What Will I Learn?
- You will learn about 2D object
- You will learn about C++ programming
- You will learn about how to create 2D object
Requirements
- You need a knowledge about 2D object
- You need a knowledge about C++ programming
- You need to know how to build a 2D object
Difficulty
- Intermediate
Tools
Tutorial Contents
Today I will post a tutorial about how to create a 2D object . The main requirement is you have to have the Visual Studio Code or other open source applications. Before we step into the tutorial, I'll explain the 2D concept first.
2D objects are formed by a set of lines, where every single line is formed from the connection of two points. One point consists of horizontal coordinates and vertical coordinates, or can be written in (x,y) format. And the function of the (x,y) coordinates is to determine the position where the point is located. Where x is the horizontal coordinate, whereas y is the horizontal coordinate.
And for this time, I will show how to create a 2D triangle object. Before that, you must know the coordinates to build the shape of the triangle. For example, we will try to build a triangle with the coordinates as shown below.
Explanations:
- Point A has coordinates (100,100)
- Point B has coordinates (300,400)
- Point C has coordinates (500,100)
Then, we can directly practice it by using C ++ programming. And you can follow the syntax below, the syntax is also equipped with an explanation, so it will facilitate you in learning and doing analysis.
#include <iostream>
The above syntax serves to declare headers to be used in C ++ programming. Commonly used to perform input and output on the program
#include <stdlib.h>
The above command is used for mathematical operations. So it is perfect for our tutorial this time.
#include <GL/glut.h>
The above command serves to call the function of openGL, or commonly used for graphical purposes. Either for 2D or 3D graphics, the openGL function should still be used.
typedef struct
{
float x,y;
}
point2D_t;
Here is the declaration of the variables x and y. Which will be used as coordinates (x, y).
void userdraw(void);
The function is to call userdraw function.
void drawDot(point2D_t p)
{
glBegin(GL_POINTS);
glVertex2f(p.x,p.y);
glEnd();
}
The above command serves to do the depiction of the dots.
void drawLine(float x1,float y1,float x2,float y2)
{
glBegin(GL_LINES);
glVertex2f(x1,y1);
glVertex2f(x2,y2);
glEnd();
}
The next command serves to perform lines drawing.
void drawLine(point2D_t p1,point2D_t p2)
{
drawLine(p1.x,p1.y,p2.x,p2.y);
}
After that, the above syntax has a function to connect the dots. So in this process will produce a line.
void drawPolyline(point2D_t pnt[],int n)
{
int i;
glBegin(GL_LINE_STRIP);
for(i=0;i<n;i++)
{
glVertex2f(pnt[i].x,pnt[i].y);
}
glEnd();
}
The above command serves to draw an open curve or named as polyline.
void drawPolygon(point2D_t pnt[],int n)
{
int i;
glBegin(GL_LINE_LOOP);
for(i=0;i<n;i++)
{
glVertex2f(pnt[i].x,pnt[i].y);
}
glEnd();
}
While the above command is used to draw a closed curve or polygon.
void setColor(float red,float green,float blue)
{
glColor3f(red,green,blue);
}
Is the command to declare the red, green, blue (RGB) color.
void userdraw(void)
{
point2D_t segitiga[3]={{100.,100.},{300.,400},{500.,100.}};
setColor(0.,0.,1.);
drawPolygon(segitiga,3);
}
It is a command to draw a triangle shape as we wish.
Here we use a segitiga[3] array to hold each value of the triangular coordinates.
For the value, we just need to fill in the corresponding value as in the triangle image above.
Then the command setColor(0., 0., 1.) serves to give the blue color on the triangle line.
void display(void)
{
glClear(GL_COLOR_BUFFER_BIT);
userdraw();
glFlush();
}
This syntax used to call the userdraw() function to be displayed as output.
void main (int argc,char **argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);
glutInitWindowSize(640,480);
glutInitWindowPosition(100, 150);
glutCreateWindow("Segitiga 2 Dimensi");
glClearColor(1.0, 1.0, 1.0, 0.0);
gluOrtho2D(0., 640., 0.0, 480.0);
glutDisplayFunc(display);
glutMainLoop();
}
The last command is as main function. This is where we can set display mode, windows size, window position, window title, and display function call.
So the results will be displayed.
For the result will look like the following picture.
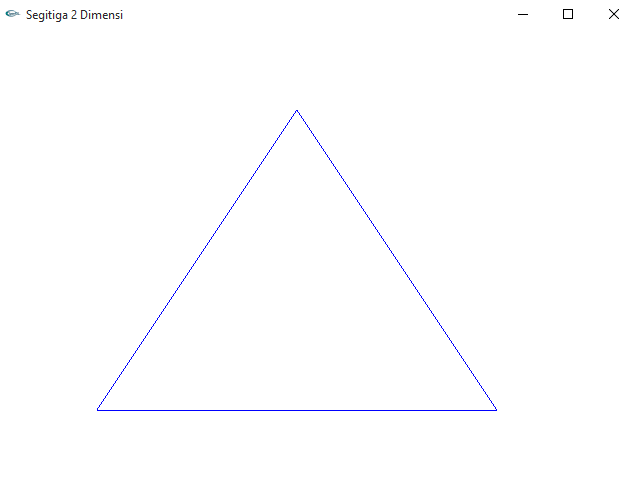
And for the full syntax, you can download it here
Hopefully this tutorial is useful for all of us.
Posted on Utopian.io - Rewarding Open Source Contributors
Would you tell me please
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Nice questions, I have some reasons for these:
In my last posting, I got a suggestion to use iostream than stdio.h. But I think that is no problem, you can use one of them.
Why drawing , because I just want to introduce that openGL is important. It is a new inovation from me. This tutorial is also rare, not general.
And I am a girl, lol :v
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Your contribution cannot be approved because it does not follow the Utopian Rules.
This contribution cannot be approved because:
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Can you show me the right repository?
@roj
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Actually, I explained that there is no proper repository for this post unless OpenGL implementations like MESA gets accepted. I am having conversations with my supervisor about this. If there is any news I will inform you, until that time this post is rejected.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Big thanks sir
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit