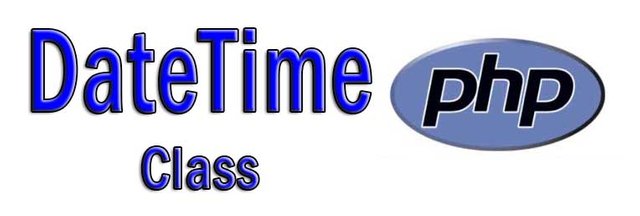
Introduction
The DateTime class provides a series of functions that allow not only to obtain the date and time of the server where the php works, but also allow to compare values of dates, add or subtract days, months or years.
It also allows you to save negative and positive dates with a range of almost 300 billion years backwards and forwards in the calendar.
Class DateTime
Instalation
The DateTime class does not need any type of installation, it is part of the php core
Requirements
Php 5.2 or higher is required.
Using DateTime
Instantiating the class
To create a DateTime object, you only need to instantiate the class, as we see in the following code:
$mydate = new DateTime();
You can also define the starting date of the object and the time zone that you want to assign to the object:
$lapazdate = new DateTime('2018-01-15', new DateTimeZone('America/La_Paz'));
You can find a complete list of time zones that php uses here
Formating the output of the DateTime class
The DateTime class has a method that allows you to display the date and time in the way you want. To do this, we use the format method, as shown in the following example:
<?php
$today = new DateTime();
$lapazdate = new DateTime('', new DateTimeZone('America/La_Paz'));
echo "today variable: ".$today->format('D, m-d-Y H:i:s')."<br><br>";
echo "lapazdate variable: ".$lapazdate->format('D, m-d-Y H:i:s');
?>
The output would be the following:
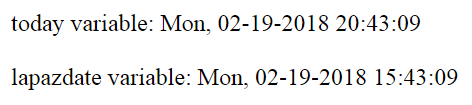
As we can see, the format method uses a series of constants to represent the month, day, year and other values:
The most used for date formats are:
Constant | Description |
---|---|
D | Name of day (Only three letters) |
l | Name of day (full name) |
d | Day of the month, 2 digits with leading zeros (01-31) |
m | Numeric representation of a month, 2 digits with leading zeros (01-12) |
Y | A full numeric year, 4 digits |
Specials:
Constant | Description |
---|---|
M | Name of month (Only three letters) |
F | Name of month (full name) |
j | Day of the month without leading zeros (1-31) |
w | Numeric representation of the day of the week 0 (for Sunday) to 6 (for Saturday) |
z | The day of the year (0 to 365) |
n | Numeric representation of a month, without leading zeros (1-12) |
y | A two digit representation of a year |
S | English ordinal suffix for the day of the month, 2 characters (st, nd, rd or th. Works with j) |
The most used for hour formats are:
Constant | Description |
---|---|
h | 12-hour format with leading zeros (01-12) |
i | Minutes with leading zeros (00-59) |
s | Seconds, with leading zeros (00-59) |
a | Lowercase Ante meridiem and Post meridiem (am/pm) |
A | Uppercase Ante meridiem and Post meridiem (AM/PM) |
Specials:
Constant | Description |
---|---|
H | 24-hour format with leading zeros (00-23) |
g | 12-hour format of an hour, no leading zeros (1-12) |
G | 24-hour format of an hour, no leading zeros (0-23) |
u | Microseconds |
Example:
<?php
$today = new DateTime();
echo "today variable: ".$today->format('l jS \of F Y h:i:s A');
?>
The output would be the following:

Getting the last day of the month
We can use the constant t together with the format method, to get the last day of the month.
Example:
<?php
$today = new DateTime();
echo "today is: ".$today->format('m-d-Y')."<br><br>";
echo "Last day of month: ".$today->format('t')."<br><br>";
?>
The output would be the following:
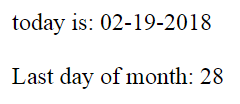
Changing the values
Adding days, months or years
You can use the add method of the DateTime class to add days, months or years.
Example:
<?php
$today = new DateTime();
echo "today is: ".$today->format('F jS, Y')."<br><br>";
$today->add(new \DateInterval('P1D'));
echo "tomorrow will be: ".$today->format('F jS, Y')."<br><br>";
$today->add(new \DateInterval('P1M'));
echo "Next month will be: ".$today->format('F jS, Y')."<br><br>";
$today->add(new \DateInterval('P1Y'));
echo "And next year will be: ".$today->format('F jS, Y')."<br><br>";
?>
The output would be the following:
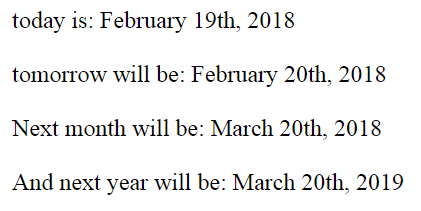
You can use the modify method of the DateTime class to increase days, months or years.
Example:
<?php
$today = new DateTime();
echo "today is: ".$today->format('F jS, Y')."<br><br>";
$today->modify('+1 day');
echo "tomorrow will be: ".$today->format('F jS, Y')."<br><br>";
$today->modify('+1 month');
echo "Next month will be: ".$today->format('F jS, Y')."<br><br>";
$today->modify('+1 year');
echo "And next year will be: ".$today->format('F jS, Y')."<br><br>";
?>
The output would be the same as the previous image:
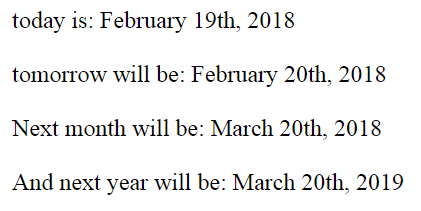
Subtracting days, months or years
You can use the sub method of the DateTime class to subtract days, months or years.
Example:
<?php
$today = new DateTime();
echo "today is: ".$today->format('F jS, Y')."<br><br>";
$today->sub(new \DateInterval('P1D'));
echo "Yesterday was: ".$today->format('F jS, Y')."<br><br>";
$today->sub(new \DateInterval('P1M'));
echo "Last month was: ".$today->format('F jS, Y')."<br><br>";
$today->sub(new \DateInterval('P1Y'));
echo "And last year was: ".$today->format('F jS, Y')."<br><br>";
?>
The output would be the following:
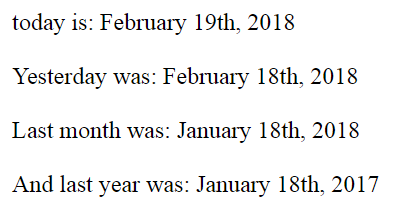
You can use the modify method of the DateTime class to decrease days, months or years.
Example:
<?php
$today = new DateTime();
echo "today is: ".$today->format('F jS, Y')."<br><br>";
$today->modify('-1 day');
echo "Yesterday was: ".$today->format('F jS, Y')."<br><br>";
$today->modify('-1 month');
echo "Last month was: ".$today->format('F jS, Y')."<br><br>";
$today->modify('-1 year');
echo "And last year was: ".$today->format('F jS, Y')."<br><br>";
?>
The output would be the same as the previous image:
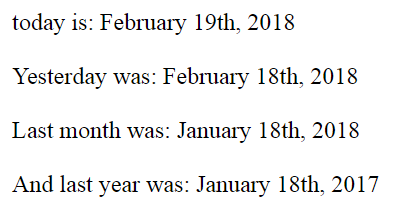
Get last day of the month (2nd way)
We can use the modify method, to obtain the date of the last day of the month.
<?php
$today = new DateTime();
echo "today is: ".$today->format('l, F jS, Y')."<br><br>";
$today->modify('last day of this month');
echo "The last day of the month will be: On ".$today->format('l, F jS, Y ')."<br><br>";
?>
The output would be the following:

Clone DateTime object
To copy a DateTime object, it is done using the clone command, as shown in the following example:
<?php
$today = new DateTime();
$tomorrow = clone $today;
$tomorrow->modify('+1 day');
echo "today is: ".$today->format('l, F jS, Y')."<br><br>";
echo "tomorrow will be:".$tomorrow->format('l, F jS, Y ')."<br><br>";
?>
The output would be the following:
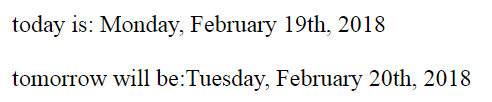
Difference between two dates
We can obtain the difference in days, months, years between two dates through the diff method, as shown in the following example:
<?php
$d1=new DateTime("2015-01-01");
$d2=new DateTime("2017-03-01");
$diff=$d2->diff($d1);
echo "d1:".$d1->format('Y-m-d')."\n";
echo "d2:".$d2->format('Y-m-d')."\n";
echo "Number of days between d1 and d2:".$diff->days."\n";
echo "Number of years:".$diff->y."\n";
echo "Number of month:".$diff->m."\n";
echo "Number of days:".$diff->d."\n\n";
print_r( $diff );
?>
The output, seen from the code of the page (Ctrl + U), would be equal to the following image:
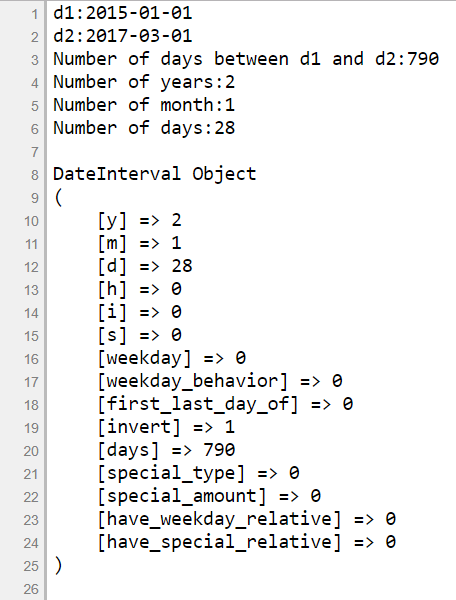
Posted on Utopian.io - Rewarding Open Source Contributors
Your contribution cannot be approved because it does not follow the Utopian Rules.
According to the blog post rules:
Your post content is very technical and is more in line with the tutorial category. It does not fit to blog post category.
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit