What will I learn?
This tutorial covers following topics:
- Multithreading
- Life cycle of threading
- creating threads
Requirements
- A laptop /PC with Mac OS/ Linux/ Wondows
- Preinstalled openjdk
- Preinstalled jvm
- Preinstalled Codeeditor
Difficulty
Intermediate, you must have good knowledge of basics of java programming to catch up this tutorial.
Tutorials Content
In the previous tutorial, we have learned about Multithreading In Python, defined multithreading and implemented in Python.
Now we will discuss multithreading in Java. Multithreading is the occurrence of multiple threads in a program, in which each thread acts as a single program and is different from each other
but uses same memory space, unlike different programs.
Life cycle of a thread
It is necessary to know the life cycle of the thread before we start multithreading in java. In a thread process, the thread may transit into different stages of its life cycle. The different
states of thread life cycle are as follows:
Newborn state: In this state the thread object is created and we are only allowed to do two operations i.e. scheduling the thread to run or kill the thread.
start() is used to schedule it for running and stop() to stop the thread.Runnable state: In this state, the thread will be executed as soon as the processor is available. If there are multiple threads in the queue to be executed than they are executed in First come - First serve approach.
Running state: In this state thread is being executed or processor has given it's time for that particular thread. While the thread is in running state we can be suspended by using
suspend() method and revived again by using resume(), we can make it pause using sleep(time) method which takes time as a compulsory argument and enters again into runnable
state as soon as time finishes.Also, we can make a thread to wait till the certain condition is met using wait() method and is scheduled to work again after the condition is met using notify().Blocked State: In this state thread is either suspended, sleeping or waiting, so it can't enter runnable and running state but it can be run again when certain requirements are met.
Dead state: In this state thread completed it's executing a process or is either killed by using stop().
Creating threads
In Java, threads are created as objects with method run() which gets called by respective thread object and is initiated by start().
We can create new threads by:
- Using a thread class
- Converting class to thread
Using a thread class
We will write a class which extends Thread class and implement the run() method to execute code as thread and call start() to initiate its execution.
Syntax
class ThreadName extends Thread
{
public void run()
{
.............
.............
{
}
Example:
We will look at an example of the thread which calculates area and perimeter of a square. We will create classes AreaSquare to calculate the area of the square and PeriSquare to calculate the
perimeter of square which extends Thread class. We use run() method to create thread objects and is initiated in ThreadTesting class by start() method.
// Creating class which extends Thread class
class AreaSquare extends Thread
{
// run method to create thread
public void run()
{
//defining array of lengths
int[] len = {5,6,7,8,9};
// defining loop to iterate array
for (int i = 0; i< len.length; i++ )
{
//calculating area
int area = len[i] * len[i];
//sleep method to pause execution of thread
try{
sleep(100);
}
catch(Exception e)
{
}
System.out.println("Area is " + area);
}
}
}
class PeriSquare extends Thread
{
public void run()
{
int[] len = {5,6,7,8,9};
for (int i = 0; i< len.length; i++ )
{
int perimeter = 4 * len[i];
try
{
sleep(100);
}
catch(Exception e)
{
}
System.out.println("Perimeter is " + perimeter);
}
}
}
class ThreadTesting
{
// main method
public static void main(String args[])
{
//initializing created thread
new AreaSquare().start();
new PeriSquare().start();
}
}
We have enclosed sleep() method inside try...catch blocks because it throws an exception which needs to be caught inorder to compile the program.
Now we have three threads in total, two threads we created by extending with Thread class and one as main program. All the threads are running independently and they may be executed
whenever processor can have time for them thus, they don't have special sequence of outputs.
AreaSquare as = new AreaSquare();
as.start()
This is equivalent to new AreaSquare().start()
where we created thread object and invoked run() method.
Output:
Perimeter is 24
Area is 49
Perimeter is 28
Area is 64
Perimeter is 32
Area is 81
Perimeter is 36
BUILD SUCCESSFUL (total time: 1 second)
What if we replace start() with run() in ThreadTesting class?
Our ThreadTesting class looks like:
class ThreadTesting
{
public static void main(String args[])
{
new AreaSquare().run();
new PeriSquare().run();
}
}
Output:
Area is 25
Area is 36
Area is 49
Area is 64
Area is 81
Perimeter is 20
Perimeter is 24
Perimeter is 28
Perimeter is 32
Perimeter is 36
BUILD SUCCESSFUL (total time: 1 second)
We can see that while calling run() method directly instead of start() then program executes sequentially and acts like single threaded program, this is because when we call run()
directly it will go into main thread and do not create separate thread for each object.
All above codes can be found on my GitHub link. Click here to download.
What's next?
In coming tutorials we will learn:
- Creating threads coverting class to threads
- Communication between threads
- Thread priority and more.
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey @creon, I just gave you a tip for your hard work on moderation. Upvote this comment to support the utopian moderators and increase your future rewards!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Sneaky Ninja Attack! You have just been defended with a 14.02% upvote!
I was summoned by programminghub. I have done their bidding and now I will vanish…
woosh
P.S. If you or anyone you know has been a victim of @grumpycat please know that he has been harming people throughout Sōsharumedia (ソーシャルメディア). Stealing the service that I (and other bots) have provided them and hiding behind a facade of stopping bid bot abuse which he clearly has no interest in.
Sneaky Ninja is a very responsible bot, working directly with steemcleaners, actively pursuing spam and abuse on our platform. If you would like to see what steps Sneaky Ninja has taken to fight bid bot abuse see this post and this post. Also know that I am working daily on other solutions.
If you would like to know my personal take on bid bot abuse and why I do not agree with the 3.5 day rule, see this post
Grumpycat is a villain that must be stopped to protect our freedoms here on steemit!
There is a resistance that has formed to counter his tyranny.
If you would like to take an active role in stopping this menace and helping other victims like yourself...
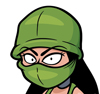
Learn More Here
I have also summoned my love, Kusari to offer some limited help to victims like you.
See Here
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey @programminghub I am @utopian-io. I have just upvoted you!
Achievements
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit