Hello Everyone
I'm AhsanSharif From Pakistan
Greetings you all, hope you all are well and enjoying a happy moment of life with steem. I'm also good Alhamdulillah. |
---|
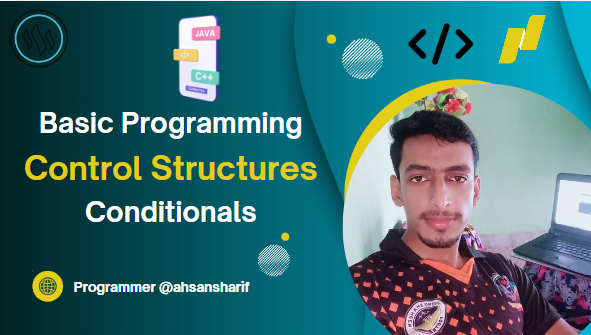
Made In Canva
Conditional structures
Conditional structures are programming constructs that allow code to make basic decisions under any given set of conditions. These tests set the execution flow and check if our condition is true or false. The most commonly found conditional structures include the if
, else if
, and else
statements. These structures allow the program to perform different actions depending on any particular condition. And they also have a special place in the decision-making process in programming.
Working
If Statement:
If the condition is true, it executes the block of code.
Example: if (temperature > 30) {wear sunglasses (); }
Else Statement:
If the if condition is false, it will execute the code block.
Example: else { carry an umbrella(); }
Else If Statement:
It provides another condition so that if the original condition is false, it still wants to test the other condition.
Example: else if (temperature<15) {wear a jacket():}
2 Everyday Situation
01). Making Decisions Based On Weather Conditions:
As the condition is that we have to go out then we have to decide what we have to wear according to our need to go out. For this, some conditional structures are applicable which are as follows.
If the weather is hot (temperature > 30 C)
then we have to decide to wear light clothes accordingly.
If the weather is cold (temperature < 15 C)
, we have to decide to wear thick clothes i.e., coats and jackets.
If it is moderate ( temperature is between 15C and 30C )
, you can wear regular clothes accordingly.
To make this decision in programming, we have to resort to the IF,
ELSE
structure.
if (temperature > 30) {
console.log("Wear light clothing");
} else if (temperature < 15) {
console.log("Wear a jacket");
} else {
console.log("Wear regular clothes");
}
01). Checking Bank Balance Before Making a Purchase:
As when making a purchase we must ensure that we have sufficient funds in our account with which to make the purchase. Here is how we can apply purchasing with conditional structures.
If your account balance is high or full for the purchase, you can proceed with the purchase.
Otherwise, you decide not to make the purchase or choose a cheaper alternative.
This logic in programming is something like this.
if (balance >= purchaseAmount) {
console.log("Proceed with the purchase");
} else {
console.log("Insufficient funds. Choose a cheaper option");
}
Combined Example Of Both Situations
Here is C++ Code
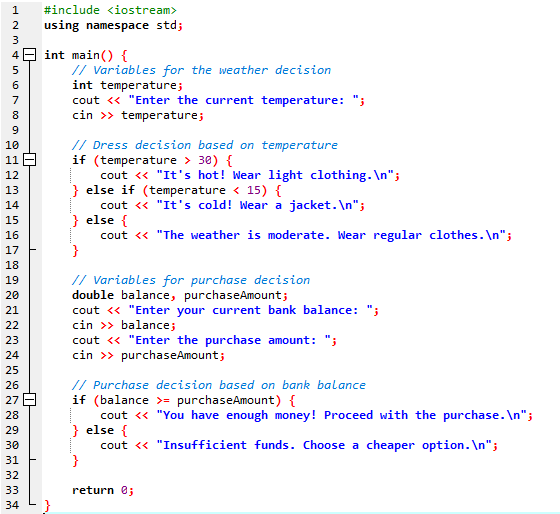
Here is the Output
The output is shown with 30 C temperature and the purchase amount is enough.
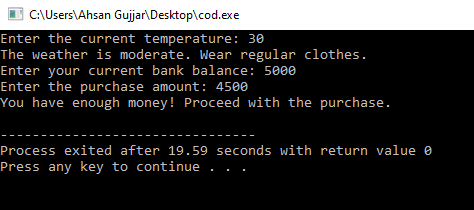
The output is shown with 15 C temperature and the purchase amount is low.
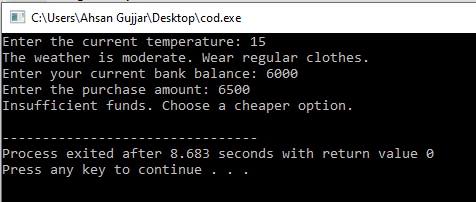

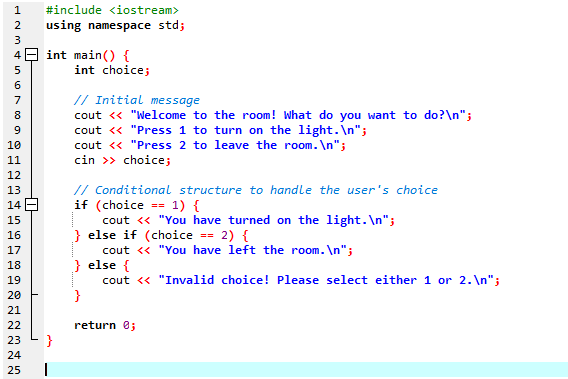
Input:
In this program, the user will be asked to enter one or two of the inputs according to which the action will be performed.
Conditional Statement:
This program will check which option the user has selected by using the structure of if
and if-else
.
If the user has typed one, "You Have Turned On The light" will be printed on the screen in front of him.
If the user has typed two, it will be written in front of it "You Left The Room".
If he types anything other than these two, then the program will inform him that he has made a wrong choice.
It is a simple structure that allows a program to handle user input and respond accordingly. Here we are being shown how conditionals work in a program at design time.
Output after pressing 1 from the user.
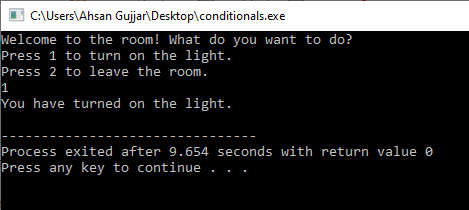
Output after pressing 2 from the user.
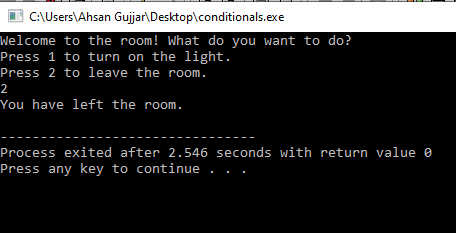

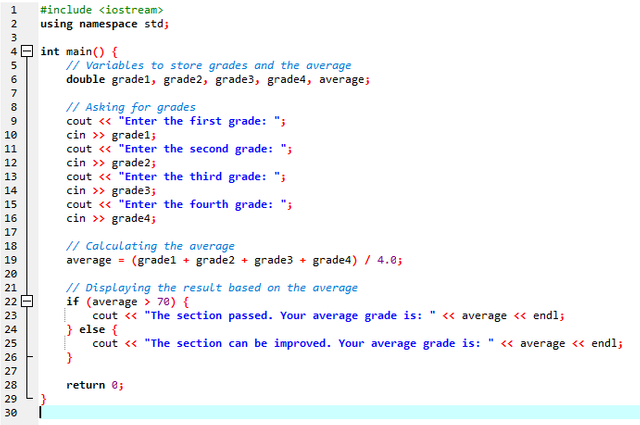
Here I have created a C++ program where the program will receive four inputs from the user, which will be four grades, and calculate the average of these four.
According to its average, it will tell us and display whether it is passed or needs to be improved.
The four grades will be added together to calculate the average. And then they will be divided by four, in which case we will get the average.
Then its conditions will be checked if the average grade is more than 70 then we will get a message displayed "The Section Passed".
If its average falls below 70, then the program will display "The Section Can Be Improved".
Here is the output on a good average.
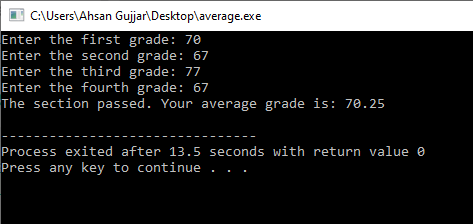
Here is the output on a low average.
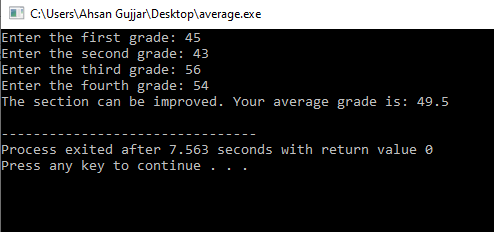

Thanks for visiting my task. I invite my friends @josepha, @kouba01, and @ruthjoe to join this challenge.
Cc:
@alejos7ven
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thank you so much dear @fantvwiki for your kind support and love.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Your post has been rewarded by the Seven Team.
Support partner witnesses
We are the hope!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit