Hello, everyone!
I'd like to share about what is and how to code object oriented programming in Java language with IntelliJ.
What is Java?
Java is a computer programming language and computing platform.
What is IntelliJ?
IntelliJ is an IDE created that make possible for us to code or develop something useful for people.
What is IDE?
IDE is an Integrated Development Environment that means provide the basic tool for developers to code and test the software. Basicly, it will contain code editor, compiler and debugger.
What is OOP?
OOP stands for Object Oriented Programming. OOP is a programming concept which applies object concept that the object is consisted of attribute and method.
Now, let's start.
Step 1
Install and run IntelliJ which can be downloaded at here or directly from the community at here. Yes, it's officially built by community. That's why it's called as community edition from the official jetbrains web and it's free.
You can choose what's disabled plugin or not.
Step 2
Create new project to begin.
Name it as you want to for the project name and package. I recommend the package to be written with lowercase, all of it. Company used to name the package just like their domains, like com.google.android
Step 3
Don't forget to setup your SDK first.
Step 4
Create new Java class. This is for the attribute and method inside since the tester class is created by default at Main.java
Name it as you want to, but fit your project.
Step 5
You begin your coding. I'll give an example of the simple class which contains constructor, attribute and method.
This coding includes all of attributes. Attributes mean like public variable. So I define Quiz as variable with Double datatype. Datatype represent variant values in the variable.
double Quiz,MidExam,LastExam,Score;
char Index;
String Desc;
This one is a constructor. Constructor is created to initialize value to the object through parameter. Just like you see below. It initializes Quiz, MidExam and LastExam. It depends on what's method you want to create.
Score(){
Quiz=0;
MidExam=0;
LastExam=0;
}
This one is method. It includes procedure and function. This is what called as procedure. Procedure is a sub program to run several process, but not return the value. Like you see below, I've created void SetQuiz
to be able to set the value of the Quiz.
void setQuiz(double x){
Quiz=x;
}
void setMidExam(double x){
MidExam=x;
}
void setLastExam(double x){
LastExam=x;
}
This one is a function. Function is statement that create to run and return the value. Just like you can see below. I've created double getScore()
to get the score from the value you set for Quiz, MidExam and LastExam before or return the value.
double getScore(){
Score=0.2*Quiz+0.3*MidExam+0.5*LastExam;
return Score;
}
char getIndex(){
if(Score>=80 && Score<=100)
Index='A';
else if(Score>=68 && Score<=80)
Index='B';
else if(Score>=56 && Score<=68)
Index='C';
else if(Score>=45 && Score<=56)
Index='D';
else
Index='E';
return Index;
}
String getDesc(){
if(Index=='A')
Desc="Very Good";
else if(Index=='B')
Desc="Good";
else if(Index=='C')
Desc="Not Bad";
else if(Index=='D')
Desc="Bad";
else
Desc="Very Bad";
return Desc;
}
Now, get back to the tester class. The example of codes are like these.
You need to create new Object of your class you created before in this class. That's why you need to create constructor. It will refer as an instance of every object created. Just like you can below. n
can be anything. You can change to whatever you want to.
Score n=new Score();
The remain depends on you. Enjoy your coding!
This is The Final Result.
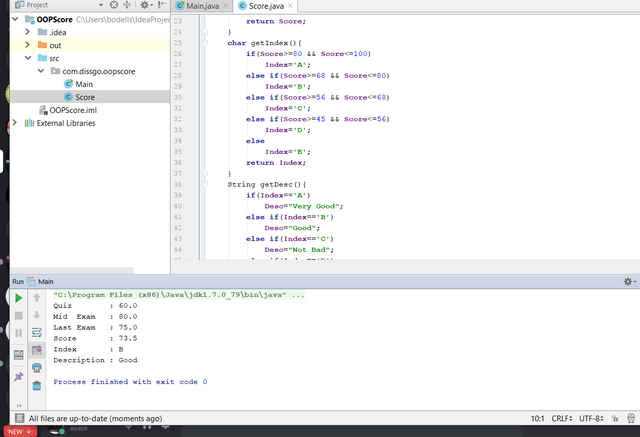
Posted on Utopian.io - Rewarding Open Source Contributors
Hmm... not bad, could use a lot more explanation instead of just the code dumping, though.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thanks for approval. Glad to contribute.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey @dissgo I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit