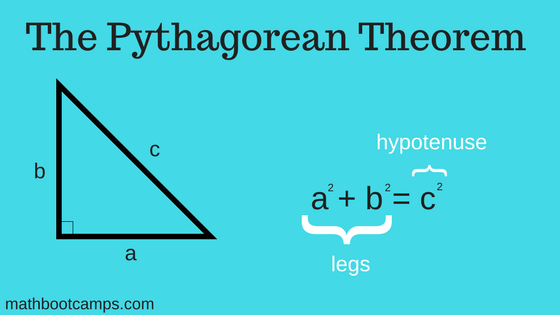
image source
the square of the hypotenuse is equal to
the sum of the squares of the other two sides.
What Will I Learn?
This tutorial aims to:
- Solve one side of a right-angled triangle using Pythagorean Theorem in Python
Requirements
- Python IDLE
Difficulty
- Basic
Tutorial Contents
The Code:
First, we import sqrt function from math module into the current namespace.
from math import sqrt
Display an information about the program (may not be included).
print('This is a Pythagorean Theorem calculator! Calculate one side of right-angled triangle.')
print('Assume the sides are a, b, c and c is the hypotenuse.')
Second, ask the user on which side to calculate and assign it to a variable (i used variable 'choice').
choice = input('Which side (a, b, c) do you want to calculate? side: ')
Third, making if ... elseif condition statement.
If the user wants to find for the hypotenuse which is side c.
if choice == 'c':
a = float(input('Input the length of side a: '))
b = float(input('Input the length of side b: '))
Solving for the c with the formula: √a^2+b^2
c = sqrt(a * a + b * b)
Display the value of side c
print('The length of side c is %g: ' %(c))
Else if, the user wants to find for the side b.
elif choice == 'b':
a = float(input('Input the length of side a: '))
c = float(input('Input the length of side c: '))
Solving for the b with the formula: √c^2-a^2
b = sqrt(c * c - a * a)
Display the value of side b
print('The length of side b is %g: ' %(b))
Else if, the user wants to find for the side a.
elif choice == 'a':
b = float(input('Input the length of side b: '))
c = float(input('Input the length of side c: '))
Solving for the a with the formula: √c^2-b^2
a = sqrt((c * c) - (b * b))
Display the value of side a
print('The length of side a is %g: ' %(a))
Lastly, if the input is invalid.
else:
print('Invalid Input!')
Curriculum
Please visit my previous tutorials:
Thanks for reading! Hope you learn something.
Posted on Utopian.io - Rewarding Open Source Contributors
Your contribution cannot be approved because it is not as informative as other contributions. See the Utopian Rules. Contributions need to be informative and descriptive in order to help readers and developers understand them.
Explanation:
Good luck on your next Python3 tutorial!
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
thank you sir
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey @scipio, I just gave you a tip for your hard work on moderation. Upvote this comment to support the utopian moderators and increase your future rewards!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit