New Features - AES Algorithm
Hello everyone, I have added new features and also changed the name of my application from EasyEncode to EasyEncrypt, a friend actually suggested that to avoid misconception of the functions of the application, and to the new features.
EasyEncrypt formerly EasyEncode will now support AES (Advanced Encryption Standard) Algorithm.
AES is based on a design principle known as a substitution–permutation network, a combination of both substitution and permutation, and is fast in both software and hardware. Unlike its predecessor DES, AES does not use a Feistel network. AES is a variant of Rijndael which has a fixed block size of 128 bits, and a key size of 128, 192, or 256 bits. By contrast, the Rijndael specification per se is specified with block and key sizes that may be any multiple of 32 bits, with a minimum of 128 and a maximum of 256 bits. Source
With the addition of this algorithm, the application will now allow the user to choose between Algorithms to be used, this new feature will improve security of encrypted text
Screenshots of the new Feature
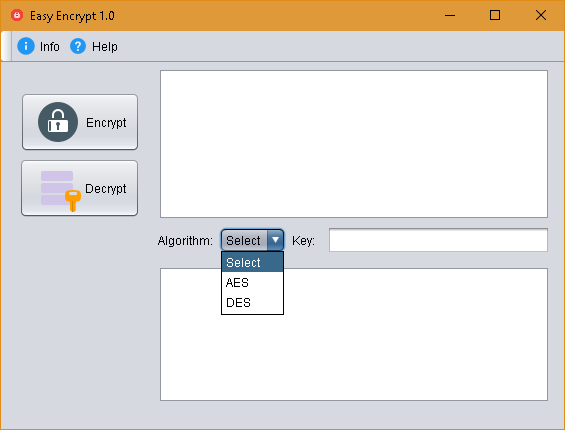
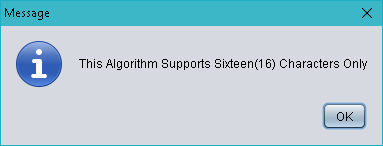
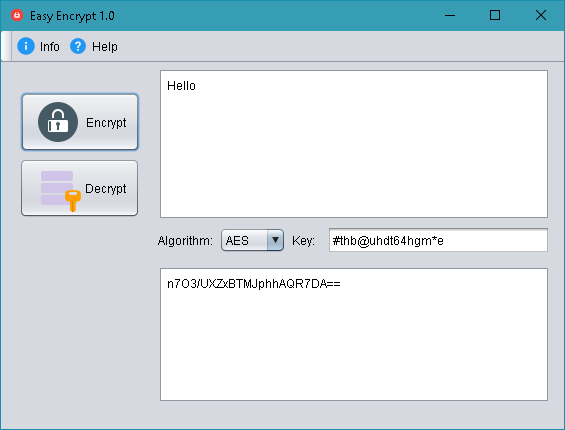
The same process happens for DES Algorithm, user is told the number of characters to be contained in the key, this feature makes it easier to be used by novices in the encryption world. I have added this thanks to suggestions from friends that have no idea what encryption is, so that it will suit the general public.
New Features - Application Guideline (Help)
Every application should have a means of teaching its users how to use it, for first timers especially and those who just want to know how to use the new features, I have made a simple description of how to use the application, linked to the help button on the menu bar of the application.
Screenshot of the Application Guideline
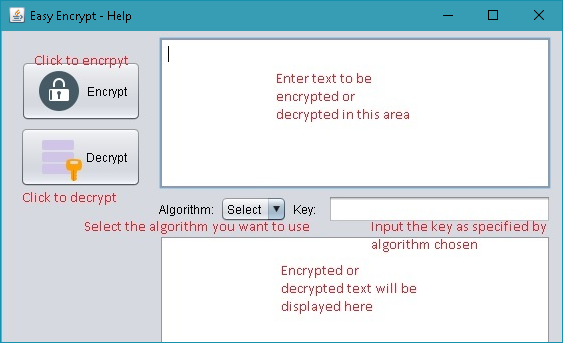
Implementation
AES Algorithm
Previously, I have added the algorithm myself upon actionperformed on the encrypt button here:
try {
byte[] k = key.getText().getBytes();
SecretKeySpec secretkey = new SecretKeySpec(k, "DES");
Cipher enc = Cipher.getInstance("DES");
enc.init(Cipher.ENCRYPT_MODE, secretkey);
byte[] utf8 = encryptarea.getText().getBytes("UTF8");
byte[] encr = enc.doFinal(utf8);
String encrypted = new sun.misc.BASE64Encoder().encode(encr);
jTextArea2.setText(encrypted);
} catch (Exception e) {
JOptionPane.showMessageDialog(null, e);
}
Since the algorithm will be chosen by the user now, It first has to be accepted into the program as a string before passed into the encryption.
Algorithm = algorithm.getSelectedItem().toString(); //This collects the algorithm from the JComboBox.
if(Algorithm.equals("Select")){
JOptionPane.showMessageDialog(null, "Please Select Encryption Algorithm");
}
else if(Algorithm.equals("DES")){
JOptionPane.showMessageDialog(null, "This Algorithm Supports Eight(8) Characters Only");
}
else if(Algorithm.equals("AES")){
JOptionPane.showMessageDialog(null, "This Algorithm Supports Sixteen(16) Characters Only");
}
//this then makes the encryption become
try {
byte[] k = key.getText().getBytes();
SecretKeySpec secretkey = new SecretKeySpec(k, Algorithm);
Cipher enc = Cipher.getInstance(Algorithm);
enc.init(Cipher.DECRYPT_MODE, secretkey);
byte[]dec = new sun.misc.BASE64Decoder().decodeBuffer(encryptarea.getText());
byte[] utf8 = enc.doFinal(dec);
jTextArea2.setText(new String (utf8,"UTF8"));
} catch (Exception e) {
JOptionPane.showMessageDialog(null, e);
}
Heres the full code for encryption and decryption
import java.awt.Toolkit;
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import javax.swing.JOptionPane;
/**
*
* @author HORD
*/
public class Easyencrypt extends javax.swing.JFrame {
public Easyencrypt() {
initComponents();
setIcon();
}
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jPanel1 = new javax.swing.JPanel();
jButton1 = new javax.swing.JButton();
jScrollPane2 = new javax.swing.JScrollPane();
jTextArea2 = new javax.swing.JTextArea();
decrypt = new javax.swing.JButton();
jScrollPane1 = new javax.swing.JScrollPane();
encryptarea = new javax.swing.JTextArea();
key = new javax.swing.JTextField();
jLabel1 = new javax.swing.JLabel();
jToolBar1 = new javax.swing.JToolBar();
jButton3 = new javax.swing.JButton();
jButton4 = new javax.swing.JButton();
jLabel2 = new javax.swing.JLabel();
algorithm = new javax.swing.JComboBox<>();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
setTitle("Easy Encrypt 1.0");
jButton1.setIcon(new javax.swing.ImageIcon(getClass().getResource("/icons8_Encrypt_48px.png"))); // NOI18N
jButton1.setText("Encrypt");
jButton1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton1ActionPerformed(evt);
}
});
jTextArea2.setColumns(20);
jTextArea2.setLineWrap(true);
jTextArea2.setRows(5);
jScrollPane2.setViewportView(jTextArea2);
decrypt.setIcon(new javax.swing.ImageIcon(getClass().getResource("/icons8_Data_Encryption_48px.png"))); // NOI18N
decrypt.setText("Decrypt");
decrypt.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
decryptActionPerformed(evt);
}
});
encryptarea.setColumns(20);
encryptarea.setLineWrap(true);
encryptarea.setRows(5);
encryptarea.setMargin(null);
encryptarea.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyTyped(java.awt.event.KeyEvent evt) {
encryptareaKeyTyped(evt);
}
});
jScrollPane1.setViewportView(encryptarea);
key.setToolTipText("Key should begin with '@' followed by a mixture of five letters and two numbers");
jLabel1.setText("Key:");
jToolBar1.setRollover(true);
jButton3.setIcon(new javax.swing.ImageIcon(getClass().getResource("/icons8_Info_20px_1.png"))); // NOI18N
jButton3.setText("Info");
jButton3.setFocusable(false);
jButton3.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton3ActionPerformed(evt);
}
});
jToolBar1.add(jButton3);
jButton4.setIcon(new javax.swing.ImageIcon(getClass().getResource("/icons8_Help_20px.png"))); // NOI18N
jButton4.setText("Help");
jButton4.setFocusable(false);
jButton4.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton4ActionPerformed(evt);
}
});
jToolBar1.add(jButton4);
jLabel2.setText("Algorithm:");
algorithm.setModel(new javax.swing.DefaultComboBoxModel<>(new String[] { "Select", "AES", "DES" }));
algorithm.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
algorithmActionPerformed(evt);
}
});
private void encryptareaKeyTyped(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_encryptareaKeyTyped
String x = encryptarea.getText();
String replace = x.replace(" ", "");
int y = x.length();
// TODO add your handling code here:
}//GEN-LAST:event_encryptareaKeyTyped
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton1ActionPerformed
try {
byte[] k = key.getText().getBytes();
SecretKeySpec secretkey = new SecretKeySpec(k, Algorithm);
Cipher enc = Cipher.getInstance(Algorithm);
enc.init(Cipher.ENCRYPT_MODE, secretkey);
byte[] utf8 = encryptarea.getText().getBytes("UTF8");
byte[] encr = enc.doFinal(utf8);
String encrypted = new sun.misc.BASE64Encoder().encode(encr);
jTextArea2.setText(encrypted);
} catch (Exception e) {
JOptionPane.showMessageDialog(null, e);
}
// TODO add your handling code here:
}//GEN-LAST:event_jButton1ActionPerformed
private void decryptActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_decryptActionPerformed
try {
byte[] k = key.getText().getBytes();
SecretKeySpec secretkey = new SecretKeySpec(k, Algorithm);
Cipher enc = Cipher.getInstance(Algorithm);
enc.init(Cipher.DECRYPT_MODE, secretkey);
byte[]dec = new sun.misc.BASE64Decoder().decodeBuffer(encryptarea.getText());
byte[] utf8 = enc.doFinal(dec);
jTextArea2.setText(new String (utf8,"UTF8"));
} catch (Exception e) {
JOptionPane.showMessageDialog(null, e);
}
// TODO add your handling code here:
}//GEN-LAST:event_decryptActionPerformed
private void jButton3ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton3ActionPerformed
JOptionPane.showMessageDialog(null, "<html><body><p><h1>Easy Encrypt <img src = icons8_Secure_48px.png></img></h1></p>"
+ "<p><h3>Version 1.0.0</h3></p>"
+ "<p>by Official-Hord</p>"
+ "</body></html>");
// TODO add your handling code here:
}//GEN-LAST:event_jButton3ActionPerformed
private void algorithmActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_algorithmActionPerformed
Algorithm = algorithm.getSelectedItem().toString();
if(Algorithm.equals("Select")){
JOptionPane.showMessageDialog(null, "Please Select Encryption Algorithm");
}
else if(Algorithm.equals("DES")){
JOptionPane.showMessageDialog(null, "This Algorithm Supports Eight(8) Characters Only");
}
else if(Algorithm.equals("AES")){
JOptionPane.showMessageDialog(null, "This Algorithm Supports Sixteen(16) Characters Only");
}
// TODO add your handling code here:
}//GEN-LAST:event_algorithmActionPerformed
private void jButton4ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton4ActionPerformed
Help s = new Help();
s.setVisible(true);
// TODO add your handling code here:
}//GEN-LAST:event_jButton4ActionPerformed
private void setIcon() {
setIconImage(Toolkit.getDefaultToolkit().getImage(getClass().getResource("icons8_Secure_48px.png")));
}
public static void main(String args[]) {
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(Easyencrypt.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(Easyencrypt.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(Easyencrypt.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(Easyencrypt.class
.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new Easyencrypt().setVisible(true);
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JComboBox<String> algorithm;
private javax.swing.JButton decrypt;
private javax.swing.JTextArea encryptarea;
private javax.swing.JButton jButton1;
private javax.swing.JButton jButton3;
private javax.swing.JButton jButton4;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JPanel jPanel1;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JScrollPane jScrollPane2;
private javax.swing.JTextArea jTextArea2;
private javax.swing.JToolBar jToolBar1;
private javax.swing.JTextField key;
// End of variables declaration//GEN-END:variables
String Algorithm;
}
The apllication is built with swing java, JFrame and All suggestions are welcome please as to improving the code and application.
Application Guideline (Help)
Instead of taking the long process, I decided to make the job a bit easier for myself by taking a screen shot of the program and adding the instructions with paint, the adding the image to the fram, as an icon to a label. made it way more easier.
setDefaultCloseOperation(javax.swing.WindowConstants.DISPOSE_ON_CLOSE);
setTitle("Easy Encrypt - Help");
jLabel1.setIcon(new javax.swing.ImageIcon(getClass().getResource("/Untitled.jpg"))); // NOI18N
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 561, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 0, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGap(0, 0, Short.MAX_VALUE)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 311, javax.swing.GroupLayout.PREFERRED_SIZE))
);
How to use the application
- First enter the text to be encrypted
- Select Algorithm of choice to be used in the encryption
This message will appear
- Enter key and Click the Encrypt button
Heres a video to explain the whole process again.
Thats all about the new features for now, othere features will be added as need arises, thanks. You can get the source files at [GIT HUB](https://github.com/officialhord/EasyEncrypt).Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
Need help? Write a ticket on https://support.utopian.io.
Chat with us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thanks...
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey @official-hord I am @utopian-io. I have just upvoted you!
Achievements
Utopian Witness!
Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Nice one there @officialhord. How do I get across to you on Discord?
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
official-hord[Ant Man]#3578
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit