.gif)
Source: http://gph.is/1GhDD0S
Disclaimer: This article is written by a beginner combing through new concepts.
React/Redux is the hottest frontend frameworks these day. If you google the term Redux diagram, and this is what you get:

Source: Google Search
As someone who just finished reading up on React and wanted to learn more about Redux, this was how I felt when I saw the search results.
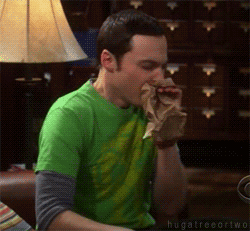.gif)
Source: Google Search
I think one of the obstacles with understanding Redux is the terms like reducers, actions, and thunks. I highly recommend anyone starting out with Redux to read the core concepts outlined in the official documentation:
Long story short, Redux application has a plain object, known as store, which serves as data model for your app. This object can be composed by a series of smaller object, known as state. For example, for a list of articles, I can have an article state that looks like this:
state = {
articles: [{
"id": 314,
"title": "6 innovative apps utilizing the ethereum network",
"source": "Investopedia",
"link": "http://www.investopedia.com/news/6-innovative...",
"date": "1500523200",
"type": "msm"
},
{
"id": 893,
"title": "what is plasma and how will it strengthen...",
"source": "Investopedia",
"link": "http://www.investopedia.com/news/what-plasma-and...",
"date": "1502856000",
"type": "msm"
},..]
}
The store is going to be the same since there’s only one state at this point. If we add more states to our application, the store object will have more key value pairs.
We have our store object. Unlike objects you encounter in Object Oriented Programming, the store has no setter. This is by design so that different parts of code can’t not change the store arbitrarily. The store is the single source of truth in our application so we guard it carefully.
To change the state and store, we dispatch actions. Actions are really just plain objects. In our article example, fetching articles is an action that looks that this:
{ type: 'ARTICLES_FETCHED',
payload: [{
"id": 314,
"title": "6 innovative apps utilizing the ethereum network",
"source": "Investopedia",
"link": "http://www.investopedia.com/news/6-innovative...",
"date": "1500523200",
"type": "msm"
},
{
"id": 893,
"title": "what is plasma and how will it strengthen...",
"source": "Investopedia",
"link": "http://www.investopedia.com/news/what-plasma-and...",
"date": "1502856000",
"type": "msm"
},..]
}
Action usually has these two properties: type and payload. A typical pattern in Redux application is dispatching an action with fetched data after an asynchronous call to the server.
And last but not least, reducers tie states and actions to together. It’s just a pure function with a switch statement that checks the action type and return new state of the app. In our article example, the reducer looks like this:
const initialState = {
articlesById: null,
}
export default function(state = initialState, action) {
switch (action.type) {
case types.ARTICLES_FETCHED:
return {
...state,
articlesById: action.articlesById
}
default:
return initialState
}
}
To summarize the basic ideas of Redux:
- it describes state as plain objects, and store (global state) by merging all the states in your app.
- it describes changes to the app as plain objects.
- it uses pure functions to handle changes.
The redux API then bridges your redux logics with react views while providing some utilities to compose states.
Say we send a request to a remote server and fetch some articles. We dispatch ARTICLE_FETCHED action. The reducer evaluates the action and store the payload articles in the state. What now?
After the state changes, we need to render the view. We need to somehow connect the store to the component. This is done with a function provided by react-redux library called connect.
import React, { Component } from 'react';
import autoBind from 'react-autobind';
import { connect } from 'react-redux';
class ArticlesIndex extends Component {
// implementation
}
function mapStateToProps(state) {
return {
articles: state.articles
}
}
export default connect(mapStateToProps)(ArticlesIndex)
Consider the code above, the connect function takes in a function called mapStateToProps that returns an object with keys. The keys is going to be mapped to the props of the component. Now let’s bring back some diagram.
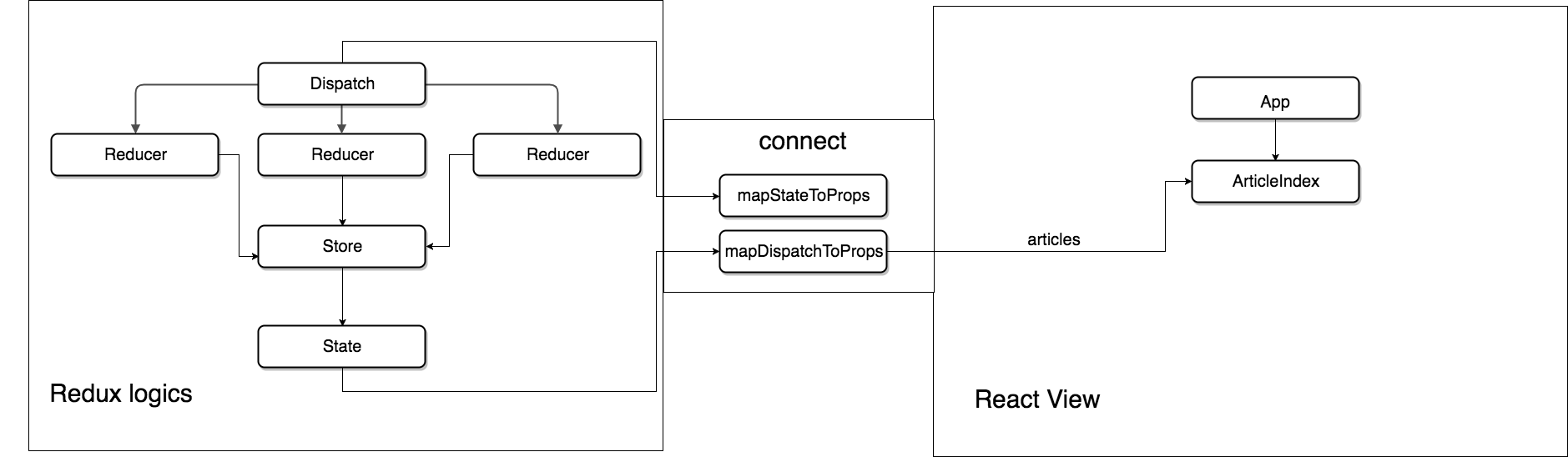
The connect function takes in two functions called mapStateToProps and mapDispatchToProps. mapStateToProps and mapDispatchToProps in the connect closure are granted access to Redux state and dispatch. The connect function acts as an API between Redux and React. The data flow under React/Redux architecture looks like this:

I just introduced the essential concepts that helped me building my first React/Redux app. There are much more to the Redux architecture, like middleware, thunks and selectors. I encourage you to read about them in the Redux documentation.
If you want a walk through these concepts with real life implementation, check out the step-by-step tutorial:
[Web dev] Beginner’s guide to react/redux — how to start learning and not be overwhelmedThanks for reading!
TC
Hello! Im a begginer too on React/Redux, i liked your article, very straightforward. I have a question, if i have lets say two components on lets say my index.js, do i need separate reducers and actios to comunicate with the same store? or can i communicate two actions to just one reducer?
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hi! I am a robot. I just upvoted you! I found similar content that readers might be interested in:
https://medium.com/@tctammychu/beginners-guide-to-react-redux-painting-a-mental-model-ed0279d55836
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit