Hello Everyone, This is a short Java program using Eclipse IDE. This program uses Multiple Classes to print out a Happy New Year message to the screen. The program has two classes; a mainClass and a secondClass. A simple method with a parameter is used in secondClass to achieve this. The message to be displayed is written in the secondClass and the called in the main class using a “dot” (.) separator. When the program is run, it displays a Happy New Year Pop-Up Message to the screen.
This program illustrates how a user can use multiple classes to write a program, also, using methods to make a program a lot shorter than when all the code is contained in the main class.
Program prompts user to enter year when it is run.
A message is displayed on the screen.
Program ends if user exits prematurely.
GitHub Account
Source Codes from GitHub Account
SECOND CLASS
Line 1: Import Statement
Line 3: Package Name
Line 4: Method. A method with a single parameter is written. The Method will display a Message to the screen when called.
Line 5: Method Closing Brace.
Line 6: Package Closing Brace.
MAIN CLASS
Line 1: Import Statement
Line 3: Package Name
Line 4: Main Method. Every Java program needs a Main Method to run.
Line 5: Exception Handling try Statement.
Line 6: Variable declaration and Initialization. The user enters a value and it is stored as a String.
Line 7: Conversion. A String variable is converted into an Integer and stored as an Integer variable.
Line 8: Object. An object of the secondClass is created. Objects are needed so the user can call Methods contained in the secondClass.
Line 9: The object created is used to call a Method in the secondClass. An Integer variable is passed as argument for the method to be called successfully. The program displays the message on the screen after the Method is called.
Line 10: Exception Handling try Statement Closing Brace.
Line 11: Exception Handling catch Statement.
Line 12: A message is displayed on the screen if the user ends the program prematurely.
Line 13: Exception Handling catch Statement Closing Brace
Line 14: Main Method Closing Brace.
Line 15: Package Closing Brace.
Code Block
SECOND CLASS
import javax.swing.JOptionPane;
public class secondClass {
public void displayMessage(int year) {
JOptionPane.showMessageDialog(null, "Happy New Year " + year);
}
}
MAIN CLASS
import javax.swing.JOptionPane;
public class mainClass {
public static void main (String args []) {
try {
String numberYear = JOptionPane.showInputDialog("Enter Year");
int numberYearInt = Integer.parseInt(numberYear);
secondClass message = new secondClass();
message.displayMessage(numberYearInt);
}
catch (Exception e) {
JOptionPane.showMessageDialog(null, "You have ended the program");
}
}
}
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
happy new year
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Happy New Year to you.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Your contribution cannot be approved yet because it is attached to the wrong repository. Please edit your contribution and fix the repository to
https://github.com/eclipse/che
to reapply for approval.You may edit your post here, as shown below:
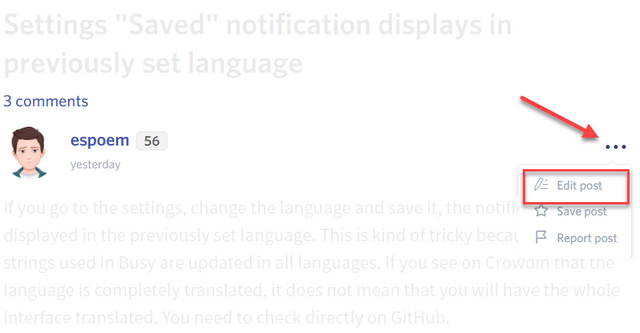
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey @will-ugo I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Congratulations you have selected for big upvote from utopian-io
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit