e
in text
are the vowel letters. And we need to double these letters and after doubling these letters they should become thiis iis soomee teext
. And finally after iterating through the whole string and finding teh vowels the rogram has returned the desired result.
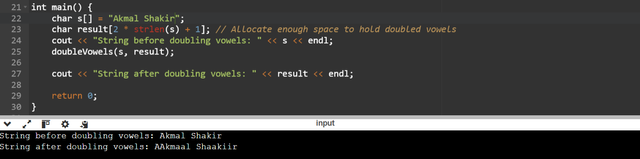
In order to test it with another example I have changed the value of the string s
to Akmal Shakir
. The program has successfully added one more vowel with the already existing vowels. And after doubling the vowels in the string it has become AAkmaal Shaakiir
. One more thing which I noticed that while doubling it doubles the vowels acccording to their initial case. If the vowel is in upper case then it will add another vowel in the upper case and wise versa for the lower case.
It was really very interesting to explore the strings with different methods.
I invite @suboohi, @irawandedy, and @chant to learn strings.
I have compiled all the code of the tasks in a famous online compiler which is OnlineGDB
First of all I want say a big thanks to the steemit team for bringing these learning challenges free of cost and supporting the participants.
I am confuse in something. I got 40% upvote from @steemcurator01 and it became around 31.59 STU.
But another account which recieved 29% upvote and its value became 39.78.
Is it because that my post is near the payout?
Sorry in advance while disturbing you @steemitblog, @steemcurator01, @steemecurator02 but I need some information about this. Means if my post is near to payout then the upvote value will be low and can we overcome this problem by providing any new post instead of that which is about to expire in the next few hours?
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit