Hello everyone! Today I am here to participate in the Domain Steem with JavaScript course by @alejos7ven. It is about learning the Broadcasting operations part 1: Transferring, and posting. If you want to join then:
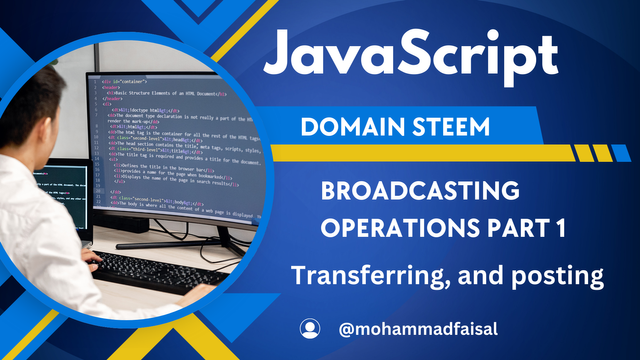
Create a bot that detects when you vote for someone else's publication, when detecting your vote creates an automatic comment on that publication you voted for (the app that created your comment should be called your username and version 1.0, example app:'alejos7ven/1.0')
Creating a bot to detect the vote of my account and then to post comment on the voted posts is looking very interesting and I am feeling confident to make it by using the Steem JS library. In the previous lessons I learnt about the streamOperations
and it is used to detect the real-time transactions happening on the blockchain/. As the votes are the real-time transactions so first I will try to detect the vote cast and then I will initiate the commenting operation to publish my comment under the post where I have voted.
Here is the complete code for this bot which is able to detect the vote and then post comment after the vote on the voted post. I have hidden the part where my private active key was used. Here is the explanation for this bot:
Here you can see that at the start I have imported Steem library to perform the operations on the steem blockchain. Then I have declared two more constants:
steem_username
which stores the Steem username for which the bot will work. In my case I have given my steem username asmohammadfaisal
.posting_key
is where we need to place the posting private key of the Steem account. This key is required to broadcast actions such as voting and commenting to the Steem blockchain. I have not shown my private key because of the security reasons.
createComment
Function
This is the major function of this code which creates comment based on the pre-defined provided data and then broadcasts the comment. There are unique things in this function. Here is the further detail of this function:
commentPermlink
: This generates a unique permlink for the comment by concatenating"mohammadfaisal"
with the current timestamp (Date.now()
). In this way my comment will be unique with the fixed keyword in the link.body
: The body of the comment is defined here. It contains a greeting message mentioning theauthor
. I have added a note about voting on the post and information that the comment was generated by a bot.jsonMetadata
: This is the metadata for the comment. It is encoded as a JSON string. In this there aretags
for the comment. We can also comment without using these tags but I added my unique tags for the comment because there was the option for it. At the ed I have provided the information about the app and its version.steem.broadcast.comment
: This function sends the comment to the Steem blockchain. It requires the following data for the comment posting:posting_key
: My private posting key which I have already provided at the top in the variable and now I have used that variable here.author
: The original author of the post at which the comment will be added.permlink
: This will fetch the permlink of the post on which my comment will be added.steem_username
: This is my username from which the comment will be done.commentPermlink
: This is the unique identifier for the comment.""
: This is the title which is empty for comments.body
: This holds the content of the comment.jsonMetadata
: This is the metadata for the comment.
After running the bot if the comment is successfully posted then it will print a success message. On the other hand if there is an error then it will print an error message with the relevant information.
Listening for Blockchain Operations (streamOperations
)
This is the function to listen to the blockchain for the detection of the transactions. It detects the current transactions for the account used in the bot and compare them to find the vote transactions.
steem.api.streamOperations
: This function listens to the live stream of Steem blockchain operations. It provides real-time updates on various blockchain activities such as votes, comments, posts, etc. But in this case it only seeks for the vote transactions.If an error occurs while streaming then the error message is printed.
When it finds the vote transaction then It extracts the necessary information from the vote operation:
voter
: The username of the person casting the vote.author
: The author of the post being voted on.permlink
: The permlink of the post being voted on.weight
: The weight of the vote.
It checks if the
voter
is the same as the bot username in my case mohammadfaisal and if the vote weight is greater than 0.If both conditions are true then it prints a message which indicates that the vote was detected and proceeds to call the
createComment
function to post a comment on the voted post.
Here the live working of this bot can be seen when I am voting on the posts then the bot is detecting the vote transaction from my account and after that the bot is commenting on the voted posts.
Here you can see the app version in the JSON Metadata which is with my name mohammadfaisal/1.0.
Use SteemWorld, Ecosynthesizer, or any other explorer to show the transactions you have created, leave link just like me.
Both the tools steemworld.org and ecosynthesizer are very extensive and useful for the steem blockchain. But I think it is easy to share the transaction information by using ecosythesizer so I will share as the professor shared the transactions using ecosynthesizer.
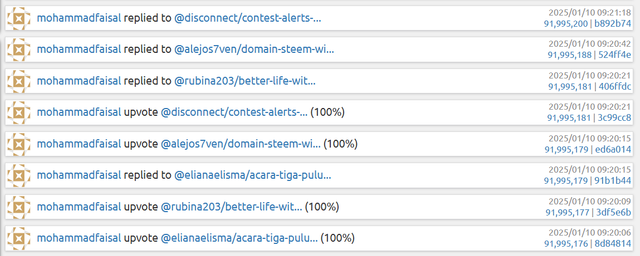
I did vote on four different people after running my bot which has detected these votes and then it broadcasted my comment under those posts on which I voted. In the screenshot there are comments followed by my vote. Here is the more detail about these transactions.
![]() | |
---|---|
![]() | |
![]() | |
![]() | |
![]() | |
![]() | |
![]() | |
![]() |
These are all the transactions which I will done with the help of my bot. All the transactions are shared from Ecosynthesizer.
Transfer 0.001 STEEM to @eight888 using Steem JS, add in the memo the title of this lesson.
In order to transfer the STEEM by using the Steem JS I will use its transfer specific operation and I will transfer the amount of STEEM to the given account.
This is the program which easily carry out the transaction of the transfer of the STEEM with the custom memo message. Here is the explanation of the code:
At the start of the program to use the transfer feature of the Steem JS I have imported the steem library in the constant variable. More information about the remaining constants is given below:
username
: This will hold the username of my account.active_key
: This will be used to hold the private active key. Because this key is used for the wallet transactions or for the transfer of the assets.recipient
: This constant will hold the username of the person to whom I will send STEEM. I have initialized this constant with the usernameeight888
as per the instructions in the question.amount
: This will be used to hold the amount of STEEM I will transfer. I have set this amount of transfer to0.001
STEEM.memo
: This is used to hold the memo or message with the transaction. I have added the memo as the title of this lesson.
This is the Transfer Function whcih performs the transfer of the STEEM to the account with the memo. It uses the constants which we have recently declared at the start of this program. More information of this function is given below:
steem.broadcast.transfer
is used to send STEEM to the recipient. While sending STEEM to the recipient it takes the following parameters:active_key
: Active key will be used as we have given and stored in the constantactive_key
. It will give access to the account for the transfer of the assets.username
: It accepts username which is mine as I have already declared in the constantusername
. If the username and the active key matches then the transaction will be carried out other it will display the error message.recipient
: This accepts the username of the receiver.amount
: With this constant it accepts the amount of STEEM to transfer including the symbol such as"0.001 STEEM"
.memo
: This gets the message stored in thememo
and attach it with the transfer transaction.
I have set some rules to handle the exceptions and errors while the execution of the program and transfer:
- If there is an error during the transfer it is logged to the console.
- If the transfer is successful then it logs the successful message to inform the user.
This code has transferred 0.001
STEEM to the user @eight888
and included a memo with the lesson title as it can be seen in the above picture.
This is the information of this transfer from the Steemworld.org where the sender, receiver, memo and the amount of the steem is visible along with the block number and transaction id.
Here is the information of this transfer transaction from the Ecosynthesizer.
Great Job!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thank you so much @alexmove.witness for praising my efforts. It motivated me to work more great.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
This is great, I'm glad. It's great when we motivate each other and inspire each other to work and develop. Steemit is a great support in this.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Indeed we can grow only with mutual support.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
@alejos7ven - when will this post be graded?
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hi,
I am going to do it in a few minutes, I was in a trip, and all entries came in the weekend when I was out already.
Don’t worry, winners will be published without delays and entries will be properly reviewed.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit