PROGRAM DESCRIPTION
Hello Everyone, In Java Programming Language, it is possible to generate Random Numbers. Random Numbers can be used for a lot of different things, e.g Writing a program that simulates rolls of a dice. Here is a program that generates a Random Number between a range given by the user and prompts the user to guess the random number generated. If the user guesses the number correctly, the program displays a message to the screen. If the user guesses wrong, the program displays a message telling the user that the user guessed high or low and then displays the random number generated.
A BRIEF SUMMARY OF THE CODES
import java.swing.JOptionPane;
This is an import statement. This statement allows us to use in built functions and methods that are contained in the JOptionPane class.
public class randomNumber {
This is just like creating a new file or document and saving it with a name. This is the name of the new class created.
public static void main (String args []) {
This is the program's Main Method. Every executable program must have a Main Method in order to execute.
String guess
This is a Variable Declaration of String type. String type variable declarations are words e.g "Random", "Wednesday".
int randomNumber
This is a Variable Declaration of int type. int type variable declarations are whole numbers e.g 1,2,3,4
int rangeInt
This is a Variable Declaration of int type. int type variable declarations are whole numbers e.g 1,2,3,4
int guessInt
This is a Variable Declaration of int type. int type variable declarations are whole numbers e.g 1,2,3,4
String range
This is a Variable Declaration of String type. String type variable declarations are words e.g "Random", "Wednesday".
try {
In Java, an Exception occurs when there is a problem during the normal flow of the program. This causes the program to end prematurely.
The process of preventing this from happening is called Exception Handling and it has two statements; try and catch. The try statement list the code in the normal working state while the catch statement states what should occur if a problem is encountered during execution.
range = JOptionPane.showInputDialog()
This is an assignment statement. The String variable declared earlier is given a String value. This value will be stored as range.
if (range == null) {
This is an if Conditional Statement stating a condition.
This simply means "if the value stored in the variable range is null".
JOptionPane.showMessageDialog()
The .showMessageDialog() Method is called from the JOptionPane class. This method allows the user to display a pop up message to the screen.
System.exit(0)
This ends the program. Embedding this line of code in an if Statement shuts down the program if the condition stated in the if statement is true.
If the condition is false, the program proceeds to execute the next line of code which is usually an else Statement
else {
This is an else conditional statement. This statement is executed if the condition in the if statement is false.
guess = JOptionPane.showInputDialog()
The .showInputDialog() Method is called from the JOptionPane class. This enables the user to input a value and it is stored in the variable guess .
Integer.parseInt()
The .parseInt() method is used to convert String type variables to Integer type variables. Here, the String variable range is being converted to an Integer variable rangeInt.
Integer.parseInt()
The .parseInt() method is used to convert String type variables to Integer type variables. Here, the String variable guess is being converted to an Integer variable guessInt.
Math.random()
This in built Method is used to generate Random Numbers in Java. The Random method generates random numbers of double type (meaning decimal numbers).
In order to make it generate integer whole numbers, we need to type cast it. Type Casting it to an Integer will then allow it to generate random integer numbers. We can type cast it by putting int in curly braces before typing the method. e.g: (int) (Math.random()).
The Random Number generated is the stored in the randomNumber variable declared earlier.
if (guessInt == randomNumber) {
This is an if Conditional Statement stating a condition. This simply means "if the value stored in the variable guessInt is equal to the value stored in the variable randomNumber.
JOptionPane.showMessageDialog()
The .showMessageDialog() Method is called from the JOptionPane class. This method allows the user to display a pop up message to the screen.
System.exit(0)
This ends the program. Embedding this line of code in an if Statement shuts down the program if the condition stated in the if statement is true.
If the condition is false, the program proceeds to execute the next line of code.
if (guess == null) {
An if statement inside an if statement is called Nested if statements. This is an if statement with a condition.
JOptionPane.showMessageDialog()
The .showMessageDialog() Method is called from the JOptionPane class. This method allows the user to display a pop up message to the screen.
System.exit(0)
This ends the program. Embedding this line of code in an if Statement shuts down the program if the condition stated in the if statement is true.
If the condition is false, the program proceeds to execute the next line of code.
else if Statement
Another form of Nested if statements is the else if statement. This gives an alternative condition if the condition in the if statement is false. This simply means if the value stored in the variable guessInt is greater than value in randomNumber.
JOptionPane.showMessageDialog()
The .showMessageDialog() Method is called from the JOptionPane class. This method allows the user to display a pop up message to the screen.
else {
This is an else conditional statement. This statement is executed if the condition in the if statement is false.
JOptionPane.showMessageDialog()
The .showMessageDialog() Method is called from the JOptionPane class. This method allows the user to display a pop up message to the screen.
JOptionPane.showMessageDialog()
The .showMessageDialog() Method is called from the JOptionPane class. This method allows the user to display a pop up message to the screen.
catch (Exception e) {
If during the course of executing the program an error is encountered, Exception catch handles the way the program behaves in response to the error.
JOptionPane.showMessageDialog()
The .showMessageDialog() Method is called from the JOptionPane class. This method allows the user to display a pop up message to the screen.
Putting this in an Exception catch statement causes the message to pop up if there is an error encountered.
When run, the program prompts user to enter range for guessing
Program prompts user to enter a guess between 0 and the range
Message prompt to tell the user if the guess was correct or wrong. In the above case, the guess is incorrect.
The random generated number is displayed
Message displayed when the user gets the guess correctly.
GitHub Account
Source Codes from GitHub Account
CODE BLOCK
import javax.swing.JOptionPane;
public class randomNumber {
public static void main (String args []) {
String guess;
int randomNumber;
int rangeInt;
int guessInt;
String range;
try {
range = JOptionPane.showInputDialog("Enter range of Numbers for guess");
if (range == null) {
JOptionPane.showMessageDialog(null, "You quit the program");
System.exit(0);
}
else {
guess = JOptionPane.showInputDialog("Enter a guess between " + 0 + " and " + range);
rangeInt = Integer.parseInt(range);
guessInt = Integer.parseInt(guess);
randomNumber = (int) (Math.random() * rangeInt);
if (guessInt == randomNumber) {
JOptionPane.showMessageDialog(null, "Congratulations, You have guessed correctly!");
System.exit(0);
}
if (guess == null) {
JOptionPane.showMessageDialog(null, "The program has ended!");
System.exit(0);
}
else if (guessInt > randomNumber)
JOptionPane.showMessageDialog(null, "Incorrect, You guessed high!");
else
JOptionPane.showMessageDialog(null, "Incorrect, You guessed low!");
JOptionPane.showMessageDialog(null, "Number generated is " + randomNumber);
}
}
catch (Exception e) {
JOptionPane.showMessageDialog(null, "The program has ended!");
}
}
}
Posted on Utopian.io - Rewarding Open Source Contributors
Nice one
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
i honestly dont understand any of these. can you make it less complex for us none programmers
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Well, you have to know a little bit about programming to understand this, i am a beginner at programming myself. This book helped me a lot, if you wish to learn. https://dcs.abu.edu.ng/staff/aliyu/material/Introduction%20to%20JAVA%20Programming%209th%20Edition%20Daniel%20Liang.pdf
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Your contribution cannot be approved yet because it is not as informative as other contributions. See the Utopian Rules. Please edit your contribution and add try to improve detail of your contribution to reapply for approval.
Presently your contribution does not qualify as a tutorial. So, please organize your information into headings and sub headings.
Include paragraphs of information instead of line by line explanation of the code.
The contribution cannot be accepted in this form. It needs to be thoroughly revised to make it into an informative tutorial.
You may edit your post here, as shown below:
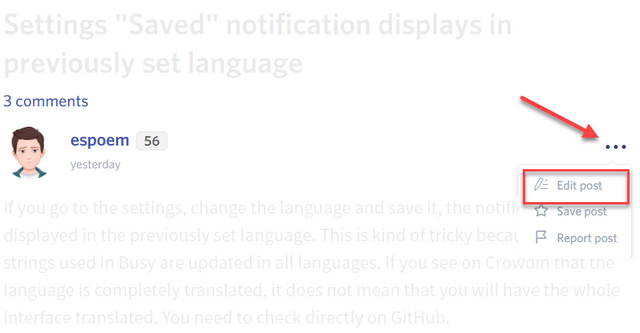
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Edited as indicated
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey @will-ugo I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit