What Will I Learn?
- Control Structures
- If-Else Structure
Difficulty
- Basic
Tutorial Contents
When writing the code, we may ask for an event to occur depending on some conditions. Let's consider a simple note system. Do not pass the student if he gets a grade below 50. We need to use the controls to achieve this. There are three types of control structures in Java. These; if-else, if else if, and switch structures. We use whichever structure we want to do.
If-Else Structure
Let's consider a student note system. If the average of the student is above 85 it's AA, if it's between the 70-85, its BA. We have to use if-else structure for this. In the -if-else structure, if the condition is true, it goes in to the "if" block and (a) block will be processed. if the condition is false, it goes into the "else" block and (b) block will be processed. If we do not want to happen any process at the else part, we can only use "if" part without writing else.
General If-else structure:
if (condition)
{
// transactions (a)
}
else
{
// transactions (b)
}
Flow Chart of the If-else structure:
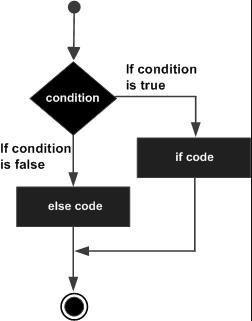
Let's see this with an example:
package com.org controlstructures;
public class Ifelsestructure {
public static void main (String[] args)
{
int grade=88;
if (grade>50)
System.out.println(" Success!" ) ;
else
System.out.println(" Fail" ) ;
}
}
On the screen we'll see;
Success!
If the process in the if or else structure is just one phrase, there is no need for { } symbols. If we want to write to the screen any other thing without "Success!", we will use { } that time.
if (grade>50)
{
System.out.println (" Success!") ;
System.out.println (" You've passed." ) ;
}
On the screen we'll see;
Success!
You've passed.
At the first example there was only one phrase. So we did not use { }. (Phrase means everything we put on ";" to the end)
Instead of giving ourselves the values we use in if we can get from the user. We can do it with "Scanner" classes. I'll teach them at the "Scanner Classes" tutorial.
package com.org.controlstructures;
import java.util.Scanner;
public class IfelseStructure {
public static void main(String[] args)
{
Scanner s=new Scanner (System.in) ;
System.out.println(" Enter the first number" ) ;
int number1=s.nextInt () ; // first number recieved
System.out.println(" Enter the second number" ) ;
int number2=s.nextInt () ; // second number recieved
if (number1>number2)
System.out.println (number1+" ," bigger than " +number2+" ) ;
else
System.out.println (number1+" ," smaller than " +number2+" ) ;
On the screen we'll see;
Enter the first number
4
Enter the second number
6
4, smaller than 6
At this example we took 2 different numbers from the user and compared those. According to the status of the numbers, the information message was automatically printed on the screen. However, in this example we assume that the numbers are not equal. In the case of equality, I will touch on the next tutorial (if- else if) to check what we need to do.
Curriculum
Before this tutorial i taught about different Java courses. If you want to check them;
- Partitioning the number using "_" - Boxing/Unboxing and Local Variables on Java
- Basic and Other Assignment Operators, Multiple Assignment Process and Variable Swap at Java
- What is Relational Operators on Java? -With examples
- Arithmetic operators and various operations we can do with them on Java
- Conditional and Bitwise operators on Java -With examples
- Shift and Type Comparison Operators / Operator Priority on Java
Posted on Utopian.io - Rewarding Open Source Contributors
Hi, your contribution cannot be approved because the tutorial does not meet the Utopian standards and requirements set by the rules.
You should focus on more advanced topics and concepts.
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit