Want to learn a new programming language? In this series, we'll be learning the basics of Perl, a gem of a programming language written by linguist Larry Wall. It will work on virtually any operating system and platform and, best of all, it's completely free. This series of masterclasses is aimed at everyone, from beginners to programming, to those with some programming experience. So, whether you prefer a GUI or command line, Windows or Linux, brace yourself for the impact as we learn the basics of programming and a computer language, starting off with variables.
Making sense of values
Data is presented to a program in a number of ways, either as a 'literal' or as a 'variable' of some sort. Literals are where the actual value is written in the program as '3.14' or 'ostrich' for example, but programs would be very restricted in what they could do if they could only process literals. This is where variables come in. Essentially, there are two types of variable: those that can hold one value, and those that can hold several. If a piece of program code can be written so that it refers to a value by a name, that piece of code can then be used with any value. In effect, variables are like pigeon holes – they can hold anything you want to put in them and all you need to know is the name you have given them. If you want to add 3 to 5 using literals, you will get 8 and a piece of code that is rather limited. If you want to add a to b you can use that code to perform any addition.
First of all, we will look at literals, both numeric and string. These are the way that data is hard-coded into a program. In effect, all data that is not gleaned from an external source, such as a file or user input, has to come directly or indirectly from a literal of some sort. A literal can be a number or a string (a series of characters) which can then be processed or used directly by the program.
Numeric literals
Examples of numbers include -25, 3.14159265358, 6.02E23 and 441332681422. The first is an integer (whole number); the second a floating point (figures after the decimal point); the third an exponential (a number that is so big or small that it's easier to write it using this shorthand – in effect, this number is 6.02 multiplied by 10 to the power of 23); and the last is just a big number. In the case of the last number, Perl makes it easier to read by using underscores in the same way we would use commas, thus it becomes 441_332_681_422.
One thing that you might not have noticed about these numbers is that they are all in base 10. As with other programming languages, Perl will also enable you to use base 2 (binary), 8 (octal) and 16 (hexadecimal). While using bases other than 10 might seem a waste of time, there are times when presenting data in these forms, either to the program or the user, is very useful.
To use binary, start the number with '0b' (number zero not letter O). For example, 0b10010101 and 0b1100101101110111. Numbers don't have to be multiples of 8 bits as long as they will be packed with leading zeros when Perl uses them. To use octal, start off with zero, for example, 05372 and 02745.
With binary, octal and decimal, we have used the numbers 0 to 9, but with base 16 we have run out of numbers. To get around this we use letters, starting with 'a' for 10. So, the series of characters that is allowed in hexadecimal is 0123456789abcdef. To present a literal for hexadecimal use '0x', for instance, 0x4a8bde. Just in case you were wondering, you can again use underscores to make long numbers easier to read. For example: 0b1001_ 0101; 053_72; and 0x4a_8b_de in binary, octal and hexadecimal notation respectively.
Coping with strings
String literals in Perl start off either as single quoted (') or double quoted (") strings. The characters they contain are all of the printable characters with ASCII values between 32 and 126 inclusive. (ASCII is a standard that designates certain values to certain characters so that a space has a value of 32 and an upper case A has a value of 65 – another standard is EBCDIC). A simple example of a string literal is 'hello world'.
One problem that you might have spotted is that if you want to use a single quote, you will end up defining the end of the string prematurely. For example, 'don't think that I didn't warn you' will be two strings ('don' and 't warn you'), with a curious bit of code between them ('t think that I didn'). To get around this you use a special character which forms an 'escape sequence'; in the case of Perl this is the backslash (). See the box on escapes on page 154. Therefore, to get a single quote in a single-quoted string, use '. Now our string looks like: 'don't think that I didn't warn you'.
Another way of getting around this problem is to use double quotes. Now, "don't think that I didn't warn you" works perfectly well without escaping any characters although, if you wanted a double-quote character, you would need to escape that instead. So, since nothing was gained in the long term, why does Perl support single- and double-quoted strings? Why not just have one? Well, each has advantages. Single-quoted strings enable you to use any character except a single quote and a backslash. Double-quoted strings enable you to use escape characters and also other variables within literals. In case you were wondering what sort of mess a string full of double and single quotes would look like if escaped, there is another mechanism to your rescue: you can use q// and qq// for single and double quotes respectively. In addition to this, you needn't use a slash, so if you have a string with lots of slashes and quotes in it (HTML code for example), you can use a '-' if you prefer.
Scalars explained
Scalars hold a single value and, unlike a lot of other languages where you have to pick between single- or double-precision, integer, long, currency, string and so on, in Perl there is just one type. Examples of scalar names are '$val', '$c', '$chosen_metal_implement' – see the box below for sensible names for variables.
Values are assigned to scalars using '=' and because, in the case of strings, white space can be used within a variable, or generally to make the program more legible, each program statement ends with a semicolon thus:
$a = 236_927;
$b = "Hello world. How are we today?";
You can also include other scalars within double-quoted strings so the following would give the same output:
$c = "Hello world.\n";
$c = "$cHow are we today?";
Note that we used the '\n' new line character. Also, we took the old value of $c, added the new bit of text to it and stored that back in $c – this shows that we are performing a process and not merely writing an equation. This last line could have been written using the concatenation operator '.' (concatenation is sticking strings together) or using a binary assignment operator (binary as in taking two operands) '.=':
$c = $c . "How are we today?";
$c .= "How are we today?";
Other similar operators, but this time for numbers, include '+=', '-=', '=', '*=' and so on. In addition to these there are also 'here documents', which enable you to input several lines or pages worth of text in one go. The assignment starts with
<<
and then defines the text label that will terminate the text. Note that you must have a line after the label and that the label does not have a semicolon after it:
$d= <<EndOfText;
Hello world. EndOfText
Arrays and hashes
Arrays are a way of holding more than one value in a variable. Instead of having $a_0, $a_1 and so on up to $a_1000, you can have $a[0], $a[1] up to $a[1000]. You are not limited to one dimension, either: you can have many if you need to, for instance, '$threed[5][2][8]'. Although there doesn't seem to be much of an advantage in terms of typing, you can use a variable instead of a 0, 1 and so on; $a[$x] is easier to process and more flexible.
Collectively, the array starts with an '@' instead of a '$' so '@vars
' and '@bikes
' are arrays. They all start at 0 and you can find the index number of the last variable by using '$#', thus '$#vars' or '$#bikes'. Assignment can be either individually or by a list so:
@names = qw/ Bob Lisa Chris Mike /;
This will give '$names[0]' a value of 'Bob' and so on. Hashes are a way of storing a special, key variable with a value. All the keys have to be unique as these are useful for storing information about users on a system or passing environment variables to your program. We'll come back to hashes in another tutorial..
Perl is free and easy to use
So, what's the catch and where do I get it?
With the obvious exception of Windows, Perl comes with virtually every OS as part of the default installation. On Unixlike systems, you will usually find it in /usr/bin or /usr/local/bin and you can execute a file just by typing './yourprog' from the directory where the script is – there is no need for any extension.
For Windows, you can download the ActivePerl distribution free from here https://www.activestate.com/activeperl.
It will run programs that are written on Unix-like OSes without any problems – the main difference is that on Windows, lines end with CRLF instead of just LF. Also, in Windows, your Perl scripts need the file extension .pl or another one associated with ActivePerl. This is because Windows doesn't recognise a file by its contents so doesn't know what to do with it without an extension. Having said that, ActivePerl does run your Unix scripts if you just add '.pl' to the end of the filename.
The programming in this series focuses on the basic Perl installation. If you want to try some of the modules, such as the Tk environment, and you have OpenBSD, you'll need to install the extra modules from the OpenBSD site/ mirror using pkg_add. The Perl modules (.tar.gz) are all under 'P5' so, for Tk, you need to install the p5-Tk-*.tar.gz module as root. Once it is installed, you can run as a normal user.
So, what's the catch? Well, there isn't one. The Perl you get is not time-limited, isn't betaware and doesn't have restrictions on how many times you can print something out or how big an array you can create. Plus, it doesn't tag all your output with an image or a line saying 'made with...'. It's the same full, unrestricted, cross-platform environment that runs on systems across the world.
Posted on Utopian.io - Rewarding Open Source Contributors
Your contribution cannot be approved yet because it is not as informative as other contributions. See the Utopian Rules. Please edit your contribution and add try to improve the length and detail of your contribution (or add more images/mockups/screenshots), to reapply for approval.
You may edit your post here, as shown below:
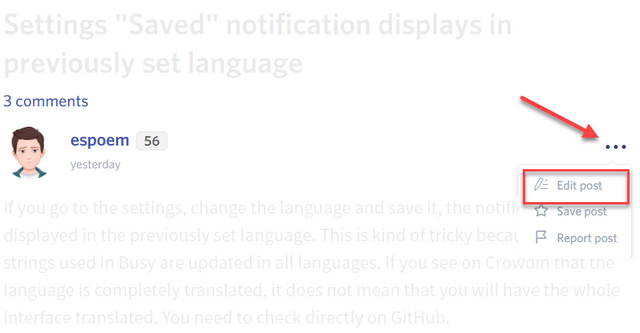
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Done.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey @aminda I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit