New Features
- What feature(s) did you add?
Encapsulate functionView
- How did you implement it/them?
Given the frequent use of functionView in projects, in order to simplify the amount of code in the project, we simply encapsulated functionView.
This is it.
Before, we put all the buttons in the same xib file.
This will not be able to deal with multiple different scenarios.
So we use each button as a separate class: STMainFunctionItem
func setSubViews(title:String,imageName:String) {
self.setSubViews(title: title, imageName: imageName, target: nil, selector: nil)
}
func setSubViews(title:String,imageName:String,target:Any?,selector:Selector?) {
titleLab.text = title
DesImgV.image = UIImage.init(named: imageName)
self.selector = selector
self.target = target
}
Created by the above two initialization methods.
In the STMainCellFunctionView class, load each item as follows.
Control each item constraint through the snap framework.
func setFunctionItem(items:[STMainFunctionItem]) {
self.items = items
// Add view
for item in items {
self.addSubview(item)
}
// Add constraints
for (idx, item) in items.enumerated() {
item.snp.makeConstraints({ (make) in
// left
if idx == 0{
make.left.equalTo(self.snp.left).offset(0)
}else{
make.left.equalTo(items[idx - 1].snp.right).offset(0)
//width
make.width.equalTo(items[idx - 1].snp.width)
}
// right
if idx == items.count - 1 {
make.right.equalTo(self.snp.right).offset(0)
}else{
make.right.equalTo(items[idx + 1].snp.left).offset(0)
}
// top
make.top.equalTo(self.snp.top).offset(0)
// bottom
make.bottom.equalTo(self.snp.bottom).offset(0)
})
}
}
This revision mainly involves the following documents
//
// STMainCellFunctionView.swift
// SteemThink
//
// Created by zhouzhiwei on 2018/2/26.
// Copyright © 2018年 zijinph. All rights reserved.
//
import UIKit
class STMainFunctionItem: UIView {
lazy var DesImgV: UIImageView = {
let imgV = UIImageView()
return imgV
}()
lazy var titleLab: UILabel = {
let lab = UILabel()
lab.textColor = colorWithHex(hex: 0x707070)
lab.font = kfont(size: 13.0)
return lab
}()
lazy var activity:UIActivityIndicatorView = {
let activity = UIActivityIndicatorView.init(activityIndicatorStyle: UIActivityIndicatorViewStyle.gray)
activity.color = kAppMainColor
activity.hidesWhenStopped = true
activity.isHidden = true
return activity
}()
var selector:Selector?
var target:Any?
override init(frame: CGRect) {
super.init(frame: frame)
self.setUpSubViews()
// 添加单机手势
let tap = UITapGestureRecognizer.init(target: self, action: #selector(itemTap))
self.addGestureRecognizer(tap)
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
override func updateConstraints() {
super.updateConstraints()
// 添加约束
self.titleLab.snp.makeConstraints({ (make) in
make.centerY.equalTo(self.snp.centerY)
make.centerX.equalTo(self.snp.centerX).offset(13)
})
self.DesImgV.snp.makeConstraints({ (make) in
make.width.equalTo(25.0)
make.height.equalTo(25.0)
make.centerY.equalTo(self.snp.centerY)
make.right.equalTo(self.titleLab.snp.left).offset(-5.0)
})
self.activity.snp.makeConstraints { (make) in
make.left.equalTo(self.titleLab.snp.right).offset(10.0)
make.centerY.equalTo(self.snp.centerY)
}
}
func setUpSubViews() {
self.addSubview(self.titleLab)
self.addSubview(self.DesImgV)
self.addSubview(self.activity)
self.updateConstraints()
}
func setSubViews(title:String,imageName:String) {
self.setSubViews(title: title, imageName: imageName, target: nil, selector: nil)
}
func setSubViews(title:String,imageName:String,target:Any?,selector:Selector?) {
titleLab.text = title
DesImgV.image = UIImage.init(named: imageName)
self.selector = selector
self.target = target
}
//MARK: - 菊花动画
func showActivityAnimate(isAnimate:Bool) {
if isAnimate {
self.activity.startAnimating()
}else{
self.activity.stopAnimating()
}
}
//MARK: - 单机手势
@objc func itemTap() {
if self.target != nil && self.selector != nil {
(self.target! as AnyObject).perform(self.selector!, with: nil, afterDelay: 0.0)
}
}
}
class STMainCellFunctionView: UIView {
var items:[STMainFunctionItem]?
func setFunctionItem(items:[STMainFunctionItem]) {
self.items = items
// 添加视图
for item in items {
self.addSubview(item)
}
// 添加约束
for (idx, item) in items.enumerated() {
item.snp.makeConstraints({ (make) in
// left
if idx == 0{
make.left.equalTo(self.snp.left).offset(0)
}else{
make.left.equalTo(items[idx - 1].snp.right).offset(0)
//width
make.width.equalTo(items[idx - 1].snp.width)
}
// right
if idx == items.count - 1 {
make.right.equalTo(self.snp.right).offset(0)
}else{
make.right.equalTo(items[idx + 1].snp.left).offset(0)
}
// top
make.top.equalTo(self.snp.top).offset(0)
// bottom
make.bottom.equalTo(self.snp.bottom).offset(0)
})
}
}
}
//
// STMainTableViewCell.swift
// SteemThink
//
// Created by zhouzhiwei on 2018/2/24.
// Copyright © 2018年 zijinph. All rights reserved.
//
import UIKit
import SDWebImage
class STMainTableViewCell: STBaseTableViewCell {
@IBOutlet weak var userIconImgV: UIImageView!
@IBOutlet weak var nameLab: UILabel!
@IBOutlet weak var timeLab: UILabel!
@IBOutlet weak var titleLab: UILabel!
@IBOutlet weak var contentLab: UILabel!
@IBOutlet weak var functionView: STMainCellFunctionView!
var favor:Bool = false
var indexPath:IndexPath?
var content:STContent?{
didSet{
// 重置点赞
favor = false
// 姓名(Name)
nameLab.text = content?.author
// 日期 (Date)
timeLab.text = NSDate_STExtension.getDateLong(fromDate: (content?.last_update)!)
// 标题 (Title)
titleLab.text = content?.root_title
// 内容 (Content)
contentLab.text = content?.body
// 头像 (通过authorName 获取个人信息列表)(UserIcon)
let url = "https://steemitimages.com/u/"+(content?.author)!+"/avatar"
self.userIconImgV.sd_setImage(with: URL.init(string: url), placeholderImage: UIImage.init(named: "user_icon_placeholder"), completed: nil)
self.setFunctionData(content: content)
}
}
var searchResult:STSearchResult?{
didSet{
// 姓名(Name)
nameLab.text = searchResult?.author
// 日期 (Date)
timeLab.text = NSDate_STExtension.getDateLong(fromDate: (searchResult?.created)!)
// 标题 (Title)
titleLab.text = searchResult?.title
// 内容 (Content)
contentLab.text = searchResult?.summary
// 头像 (通过authorName 获取个人信息列表)(UserIcon)
let url = "https://steemitimages.com/u/"+(searchResult?.author)!+"/avatar"
self.userIconImgV.sd_setImage(with: URL.init(string: url), placeholderImage: UIImage.init(named: "user_icon_placeholder"), completed: nil)
// functionView.content = content
// functionView.delegate = self
}
}
override func awakeFromNib() {
super.awakeFromNib()
self.setUpFunctionView()
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
}
func setUpFunctionView() {
functionView.setFunctionItem(items: [STMainFunctionItem(),STMainFunctionItem(),STMainFunctionItem()])
}
// MARK: - 填充function数据
func setFunctionData(content:STContent?) {
let sbd = content?.pending_payout_value.components(separatedBy: " ").first
let dolerStr = " " + "\(String(describing: sbd!))"
let item1 = functionView.items![0]
item1.setSubViews(title: dolerStr, imageName: "main_doller")
let commentStr = String(format: "%d",(content?.children)!)
let item2 = functionView.items![1]
item2.setSubViews(title: commentStr, imageName: "main_comment")
let voteStr = String(format: "%d",(content?.active_votes_arr?.count)!)
let item3 = functionView.items![2]
item3.showActivityAnimate(isAnimate: (content?.loading)!)
if UserDataManager.sharedInstance.isLogin() {
for vote in (content?.active_votes_arr)! {
if vote.voter == UserDataManager.sharedInstance.getUserName(){
favor = true
break
}
}
}
let imageName = favor ? "main_vote":"main_unvote"
item3.setSubViews(title: voteStr, imageName: imageName, target: self, selector: #selector(voteViewOnTap))
}
//MARK: - Vote
@objc func voteViewOnTap() {
if !self.favor {
content?.loading = true
functionView.items![2].showActivityAnimate(isAnimate: true)
let idx = self.indexPath
STClient.vote(voter: UserDataManager.sharedInstance.getUserName(), author: content!.author, permlink: content!.permlink, weight: 10000, to:nil) { (response, error) in
self.content?.loading = false
self.functionView.items![2].showActivityAnimate(isAnimate: false)
if error == nil && idx == self.indexPath{
self.voteAnimate()
}
}
}
}
//MARK: - Animate
func voteAnimate() {
// 如果已经点赞忽略
if self.favor {
return
}
// 插入点赞的模型(本人点赞)
let mineVote = STContentActiveVotes()
mineVote.voter = UserDataManager.sharedInstance.getUserName()
self.content?.active_votes_arr?.append(mineVote)
// 刷新点赞个数
let item3 = functionView.items![2]
item3.titleLab.text = String(format: "%d",(content?.active_votes_arr?.count)!)
// 点赞动画
UIView.animate(withDuration: 0.3, animations: {
let newTransform = CGAffineTransform.init(scaleX: 1.2, y: 1.2)
item3.DesImgV.transform = newTransform
item3.DesImgV.alpha = 0.5
}) { (finished) in
// if self.favor {
// self.voteImgV.image = UIImage.init(named: "main_unvote")
// }else{
item3.DesImgV.image = UIImage.init(named: "main_vote")
// }
self.favor = !self.favor
UIView.animate(withDuration: 0.3, animations: {
item3.DesImgV.transform = CGAffineTransform.identity
item3.DesImgV.alpha = 1
})
}
}
}
//
// STContentDetailTableViewCell.swift
// SteemThink
//
// Created by zhouzhiwei on 2018/2/28.
// Copyright © 2018年 zijinph. All rights reserved.
//
import UIKit
class STContentDetailTableViewCell: STBaseTableViewCell {
@IBOutlet weak var userIconImgV: UIImageView!
@IBOutlet weak var nameLab: UILabel!
@IBOutlet weak var timeLab: UILabel!
@IBOutlet weak var contentLab: UILabel!
@IBOutlet weak var functionView: STMainCellFunctionView!
var favor:Bool = false
var indexPath:IndexPath?
var comment:STComment?{
didSet{
// 重置点赞
favor = false
// 姓名(Name)
nameLab.text = comment?.author
// 日期 (Date)
// let timeStr = comment?.created.replacingOccurrences(of: "T", with: " ")
timeLab.text = NSDate_STExtension.getDateLong(fromDate: (comment?.created)!)
// 内容 (Content)
contentLab.text = comment?.body
// 头像 (通过authorName 获取个人信息列表)(UserIcon)
let url = "https://steemitimages.com/u/"+(comment?.author)!+"/avatar"
self.userIconImgV.sd_setImage(with: URL.init(string: url), placeholderImage: UIImage.init(named: "user_icon_placeholder"), completed: nil)
// let sbd = comment?.pending_payout_value.components(separatedBy: " ").first
// functionView.dollarLab.text = " " + "\(String(describing: sbd!))"
// functionView.voteLab.text = String(format: "%d",(comment?.active_votes_arr?.count)!)
// functionView.commentLab.text = String(format: "%d",(comment?.children)!)
self.setFunctionData(comment: comment!)
}
}
override func awakeFromNib() {
super.awakeFromNib()
self.setUpFunctionView()
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
}
func setUpFunctionView() {
functionView.setFunctionItem(items: [STMainFunctionItem(),STMainFunctionItem()])
}
// MARK: - 填充function数据
func setFunctionData(comment:STComment?) {
let sbd = comment?.pending_payout_value.components(separatedBy: " ").first
let dolerStr = " " + "\(String(describing: sbd!))"
let item1 = functionView.items![0]
item1.setSubViews(title: dolerStr, imageName: "main_doller")
// let commentStr = String(format: "%d",(content?.children)!)
// let item2 = functionView.items![1]
// item2.setSubViews(title: commentStr, imageName: "main_comment")
let voteStr = String(format: "%d",(comment?.active_votes_arr?.count)!)
let item2 = functionView.items![1]
if UserDataManager.sharedInstance.isLogin() {
for vote in (comment?.active_votes_arr)! {
if vote.voter == UserDataManager.sharedInstance.getUserName(){
favor = true
break
}
}
}
let imageName = favor ? "main_vote":"main_unvote"
item2.setSubViews(title: voteStr, imageName: imageName, target: self, selector: #selector(voteViewOnTap))
}
//MARK: - Vote
@objc func voteViewOnTap() {
if self.favor {
let window = UIApplication.shared.keyWindow
let idx = self.indexPath
STClient.vote(voter: UserDataManager.sharedInstance.getUserName(), author: comment!.author, permlink: comment!.permlink, weight: 10000, to:window) { (response, error) in
if error != nil && idx == self.indexPath{
self.voteAnimate()
}
}
}
}
//MARK: - Animate
func voteAnimate() {
// 如果已经点赞忽略
if self.favor {
return
}
// 插入点赞的模型(本人点赞)
let mineVote = STContentActiveVotes()
mineVote.voter = UserDataManager.sharedInstance.getUserName()
self.comment?.active_votes_arr?.append(mineVote)
// 刷新点赞个数
let item3 = functionView.items![1]
item3.titleLab.text = String(format: "%d",(comment?.active_votes_arr?.count)!)
// 点赞动画
UIView.animate(withDuration: 0.3, animations: {
let newTransform = CGAffineTransform.init(scaleX: 1.2, y: 1.2)
item3.DesImgV.transform = newTransform
item3.DesImgV.alpha = 0.5
}) { (finished) in
// if self.favor {
// self.voteImgV.image = UIImage.init(named: "main_unvote")
// }else{
item3.DesImgV.image = UIImage.init(named: "main_vote")
// }
self.favor = !self.favor
UIView.animate(withDuration: 0.3, animations: {
item3.DesImgV.transform = CGAffineTransform.identity
item3.DesImgV.alpha = 1
})
}
}
}
//
// ViewController.swift
// SteemThink
//
// Created by zhouzhiwei on 2018/2/12.
// Copyright © 2018年 zijinph. All rights reserved.
//
import UIKit
import MJRefresh
enum MainRequestType: Int {
case New = 0
case Hot = 1
case Trending = 2
}
class STMainViewController: UITableViewController{
lazy var dataSource:[STContent] = {
let source:[STContent] = []
return source
}()
var requestType:MainRequestType?
override func viewDidLoad() {
super.viewDidLoad()
self.setUpTableView()
// self.loadData()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
//MARK: - 初始化
class func initWith(requestType:MainRequestType) -> STMainViewController {
let VC = STMainViewController.init()
VC.requestType = requestType
return VC
}
//MARK: - 初始化TableView
func setUpTableView() -> Void {
self.tableView.tableFooterView = UIView.init()
self.tableView.register(UINib.init(nibName:String(describing: type(of:STMainTableViewCell())), bundle: Bundle.main), forCellReuseIdentifier: STMainTableViewCell.cellIdentifier())
self.tableView.estimatedRowHeight = 218
self.tableView.separatorInset = UIEdgeInsetsMake(0, 12, 0, 12)
self.tableView.mj_header = MJDIYHeader.init(refreshingBlock: {
self.loadData()
})
self.tableView.mj_footer = MJRefreshAutoFooter.init(refreshingBlock: {
})
self.tableView.mj_header.beginRefreshing()
}
//MARK: - 获取数据
func loadData() -> Void {
let dict = ["tag":"life", "limit": "50"]
let jsonStr = NSDictionary_STExtension.getJSONStringFromDictionary(dictionary: dict)
// 根据枚举类型选择URL
var urlH:String?
switch self.requestType! {
case .New:
urlH = discussions_by_created;
break
case .Hot:
urlH = discussions_by_hot
break
case .Trending:
urlH = discussions_by_trending
break
}
let url = urlH! + "query=" + jsonStr.addingPercentEncoding(withAllowedCharacters: .urlQueryAllowed)!
STClient.get(url: url, parameters: nil, to: nil) { (response: Any?,error: Error?) in
self.tableView.mj_header.endRefreshing()
self.tableView.mj_footer.endRefreshingWithNoMoreData()
if !(error != nil) {
if response is NSArray{
let arr3:NSArray = response as! NSArray
for dict3:Dictionary in arr3 as! [Dictionary<String, Any>]{
// 遍历得到Model
let trending = STContent.init(dict: dict3)
self.dataSource.append(trending)
}
}
self.tableView.reloadData()
}
}
}
// MARK: - TableViewDelegate & TableViewDataSource
override func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return self.dataSource.count
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell:STMainTableViewCell = tableView.dequeueReusableCell(withIdentifier: STMainTableViewCell.cellIdentifier(), for: indexPath) as! STMainTableViewCell
cell.content = self.dataSource[indexPath.row]
cell.indexPath = indexPath
return cell
}
override func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
tableView.deselectRow(at: indexPath, animated: true)
let content = self.dataSource[indexPath.row]
let VC:STContentDetailViewController = UIStoryboard.init(name: "STBodyStoryboard", bundle: Bundle.main).instantiateViewController(withIdentifier: String(describing: STContentDetailViewController.classForCoder())) as! STContentDetailViewController
VC.content = content
VC.hidesBottomBarWhenPushed = true;
self.navigationController?.pushViewController(VC, animated: true)
}
}
//
// STContentDetailViewController.swift
// SteemThink
//
// Created by zhouzhiwei on 2018/2/28.
// Copyright © 2018年 zijinph. All rights reserved.
//
import UIKit
import IQKeyboardManager
let bootomEdge:CGFloat = 45.0
class STContentDetailViewController: UIViewController,UITableViewDelegate,UITableViewDataSource {
@IBOutlet weak var tableView: UITableView!
var content:STContent?
lazy var dataSource:[STComment] = {
let source:[STComment] = []
return source
}()
override func viewDidLoad() {
super.viewDidLoad()
self.title = localizedString(key: "Content", comment: "")
self.setUpTableView()
self.setUpInputView()
// self.loadData()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
IQKeyboardManager.shared().isEnabled = false
}
override func viewDidDisappear(_ animated: Bool) {
super.viewDidDisappear(animated)
IQKeyboardManager.shared().isEnabled = true
}
func setUpTableView() -> Void {
self.tableView.register(UINib.init(nibName: String(describing: STContentDetailTableViewCell.classForCoder()), bundle: Bundle.main), forCellReuseIdentifier: STContentDetailTableViewCell.cellIdentifier())
let contentHeaderView:STContentHeaderView = Bundle.main.loadNibNamed(String(describing: STContentHeaderView.classForCoder()), owner: self, options: nil)?.first as! STContentHeaderView
contentHeaderView.content = self.content
contentHeaderView.reloadFrame()
self.tableView.tableHeaderView = contentHeaderView
self.tableView.tableFooterView = UIView()
let edge = self.tableView.contentInset
self.tableView.contentInset = UIEdgeInsetsMake(edge.top, edge.left, bootomEdge, edge.right)
self.tableView.mj_header = MJDIYHeader.init(refreshingBlock: {
self.loadData()
})
self.tableView.mj_header.beginRefreshing()
}
func setUpInputView() {
let inputView = STCommentInputView.init(frame: CGRect.init(x: 0, y: screen_height - bootomEdge, width: screen_width, height: bootomEdge)) {[weak self] (text) in
self?.sencComment(text: text)
}
self.view.addSubview(inputView)
}
func setFooterView(isShow:Bool) -> Void {
if isShow {
let contentFooterView:STContentNoDataFooterView = Bundle.main.loadNibNamed(String(describing: STContentNoDataFooterView.classForCoder()), owner: self, options: nil)?.first as! STContentNoDataFooterView
contentFooterView.detailLab.text = localizedString(key: "No Comment", comment: "")
self.tableView.tableFooterView = contentFooterView;
}else{
self.tableView.tableFooterView = UIView()
}
}
func loadData() -> Void {
let url = get_content_replies + "author=" + (content?.author)! + "&permlink=" + (content?.permlink)!
STClient.get(url: url, parameters: nil, to: nil) { (response: Any?,error: Error?) in
self.tableView.mj_header.endRefreshing()
self.dataSource.removeAll()
if response is NSArray{
let dicArr:NSArray = response as! NSArray
if dicArr.count == 0 {
// 显示占位图
self.setFooterView(isShow: true)
return;
}
// 不显示占位图
self.setFooterView(isShow: false)
for dic:Dictionary in dicArr as! [Dictionary<String, Any>]{
// 遍历得到Model
let comment = STComment.init(dict: dic)
self.dataSource.append(comment)
}
}
self.tableView.reloadData()
}
}
// MARK: - TableViewDelegate & TableViewDataSource
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return self.dataSource.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell:STContentDetailTableViewCell = tableView.dequeueReusableCell(withIdentifier: STContentDetailTableViewCell.cellIdentifier(), for: indexPath) as! STContentDetailTableViewCell
cell.indexPath = indexPath
cell.comment = self.dataSource[indexPath.row]
return cell
}
//MARK: - Send Comment
func sencComment(text:String?) {
let permlink = STFormatter.commentPermlink(parentAuthor: (content?.author)!, parentPermlink: (content?.parent_permlink)!)
print("permlink ========" + permlink!)
if permlink != nil {
STClient.comment(parentAuthor: (content?.author)!, parentPermlink: (content?.permlink)!, author: UserDataManager.sharedInstance.getUserName(), permlink: permlink!, body: text!, to:self.view) { (response, error) in
self.tableView.mj_header.beginRefreshing()
}
}
}
}
//
// STSearchViewController.swift
// SteemThink
//
// Created by zhouzhiwei on 2018/2/27.
// Copyright © 2018年 zijinph. All rights reserved.
//
import UIKit
import MJRefresh
class STSearchViewController: UIViewController,UITableViewDelegate,UITableViewDataSource,UISearchBarDelegate {
@IBOutlet weak var tableView: UITableView!
lazy var dataSource:[STSearchResult] = {
let source:[STSearchResult] = []
return source
}()
var searchBar:UISearchBar?
var page:Int = 1
override func viewDidLoad() {
super.viewDidLoad()
self.navigationItem.title = localizedString(key: "Search", comment: "")
self.setUpNavigationBar()
self.setUpTableView()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
// MARK: - UINavigationBarappend
func setUpNavigationBar() -> Void {
self.searchBar = UISearchBar.init(frame: CGRect.zero)
self.searchBar?.placeholder = localizedString(key: "Search", comment: "")
self.searchBar?.delegate = self
self.navigationItem.titleView = searchBar
// 导航栏放置UISearchBar 导航栏高度变高 (iOS 11)
if #available(iOS 11.0, *) {
self.searchBar?.heightAnchor.constraint(equalToConstant: 44.0).isActive = true
}
// 弹出键盘
self.searchBar?.becomeFirstResponder()
}
//MARK: - 初始化TableView
func setUpTableView() -> Void {
self.tableView.tableFooterView = UIView()
self.tableView.register(UINib.init(nibName:String(describing: type(of:STMainTableViewCell())), bundle: Bundle.main), forCellReuseIdentifier: STMainTableViewCell.cellIdentifier())
self.tableView.estimatedRowHeight = 218
self.tableView.separatorInset = UIEdgeInsetsMake(0, 12, 0, 12)
self.tableView.mj_footer = MJDIYAutoFooter.init(refreshingBlock: {
self.page += 1
self.loadData(searchText: self.searchBar!.text!)
})
}
//MARK: - 获取数据
func loadData(searchText:String) {
let url = get_search_result + "+" + searchText + "&pg=" + "\(self.page)"
// url编码
STClient.get(url: url.addingPercentEncoding(withAllowedCharacters: .urlQueryAllowed), parameters: nil, to: nil) { (response: Any?,error: Error?) in
self.tableView.mj_footer.endRefreshing()
if !(error != nil) {
let responseData = response as! Dictionary<String, Any>
// 判断有无更多数据
if responseData["pages"] != nil {
let page = STSeachPages.init(dict: responseData["pages"] as! [String : Any])
if !page.has_next {
self.tableView.mj_footer.endRefreshingWithNoMoreData()
}
}
// 数据装载
if responseData["results"] is NSArray{
let arr:NSArray = responseData["results"] as! NSArray
for dict:Dictionary in arr as! [Dictionary<String, Any>]{
// 遍历得到Model
let searchResult = STSearchResult.init(dict: dict)
self.dataSource.append(searchResult)
}
}
self.tableView.reloadData()
}
}
}
// MARK: - TableViewDelegate & TableViewDataSource
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return self.dataSource.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell:STMainTableViewCell = tableView.dequeueReusableCell(withIdentifier: STMainTableViewCell.cellIdentifier(), for: indexPath) as! STMainTableViewCell
cell.searchResult = self.dataSource[indexPath.row]
cell.indexPath = indexPath
return cell
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
tableView.deselectRow(at: indexPath, animated: true)
// let content = self.dataSource[indexPath.row]
// let VC:STContentDetailViewController = UIStoryboard.init(name: "STBodyStoryboard", bundle: Bundle.main).instantiateViewController(withIdentifier: String(describing: STContentDetailViewController.classForCoder())) as! STContentDetailViewController
// VC.content = content
// VC.hidesBottomBarWhenPushed = true;
// self.navigationController?.pushViewController(VC, animated: true)
}
// func scrollViewDidScroll(_ scrollView: UIScrollView) {
// self.searchBar?.resignFirstResponder()
// }
func scrollViewWillBeginDragging(_ scrollView: UIScrollView) {
self.searchBar?.resignFirstResponder()
}
//MARK: - UISearchBarDelegate
func searchBarSearchButtonClicked(_ searchBar: UISearchBar) {
self.page = 1
self.loadData(searchText: searchBar.text!)
}
}
Posted on Utopian.io - Rewarding Open Source Contributors
Your contribution cannot be approved because it does not follow the Utopian Rules.
Hello @steemthinkcom, thank you for providing me the test link for the app. After much thought, I cannot accept this contribution for the following reasons:
WayneChou1/master
- is this you or another developer?If you think this decision was a mistake, please feel free to contact my supervisor (Amos#4622) in discord.
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey @eastmael, I just gave you a tip for your hard work on moderation. Upvote this comment to support the utopian moderators and increase your future rewards!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Your contribution cannot be approved yet. See the Utopian Rules. Please edit your contribution to reapply for approval.
Can you please send a link on where we can test this?
You may edit your post here, as shown below:
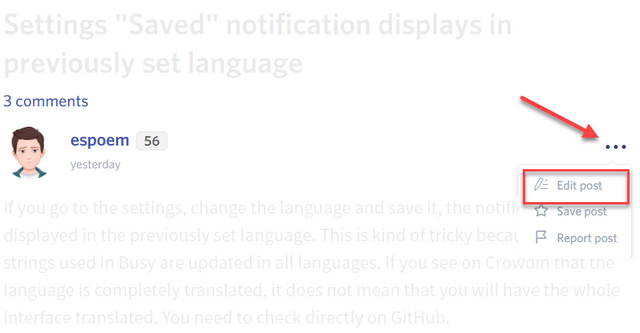
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Can you install ipa? If you can upload IPA to the network, I can install it for testing
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
I have an iPhone. Can't this be tested using TestFlight?
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
yeah~
can you give me you "first name / last name / email"
I will add you as a tester
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
DMed you the details in discord.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hi! I am a robot. I just upvoted you! I found similar content that readers might be interested in:
http://www.seemuapps.com/tutorial-custom-uitableview-cell
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit