Given a string s, return the length of the longest substring between two equal characters, excluding the two characters. If there is no such substring return -1.A substring is a contiguous sequence of characters within a string.
Example 1:
Input: s = "aa"
Output: 0
Explanation: The optimal substring here is an empty substring between the two 'a's.Example 2:
Input: s = "abca"
Output: 2
Explanation: The optimal substring here is "bc".Example 3:
Input: s = "cbzxy"
Output: -1
Explanation: There are no characters that appear twice in s.Example 4:
Input: s = "cabbac"
Output: 4
Explanation: The optimal substring here is "abba". Other non-optimal substrings include "bb" and "".Constraints:
1 <= s.length <= 300
s contains only lowercase English letters.Hints:
Try saving the first and last position of each character
Try finding every pair of indexes with equal characters
Using Hash Table to Compute the Largest SubString between Two Same Characters in a String
We can use a hash table to store the first seen position of a character. Then, if we see the same character we know the substring length. And sure we can compute the maximum substring.
class Solution { public: int maxLengthBetweenEqualCharacters(string s) { unordered_map<char, int> data; int ans = -1; for (auto i = 0; i < s.size(); ++ i) { if (data.count(s[i])) { ans = max(ans, i - data[s[i]] - 1); } else { data[s[i]] = i; } } return ans; } };
The time complexity is O(N) and the space complexity is also O(N) as we are using a hash set.
--EOF (The Ultimate Computing & Technology Blog) --
Reposted to Algorithms, Blockchain, and Cloud
Follow me for topics of Algorithms, Blockchain and Cloud.
I am @justyy - a Steem Witness
https://steemyy.com
My contributions
- Steem Blockchain Tools
- Computing & Technology
- Download Youtube Video
- Find Cheap & Bargin VPS: VPS Database
- Online Software and Tools
Support me
If you like my work, please:
- Buy Me a Coffee, Thanks!
- Become my Sponsor, Thanks!
- Voting for me:
https://steemit.com/~witnesses type in justyy and click VOTE
Alternatively, you could proxy to me if you are too lazy to vote! and you can also vote me at the tool I made: https://steemyy.com/witness-voting/?witness=justyy
拍拍拍拍拍
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thanks!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
🈵👏🏻!shop 行长牛逼
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thanks!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
good morning
[WhereIn Android] (http://www.wherein.io)
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
拍拍
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
你好鸭,justyy!
@icon123456给您叫了一份外卖!
夏日必备冰淇淋
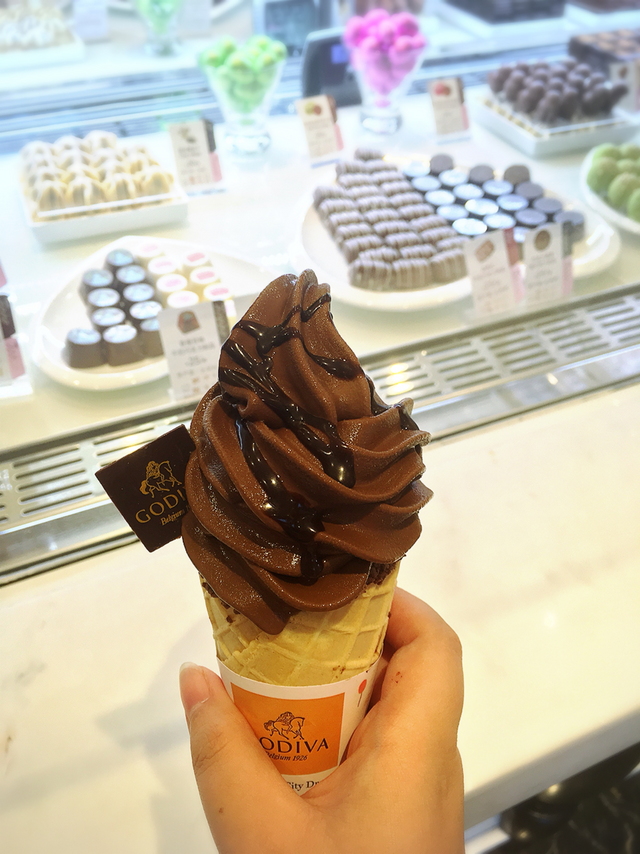
吃饱了吗?跟我猜拳吧! 石头,剪刀,布~
如果您对我的服务满意,请不要吝啬您的点赞~
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit