Repository With Sample Code
https://github.com/imwatsi/crypto-market-samples
Find the complete Python script on GitHub: ta_stoch.py
My Profile On GitHub
You can find code used in this tutorial as well as other tools and examples in the GitHub repositories under my profile:
What Will I Learn
- Import ETHUSD 1 hour candle data from HitBTC
- How to use the Stochastic Oscillator class from the open source technical analysis library from Bitfinex
- Filter the results based on basic overbought and oversold readings
Requirements
- Python 3.6+
- Dependencies
requests
modulebfxhfindicators
module
- Active internet connection
Difficulty
Basic
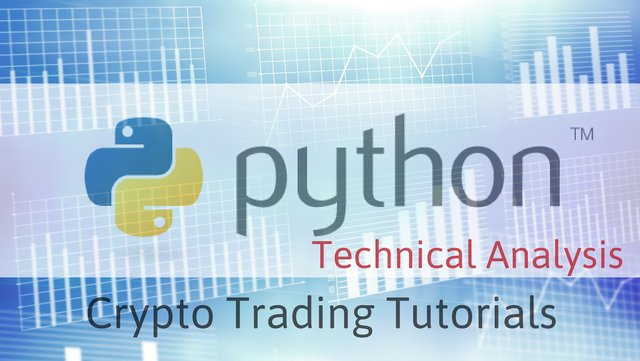
Tutorial Content
In this tutorial, we will use the Stochastic indicator from bfxhfindicators to calculate the Stochastic Oscillator values of the ETHUSD market, in the 1 hour timeframe.
Definitions of terms
Here are a few definitions of technical terms used in this tutorial:
- Stochastic Oscillator: a momentum indicator comparing a particular closing price of a security to a range of its prices over a certain period of time. It is used to generate overbought and oversold trading signals, within a 0-100 bounded range of values. It has two values: K and D. K is a fast moving value and D lags behind and is less sensitive to fluctuations.
- Candles: candle, also called candlestick, is a representation of a range of prices that an asset traded within over a certain period of time. They are used to generate candlestick charts. Each candle has high, low, open, and close price values. When obtained from the exchanges, volume info is also included
- Overbought: a state in which the price has been increasing and is considered due for a reversal
- Oversold: a state in which the price has been decreasing and is considered due for a reversal
About the Stochastic indicator
The Stochastic indicator from bfxhfindicators accepts candles as input. This means we need to feed it dictionary entries of candles, i.e. a stream of dictionaries containing the following:
high
: the highest price reached during the time periodlow
: the lowest price traded at during the time periodclose
: the price at which the time period ended
An overview
Below is a screenshot of the ETHUSD chart on TradingView, showing the 1 hour candles and the Stochastic Oscillator values beneath.
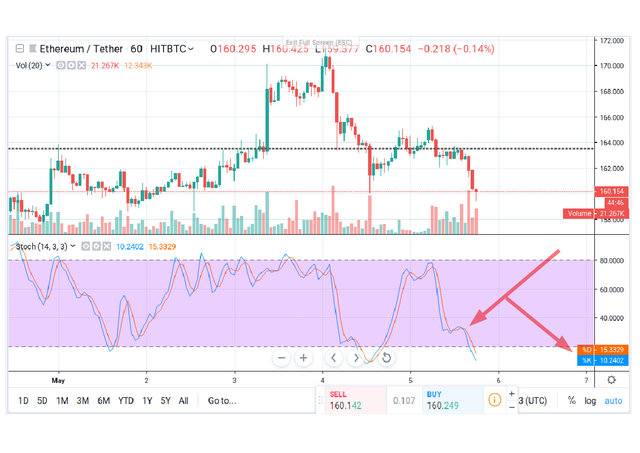
What we want to do is to have an object in our code that contains the current values for the %K and %D Stochastic Oscillator numbers. This can be used as part of a strategy to initiate buy or sell signals.
Let's write the code
Import the modules
We first import the modules needed:
import requests
import json
import time
from bfxhfindicators import Stochastic
from threading import Thread
requests
to connect to the HitBTC REST API and get candle datajson
to parse responses received via requeststime
for implementing time delaysbfxhfindicators
module hosts the 'Stochastic' class, which we will use for the technical analysisthreading
to use the Thread class to open new threads for functions
Define a function that continuously loads candle data
To have continuously updated candle data, a loop is created. It will make a request to the HitBTC API for the latest candle data, at a predefined rate (every 5 seconds in this tutorial, but can be anything you want). This will update the values for the latest candle (the hour we are in) and will also add new candles when we enter a new hour.
candles = []
def load_candles():
global candles
while True:
resp = requests.get('https://api.hitbtc.com/api/2/public/candles/ETHUSD?period=H1')
raw_candles = json.loads(resp.content)
parsed_candles = []
for raw_c in raw_candles:
new_candle = {
'timestamp': raw_c['timestamp'],
'close': float(raw_c['close']),
'low': float(raw_c['min']),
'high': float(raw_c['max'])
}
parsed_candles.append(new_candle)
candles = parsed_candles[:]
time.sleep(5)
We also defined a variable candles = []
which will store the candles. We define it here because it will be referenced by some code that starts before the requests come in from the server. Without this explicit definition, an error will occur for trying to reference a variable before it is defined.
By issuing a GET request to the url https://api.hitbtc.com/api/2/public/candles/ETHUSD?period=H1
, we state the symbol 'ETHUSD' and time period for candle 'H1' for 1 hour.
HitBTC sends back a JSON object containing a list of dictionaries, with each dictionary being a candle. Here's the format:
[
{
"timestamp": "2017-10-20T20:00:00.000Z",
"open": "0.050459",
"close": "0.050087",
"min": "0.050000",
"max": "0.050511",
"volume": "1326.628",
"volumeQuote": "66.555987736"
},
...
As it is, we cannot pass this dictionary format to the Stochastic indicator because it requires the 'high' and 'low' keys, which in this case are named 'max' and 'min' respectively. That's where the code under this 'for' statement comes into play: for raw_c in raw_candles:
. This is effectively a loop that goes through the entire list of candles and recreates it, changing the 'max' and 'min' keys to 'high' and 'low', saving the resulting list in the variable parsed_candles
.
This final line code copies the new list to our global variable candles
which we will use for our technical analysis: candles = parsed_candles[:]
.
The main code
From this point, we write the code that will start executing as soon as the script is run. First, we start a new thread for the load_candles()
loop.
# start loop that loads candles
Thread(target=load_candles).start()
Next, we put a loop with a time delay conditioned on the length of the candles
variable. It makes the script wait until the candles are loaded (until the length of the candles list is bigger than zero).
# wait for candles to populate
while len(candles) == 0:
time.sleep(1)
Finally, we write code to do the calculations for the Stochastic Oscillator:
# calculate Stochastic Oscillator values
while True:
iStoch = Stochastic([14,3,3])
for candle in candles:
iStoch.add(candle)
stoch_values = iStoch.v()
# print Stochastic values, identify basic levels
str_print = 'ETHUSD: K:%s D:%s' %(round(stoch_values['k'],4), round(stoch_values['d'],4))
if stoch_values['k'] > 80 and stoch_values['d'] > 80:
str_print += ' In overbought area...'
elif stoch_values['k'] < 20 and stoch_values['d'] < 20:
str_print += ' In oversold area...'
print(str_print, end='\r', flush=True)
time.sleep(1)
The Stochastic Oscillator will have the following settings:
- K = 14
- D = 3
- Smooth = 3
This line is used to create an instance of the indicator with those settings:
iStoch = Stochastic([14,3,3])
The current Stochastic values are stored in the
stoch_values
variable by this linestoch_values = iStoch.v()
.The section commented "print Stochastic values..." prints the values on the terminal, in realtime.
Basic filtering based on Stochastic values
In the code above, a simple way to filter the results of the Stochastic Oscillator is shown. Values are checked for being either above 80 (for overbought readings) or below 20 (for oversold readings). Much more can be done with these values, including identifying divergences with price action, or identifying when the faster moving %K crosses the smaller moving %D in critical areas.
I plan to write a follow-up tutorial with these more advanced filters in the near future. This brings us to the end of this tutorial.
Find the complete Python script on GitHub: ta_stoch.py
Other Related Tutorials
Use Python to Retrieve All USDT Trading Pairs on Binance and Filter by {Price Vs EMA}
Thank you for your contribution @imwatsi
After reviewing your contribution, we suggest you following points:
The structure of your tutorial has improved.
In your tutorial put GIFs with the results of the terminal.
Thank you for following some suggestions we put on your previous tutorial.
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Chat with us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thanks for the review.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thank you for your review, @portugalcoin! Keep up the good work!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hi @imwatsi!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey, @imwatsi!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Very useful guide Thank you for providing this https://9blz.com/smi-indicator-explained/
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit